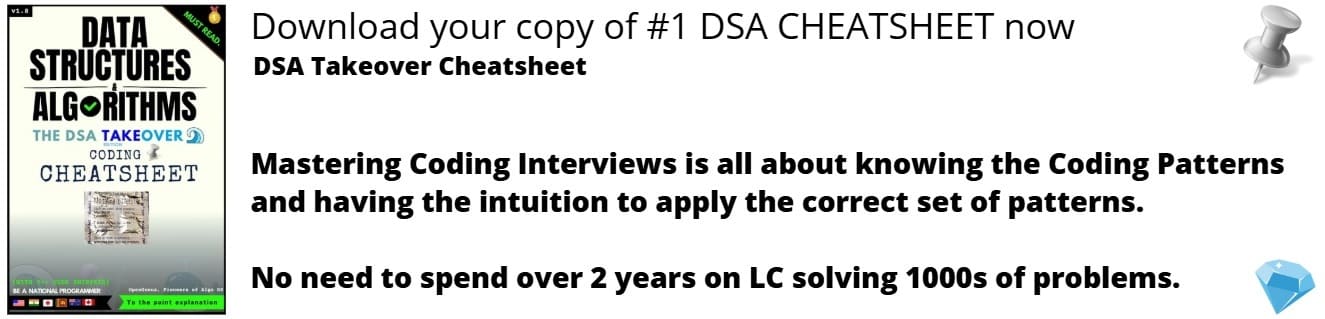
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explored 6 different ways to initialize Multiset in C++ STL along with C++ code snippets for each approach.
Table of contents:
- Introduction to Multiset in C++
- Initializing by inserting values one by one
- Initializing like arrays
- Initializing from an array
- Initializing from a vector
- Initializing from another multiset
- Initializing in descending order
Introduction to Multiset in C++
Multiset is a data structure that is used to store values. In C++'s Standard Template library (STL), we have multiset as an Associative container. Associative containers are those containers which is used to store the sorted data and are much faster in searching the data than other containers.
Multiset is present in "set header" file. It's internal implementation is done using Self Balanced Binary Search Tree. The main difference between a set and multiset is that a set can store only unique values but a multiset can store duplicate values.
In a multiset, the elements are present in the sorted order. The random access of a multiset is not possible. It means that we can’t access an element in O(1) time complexity. The time complexity in searching for an element in a Multiset is O(logn). Once insertion of elements is done in multiset then, we can not modify the value of the element. We can insert more elements to it and even delete the elements from the multiset but modification of elements is not possible.
Syntax:
multiset <datatype> multisetname
Multiset can take data type according to the value ,i.e, int, string, float, double etc.
Different ways to initialize a Multiset in C++ STL:
- Initializing by inserting values one by one
- Initializing like arrays
- Initializing from an array
- Initializing from a vector
- Initializing from another multiset
- Initializing in descending order
1. Initializing by inserting values one by one
All the elements in a multiset can be inserted one-by-one using the class method 'insert'. First, declare the multiset of data type of the value (which we going to store) and then insert the values.
Steps:
- Including the iostream header file in our code, this will allow us to read from the console and write to the console.
- Including the set header file, it defines the set and multiset container classes.
- Including the std namespace,so that we can use the member of std, without calling it again and again.
- Call the main() function inside which the logic of the program is going to be written.
- Start of the body of the main() function.
- Declaring a multiset named as mymultiset.
- Initializing the multiset using insert() method like mymultiset.insert(1). All the elements that is needed to be in multiset should be inserted one-by-one.
- Beginning of the body of the for loop to traverse the mymultiset.
- Using the loop variable "it" to iterate the values of the mymultiset and print them on the console.
- End of the body of the for loop.
- End of the body of main() function.
#include<iostream>
#include<set>
using namespace std;
int main()
{
multiset<int>mymultiset;
mymultiset.insert(1);
mymultiset.insert(2);
mymultiset.insert(3);
mymultiset.insert(4);
mymultiset.insert(5);
for(auto it=mymultiset.begin(); it != mymultiset.end(); it++)
cout << ' ' << *it;
return 0;
}
Output:
1 2 3 4 5
2. Initializing like arrays
We can initialize a multiset similar to the array initialization. We have to give the values in the multiset while declaring it.
Steps:
- Including the iostream header file in our code, this will allow us to read from the console and write to the console.
- Including the set header file, it defines the set and multiset container classes.
- Including the std namespace, so that we can use the member of std, without calling it again and again.
- Call the main() function inside which the logic of the program is going to be written.
- Start of the body of the main() function.
- Declaring a multiset named as multisetname.
- Initializing the multiset by writing values within curly braces{ } like mymultiset{1,2}.
- Beginning of the body of the for loop to traverse the multiset.
- Using the loop variable "it" to iterate the values of the multiset mymultiset and print them on the console.
- End of the body of the for loop.
- The main() function should return an integer value if the program runs fine.
- End of the body of main() function.
#include<iostream>
#include<set>
using namespace std;
int main()
{
multiset<int>mymultiset{ 1, 2, 3, 4, 5 };
for(auto it=mymultiset.begin(); it != mymultiset.end(); it++)
cout << ' ' << *it;
return 0;
}
Output:
1 2 3 4 5
3. Initializing from an array
We can pass an array to the multiset. The Array contains the elements which is to be filled in the multiset and then add the content of the Array to the multiset using range constructor.
Steps:
- Including the iostream header file in our code, this will allow us to read from the console and write to the console.
- Including the set header file, it defines the set and multiset container classes.
- Including the std namespace, so that we can use the member of std, without calling it again and again.
- Call the main() function inside which the logic of the program is going to be written.
- Start of the body of the main() function.
- Declaring an array named arr to store 5 integers (which is to be filled in multiset) and also initialized the five integers.
- Create an integer "n" to store the size of the array.
- Declaring a multiset named as mymultiset.
- Initializing the multiset by passing the array "arr" to the multiset like mymultiset(arr,arr+n).
- Beginning of the body of the for loop to traverse the multiset.
- Using the loop auto variable "it" to iterate the elements of the multiset mymultiset and print them on the console.
- For accessing the elements, use "*it" as iterators are pointers and are pointing to the elements in the mymutiset.
- End of the body of the for loop.
- End of the body of main() function.
#include <iostream>
#include <set>
using namespace std;
int main()
{
int arr[5] = { 1, 2, 3, 4, 5 };
int n = sizeof(arr) / sizeof(arr[0]);
multiset<int> mymultiset(arr, arr + n);
for(auto it=mymultiset.begin(); it != mymultiset.end(); it++)
cout << ' ' << *it;
return 0;
}
Output:
1 2 3 4 5
4. Initializing from a vector
Here, first we initialize a vector with values which we going to store in the multiset. Then, we have to pass the begin() and end() iterators of the initialized vector to the multiset class constructor.
Steps:
- Including the iostream header file in our code, this will allow us to read from the console and write to the console.
- Including the vector header file, it defines the vector container classes.
- Including the set header file, it defines the set and multiset container classes.
- Including the std namespace, so that we can use the member of std, without calling it again.
- Call the main() function inside which the logic of the program is going to be written.
- Start of the body of the main() function.
- Declaring a vector named vect to store 5 integers (which is to be filled in multiset) and also initialized the five integers.
- Declaring a multiset named as mymultiset.
- Initializing the multiset by passing the begin() and end() iterators of the vector "vect" to the multiset like mymultiset(vect.begin(),vect.end()).
- Beginning of the body of the for loop to traverse the multiset.
- Using the loop auto variable "it" to iterate the elements of the mymultiset and print them on the console.
- For accessing the elements, use "*it" as iterators are pointers and are pointing to the elements in the mymutiset.
- End of the body of the for loop.
- End of the body of main() function.
Example 1:
#include <iostream>
#include <vector>
#include <set>
using namespace std;
int main()
{
vector<int> vect{ 1, 2, 3, 4, 5 };
multiset<int> mymultiset(vect.begin(), vect.end());
for(auto it=mymultiset.begin(); it != mymultiset.end(); it++)
cout << ' ' << *it;
return 0;
}
Output:
1 2 3 4 5
Example 2:
#include <iostream>
#include <vector>
#include <set>
using namespace std;
int main()
{
vector<int>vec;
vec.push_back(1);
vec.push_back(8);
vec.push_back(10);
vec.push_back(3);
vec.push_back(6);
multiset<int> mymultiset(vec.begin(), vec.end());
for(auto it=mymultiset.begin(); it != mymultiset.end(); it++)
cout << ' ' << *it;
return 0;
}
Output:
1 3 6 8 10
5. Initializing from another multiset
1st method: Using basic functions like mutiset.begin() and multiset.end()
Here, we can copy values from existing multiset and pass it to the new multiset class contructor by using begin() and end() iterators of the existing multiset.
Steps:
- Including the iostream header file in our code, this will allow us to read from the console and write to the console.
- Including the set header file, it defines the set and multiset container classes.
- Including the std namespace, so that we can use the member of std, without calling it again.
- Call the main() function inside which the logic of the program is going to be written.
- Start of the body of the main() function.
- Declaring a multiset name as mymultiset1 and insert values to it by using insert() method.
- Declare another multiset named as mymultiset2.
- Initializing the mymutiset2 by using begin() and end() iterators of the mymultiset1 like mymultiset2(mymultiset1.begin(), mymultiset1.end()).
- Beginning of the body of the for loop to traverse the mymultiset2.
- Using the loop variable "it" to iterate the values of the mymultiset2 and print them on the console.
- End of the body of the for loop.
- End of the body of main() function.
#include <iostream>
#include <set>
using namespace std;
int main()
{
multiset<int> mymultiset1{ 1, 2, 3, 4, 5 };
multiset<int> mymultiset2(mymultiset1.begin(), mymultiset1.end());
for(auto it=mymultiset2.begin(); it != mymultiset2.end(); it++)
cout << ' ' << *it;
return 0;
}
Output:
1 2 3 4 5
2nd method: Using assignment operator
Here, we are simply initializing a multiset by inserting values one by one and then copying its values to another multiset class using assignment operator (=).
Steps:
- Including the iostream header file in our code, this will allow us to read from the console and write to the console.
- Including the set header file, it defines the set and multiset container classes.
- Including the std namespace, so that we can use the member of std, without calling it again.
- Call the main() function, inside which the logic of the program is going to be written.
- Start of the body of the main() function.
- Declaring a multiset named as mymultiset1 and initialize values one by one by insert() method.
- Declaring another multiset named as mymultiset2.
- Using an assignment operator(=), we copy the the values of mymultiset1 to the mymultiset2.
- Beginning of the body of the for loop to traverse the mymultiset2.
- Using the loop variable "it" to iterate the values of the mymultiset2 and print them on the console.
- End of the body of main() function.
#include <iostream>
#include <set>
using namespace std;
int main()
{
multiset<int> mymultiset1;
mymultiset.insert(1);
mymultiset.insert(2);
mymultiset.insert(3);
mymultiset.insert(4);
mymultiset.insert(5);
multiset<int> mymultiset2;
mymultiset2 = mymultiset1;
for(auto it=mymultiset2.begin(); it != mymultiset2.end(); it++)
cout << ' ' << *it;
return 0;
}
Output:
1 2 3 4 5
6. Initializing in descending order
We can simply initialize values in the multiset in descending order.
Steps:
- Including the iostream header file in our code, this will allow us to read from the console and write to the console.
- Including the set header file, it defines the set and multiset container classes.
- Including the std namespace, so that we can use the member of std, without calling it again.
- Call the main() function inside which the logic of the program is going to be written.
- Start of the body of the main() function.
- Declaring a multiset named as mymultiset and adding "greater int" in the data type.
- Initializing the multiset using insert() method like mymultiset.insert(1).
- Beginning of the body of the for loop to traverse the mymultiset.
- Using the loop variable "it" to iterate the values of the mymultiset and print them on the console.
- End of the body of main() function.
#include<iostream>
#include<set>
using namespace std;
int main()
{
multiset< int, greater<int> > mymultiset;
mymultiset.insert(8);
mymultiset.insert(1);
mymultiset.insert(3);
mymultiset.insert(2);
mymultiset.insert(5);
for(auto it=mymultiset.begin(); it != mymultiset.end(); it++)
cout << ' ' << *it;
return 0;
}
Output:
8 5 3 2 1
With this article at OpenGenus, you must have the complete idea of different ways to initialize a Multiset in C++.