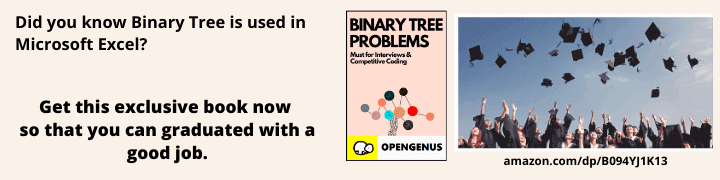
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
GraphQL is defined as a query language for APIs. With the help of graphQL, we can write queries for the data received. It is explained as Front-End focussed API but the fact is that, it is implemented on the server side. GraphQL enables us to describe the data rather than optimizing and implementing it.
A GraphQL server is a server side implementation of GraphQL. It provides the GraphQL data as an API that is needed by frontend applications.
We will implement GraphQL and GraphQL server from a middleware called Apollo Server in Node.JS.
Apollo Server
Apollo server is a JavaScript library that allows the connection between GraphQL schema to an HTTP server. In our application we will connect the GraphQL schema with Express Server. Some of the benefits of using Apollo server is -
- It offers flexibility of Schemas and hence our application.
- It supports community-driven development. Community-Driven development means that it will provide the access of the whole development process, its resources to the group that is involved in the development.
- This server can run and implemented from any GraphQL client.
- Avoids Over-Fetching of Data.
- Avoids Under-Fetching of Data.
- It will only fetch the data that is required.
Target
- Attaching a GraphQL Schema to the HTTP server.
- Adding GraphQL end-points.
Setting Up of Server
To start the server, firstly, we have to initialize our application with package.json file. Therefore, run the following command in the terminal -
npm init
Now, we need to install the dependencies for our application. Therefore, we will install graphql and apollo-server-express and express as our dependencies. To install run the following command -
npm install express graphql apollo-server-express --save
And, we also need to install apollo-server for defining the schema and passing the information to the server. So, run the following command -
npm install apollo-server --save
After installing the dependencies, the package.json file will look like this -
{
"name": "GraphQL_Server",
"version": "1.0.0",
"description": "",
"main": "main.js",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1"
},
"keywords": [],
"author": "",
"license": "ISC",
"dependencies": {
"apollo-server-express": "^2.13.1",
"express": "^4.17.1",
"graphql": "^15.0.0",
"apollo-server": "^2.13.1"
}
}
Now, create the main.js file to keep all the code.
Defining the Schema
A server needs a schema to be defined. Schema defines the structure of the data. For example, lets define a schema in main.js -
const { ApolloServer, gql } = require('apollo-server');
const typeDefs = gql;
const articles = [
{
title: 'opengenus',
author: 'opengenus iq',
},
{
title: 'intern',
author: 'opengenus iq',
},
];
In Schema, we define objects alongwith their types. Therefore, here, we used typeDefs. Also, we defined an array of articles that is called the data set containing titles and authors as objects.
There is another important type called Query type. It lists all the executable queries, alongwith the return type. For example, the structure for query looks like this -
type Query {
articles: [articles]
}
Here, The Object type Query returns the array of articles.
Resolver
Resolver is a callback function that is provided by the GraphQL server. This function is responsible for populating the data for a single field in the schema. It defines the techniques for fetching the types that are defined in the Schema. For example, write the following code in main.js file:
const resolvers = {
Query: {
articles: () => articles,
}
};
Here, our Resolver is set in such a way that it retrieves each article from the articles array we defined earlier.
Instance of ApolloServer
After defining the structure, data set and resolver, we need to create an instance of ApolloServer so that these information can be passed to the server. For creating an instance, we need the new keyword. Therefore,
const server = new ApolloServer({ typeDefs, resolvers })
Here, the instance requires two parameters. First is the Schema definition and second will be the resolvers.
Setting Up of servers
Here comes the final part of our application. In this part, we will setup the listen method, so that the application can be deployed to the local server. Therefore, write the following code in main.js file:
server.listen(3000)
.then(({ url }) => {
console.log(`Server started at ${url}`);
});
Now, start the server with the following command -
node main.js
You will see the following output in the terminal:
Server started at http://localhost:3000/
Therefore, our whole code will be -
const { ApolloServer, gql } = require('apollo-server');
const typeDefs = gql;
const articles = [
{
title: 'opengenus',
author: 'opengenus iq',
},
{
title: 'intern',
author: 'opengenus iq',
},
];
const resolvers = {
Query: {
articles: () => articles,
}
};
const server = new ApolloServer({ typeDefs, resolvers });
server.listen(3000).then(({ url }) => {
console.log(`Server ready at ${url}`);
});
Adding End-Points
For adding endpoints we need to install an extra dependency called body-parser so that it can parse the request coming from the client side. Therefore, write the following command in the terminal -
npm install body-parser --save
To define end-points in express, write the following code in your file -
const express = require('express');
const bodyParser = require('body-parser');
const { graphqlExpress } = require('apollo-server-express')
var app = express();
app.use('/graphql', bodyParser.json(), graphqlExpress({
schema: mySchema
}));
app.listen(3000, (err) => {
if (err) { console.log(err) }
else { console.log('Server Started') }
})
Here, we are setting up the route(end-point) as /graphql. It takes schema defined as an object. In the last line, we have setup the port no. and our server is ready to be deployed on the localhost.
Therefore, the output for the above code will be -
Server Started
You can use any GraphQL client to use the application and setup the schema. Here, I will use this as my graphQL client.
Now, after running the application, just open the above given link and change the endpoint to the url in which you are running your application. Here, the endpoint will be - http://localhost:3000/graphql. Now provide the structure of your Schema as -
query {
articles {
title
author
}
}
Now, hit the play button. You will see the output as following -
{
"data": {
"articles": [
{
"title": "opengenus",
"author": "opengenus iq"
},
{
"title": "intern",
"author": "opengenus iq"
}
]
}
}
With this article at OpenGenus, you must have the complete idea of GraphQL Server with Node.JS. Enjoy.