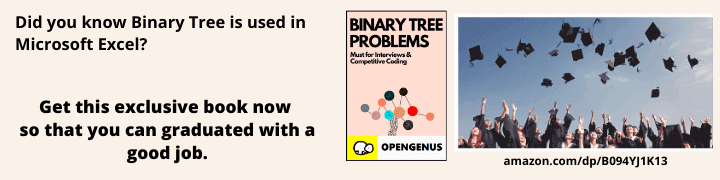
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have developed a script in Python Programming Language to generate secure random passwords.
Table of Contents
- Introduction
- Code
- Complexity
- Applications
- Questions
- Conclusion
Introduction
-
In today’s digital age, password security is more important than ever. With the increasing number of online accounts and sensitive information stored on the internet, it’s crucial to have strong and unique passwords for each account. One way to achieve this is by using a password generator to create random and secure passwords.
-
Python provides several built-in libraries that make it easy to generate random passwords. In this article, we’ll explore how to use these libraries to create a simple password generator script in Python. We’ll also discuss the complexity of the generated passwords and their potential applications.
Code
- First, let’s take a look at a basic password generator script in Python:
import random
import string
def generate_password(length=8):
# Define character sets
lowercase = string.ascii_lowercase
uppercase = string.ascii_uppercase
digits = string.digits
symbols = string.punctuation
# Combine all character sets
all_chars = lowercase + uppercase + digits + symbols
# Generate password
password = ''.join(random.choice(all_chars) for _ in range(length))
return password
# Example usage:
print(generate_password(12))
- Explanation of each part of the code:
1.
import random import string
These two lines import the random and string modules. The random module provides a suite of functions to perform stochastic operations, while the string module provides a set of useful constants and classes for working with strings.
2.
def generate_password(length=8):
This line defines a function called generate_password that takes one optional argument: the desired length of the password. If no value is provided for this argument when calling the function, it will default to 8.
3.
# Define character sets lowercase = string.ascii_lowercase uppercase = string.ascii_uppercase digits = string.digits symbols = string.punctuation
These lines define four variables that contain different sets of characters that can be used in the password. The lowercase variable contains all lowercase ASCII letters, while the uppercase variable contains all uppercase ASCII letters. The digits variable contains all digits (0-9), and the symbols variable contains all punctuation characters.
4.
# Combine all character sets all_chars = lowercase + uppercase + digits + symbols
This line combines all four character sets into a single variable called all_chars. This variable now contains all characters that can be used in the password.
5.
# Generate password password = ''.join(random.choice(all_chars) for _ in range(length))
This line generates the password. It uses a list comprehension to generate a list of length random characters from the all_chars variable. The random.choice() function is used to select a random character from this list on each iteration. The resulting list of characters is then joined into a single string using the .join() method.
6.
return password # Example usage: print(generate_password(12))
These last three lines show an example usage of the generate_password() function. It generates and prints a random password with 12 characters.
-
This script uses the random and string libraries to generate a random password of the specified length (default is 8 characters). The string library provides several pre-defined character sets such as ascii_lowercase, ascii_uppercase, digits, and punctuation. These character sets are combined into one large set (all_chars) which is used by the random.choice() function to select random characters for the password.
-
The code above generates a simple random password using all available characters. However, you can easily modify it to include additional requirements or constraints. For example, you could add a minimum number of uppercase letters or symbols by modifying the script as follows:
def generate_password(length=8):
# Define character sets
lowercase = string.ascii_lowercase
uppercase = string.ascii_uppercase
digits = string.digits
symbols = string.punctuation
# Combine all character sets
all_chars = lowercase + uppercase + digits + symbols
while True:
# Generate password
password = ''.join(random.choice(all_chars) for _ in range(length))
# Check if requirements are met (e.g., at least 2 uppercase letters)
if sum(c.isupper() for c in password) < 2:
continue
return password
This modified version of the script uses a while loop to keep generating passwords until one is found that meets the specified requirements. In this example, the requirement is that the password must contain at least 2 uppercase letters. You can add additional requirements by modifying the if statement inside the loop.
Complexity
-
The complexity of a generated password depends on several factors such as its length and character set. A longer password with a larger character set will generally be more secure than a shorter password with a smaller character set. However, even a long and complex password can be vulnerable if it’s not stored securely or if it’s used for multiple accounts.
-
Increasing the length of a generated password can significantly improve its security. For example, an 8-character password using only lowercase letters has around 200 billion possible combinations. By increasing its length to 12 characters, the number of possible combinations increases to over 95 trillion! This makes it much harder for an attacker to guess or crack your password using brute-force methods.
-
Another factor that can affect the security of a generated password is its character set. A larger character set means more possible combinations and thus greater security. For example, an 8-character password using only lowercase letters has around 200 billion possible combinations. By including uppercase letters, digits, and symbols in its character set, an 8-character password now has over 6 quadrillion possible combinations!
-
It’s important to note that even a long and complex password can be vulnerable if it’s not stored securely or if it’s used for multiple accounts. For example, if you use the same password for multiple accounts and one of those accounts is compromised, all your other accounts using that password are also at risk. It’s therefore crucial to use unique passwords for each account and to store them securely using a password manager.
Applications
-
One potential application of this script is for generating secure passwords for online accounts. By using a unique and randomly generated password for each account, you can reduce the risk of your accounts being compromised due to weak or reused passwords. Another application could be for generating temporary access codes or tokens for secure systems.
-
In addition to these applications, this script could also be used in various other contexts where secure random strings are needed. For example, it could be used to generate random session IDs or CSRF tokens in web applications. It could also be used to generate random encryption keys or initialization vectors (IVs) in cryptographic systems.
Questions
- How does increasing the length of the generated password affect its security?
- What other factors can affect the security of a generated password?
- Can you think of any other potential applications for this script?
Conclusion
In conclusion of this article at OpenGenus, generating random passwords in Python is easy thanks to its built-in libraries. By using these libraries and adding additional requirements or constraints, you can create a powerful password generator script that can help improve your online security.