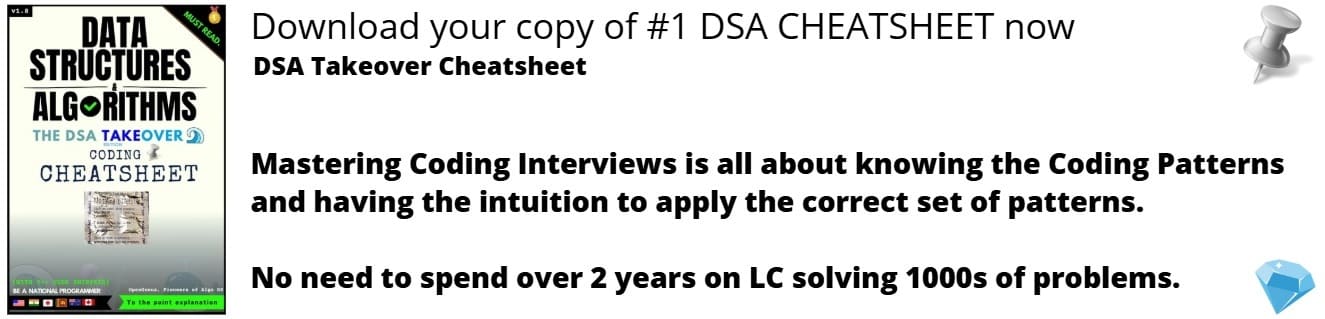
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explained how to take string input in C Programming Language using C code examples. We have explained different cases like taking one word, multiple words, entire line and multiple lines using different functions.
Table of contents:
- String input using scanf Function
1.1. Reading One Word
1.2. Reading Multiple Words
1.3. Reading Multiple Words to Form a Line
1.4. Reading an entire line - Using getchar
- Reading Entire Line using gets()
- Read One Line at a Time from a File using fgets()
- Reading Multiple Lines
Let us learn these techniques using C code examples.
String input using scanf Function
The input function scanf can be used with %s format specification to read in a string of characters.
Reading One Word
For Example :
char instr[10];
scanf("%s",instr);
The problem with the scanf function is that it terminates its input on the first white space it finds. A white space includes blanks,tabs,carraige returns,form feeds and new lines.
Therefore, if the following line of text is typed :
HELLO BOY
then only the string "HELLO" will be read into the array address , since the blank space after the word 'NEW' will terminate the reading of string.
The unused locations are filled with garbage.
The scanf function automatically terminates the string that is read with a null character and therefore, the character array should be large enough to hold the input string plus the null character. Note that unlike previous scanf calls, in the case of character arrays, the ampersand (&) is not required before the variable name.
Reading Multiple Words
If we want to read the entire line "HELLO BOY", then we may use two character arrays of appropriate sizes.
char instr1[10], instr2[10];
scanf("%s %s", instr1,instr2);
It will assign the string "HELLO" to instr1 and "BOY" to instr2.
#include<stdio.h>
#include<string.h>
int main()
{
char instr[100];
printf("Enter a string\n");
scanf("%s",bin);
printf("String is : \n");
puts(bin);
return 0;
}
If we give "WELCOME TO OPENGENUS" as input it will only read WELCOME and displays it as output.
To overcome this problem we can make different arrays for all the words present in the string .
Reading Multiple Words to Form a Line
#include<stdio.h>
#include<string.h>
int main()
{
char instr1[100],instr2[100],instr3[100],instr4[100];
printf("Enter the string of four words\n");
scanf("%s",instr1);
scanf("%s",instr2);
scanf("%s",instr3);
scanf("%s",instr4);
printf("String is : \n");
printf("%s %s %s %s" ,instr1,instr2,instr3,instr4 );
return 0;
}
It will take four words in four different character arrays and displays on the output screen.
Reading an entire line
We have seen that scanf with %s or %ws can read only strings without whitespaces.
However, C supports a format specification known as the edit set conversion code %[..] that can be used to read a line containing a variety of characters, including whitespaces.
char instr[100];
scanf("%[^\n]",instr);
A similar method to above:
char instr[100];
scanf("%[ ABCDEFGHIJKLMNOPQRSTUVWXYZ]", instr);
Using getchar
We can use it repeatedly to read successive single characters from the input and place them into a character array. Thus, an entire line of text can be read and stored in an array. The reading is terminated when the new line character ('\n')
is entered and the null character is then inserted at the end of the string.
#include<stdio.h>
#include<string.h>
main()
{
char instr[100] , ch;
int c = 0;
printf("Enter the line \n");
do
{
ch = getchar();
instr[c]=ch;
c++;
}while(ch != '\n');
c=c-1;
instr[c]='\0';
printf("%s \n", instr);
}
Reading Entire Line using gets()
Another method of reading a string of text containing whitespaces is to use the library function gets available in the <stdio.h> header file.
It reads characters from the keyboard until a new line character is encountered and then appends a null character to the string.
Unlike scanf, it does not skip whitespaces.
For example :
char instr[100];
gets (line);
printf("%s", instr);
It will reads a line from the keyboard and displays it on the screen.
The last program can be also written as :
char instr[100];
printf("%s" , gets(line));
Please note that C does not check for array bounds , so be careful not to input more character that can be stored in the string variable used.
A complete program to demonstrate working of gets :
#include<stdio.h>
#include<string.h>
int main()
{
char instr[100];
printf("Enter a string\n");
scanf("%[^\n]",instr);
printf("The output is:-\n");
puts(bin);
return 0;
}
This program will work perfectly fine and it will take the entire string as input from the keyboard and displays it on the output screen.
Read One Line at a Time from a File using fgets()
The function fgets() takes three parameters that is :
- string_name
- string_max_size
- stdin
We can write it as :
fgets(string_name,string_max_size,stdin);
For Example :
#include<stdio.h>
#include<string.h>
int main()
{
char instr[100];
printf("Enter the string\n");
fgets(instr,100,stdin);
printf("The string is:-\n");
puts(bin);
return 0;
}
It will take the entire string as input from the keyboard and displays the entire screen on the output screen.
Reading Multiple Lines
The following program reads multiple line from the keyboard.
#include<stdio.h>
int main()
{
int s[100];
printf("Enter multiple line strings\n");
scanf("%[^\r]s",s);
printf("Entered String is\n");
printf("%s\n",s);
return 0;
}
Question
Which of the following is the correct input statement for reading an entire string from keyboard ?
All these methods are discussed in the article that is gets() , scanf("%[^\n]",str); and fgets()
Question
A character array instr[] stores the "HELLO". What will be the length of array instr[] requires to store the string completely ?
As the word hello is 5 characters long and there will be a null character at end , we require an array of size 6.
Question
State True or False : "The input function gets has one string parameter ."
The input function gets has one string parameter which reads characters from the keyboard until a new line character is encountered and then appends a null character to the string.
So, with this article at OpenGenus, we have seen all the possible methods to take input string in C programming language.