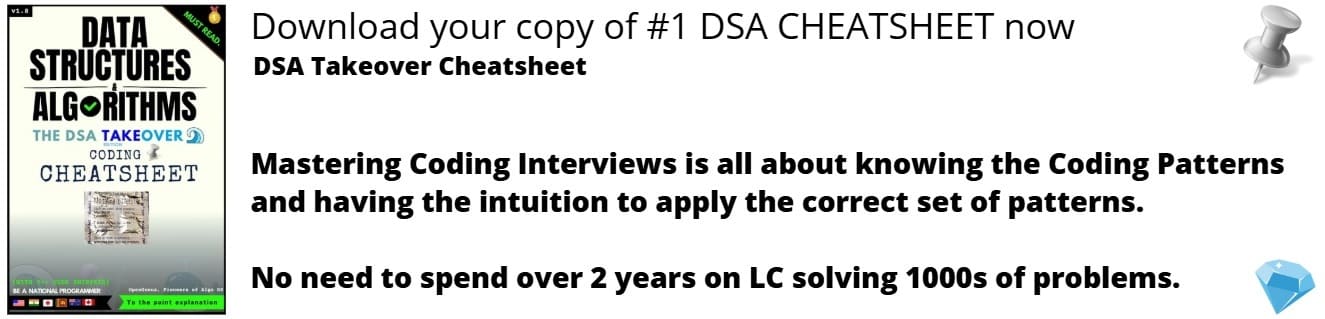
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explored the differences between List, Tuple and Dictionary in Python.
TABLE CONTENTS |
---|
Introduction |
what is list,tuple,dictionary? |
Creation |
Accessing the elements |
Mutability |
Adding an element |
Removing an element |
some methods |
INTRODUCTION
Hola everyone! Most of us know to do code using list, tuple and dictionaries in python separately without any confusions, but when we came to work with all the three together we would get confused, so to avoid those confusions this article helps you in a clear understanding of list, tuple,dictionary. Lets get started!!!
What is list,tuple,dictionary?
lists ,tuple, dictionaries are the built in data types in python used to store the collections of data. Ahh whats new in that? arrays can do this well right? why do we need list,tuple and dictionary then?
arrays can store only the homogenous data type whereas list ,tuple, dictionaries can store multiple data types in a single variable.we will see this visually futher in this article.
Creation
Hey peeps,now we know what is list,tuple and dictionary. then what's next? yeah, you guessed it correct!! we are going to create them now.
Lists are created using [ ] square brackets,a list can different data types.
list1=["carrot","spinach","Avacado","Millet']
list2=[1,6,7,9]
list3=[True,False,True,True]
list4=["apple", 1, 98,True]
Now you can able to see the advantage of using list instead of array which i have mentioned in introduction.
Tuples are created as same as the list ,but we use ( ) instead of square brackets.
tuple1=("Maths","Science","Social")
tuple2=(1,2,3,5)
tuple3=(True,"Saffron",9.8,1)
tuple4=(True,False,True)
Dictionaries are created by placing the sequence of elements inside the curly braces {}, in addition it contains the pair of values, key:Value.
values can be duplicated and can be of any data type but the keys cannot be repeated.
dict = {
"Name" : "Anusha"
"Reg No" : 51
"School" : "VCHSS"
}
Accessing the elements
okay we created the lists, tuple and dictionary ,now how to access the elements in them? can we access the elements with the same method for all three ? lets see.
In List and Tuple we can access the elements by using index,whereas in dictionary we can access the elements by reffering key values. may be it would be vague without seeing an example,let we jump into an example.
>>list1=["Shalini",22,"Shabby",True]
>>print(list1[0])
Shalini
>>tuple1=("Apple","Banana","cherry")
>>print(tuple1[1])
Banana
>>dict1={"Name":"Subashini","Age":22,"Mark":99}
>>print(dict1["Mark"])
99
You would have got a clearance about how to access elements in list vs tuple vs dictionary.
Mutability
An object is mutable if the value of the object can be changed else it is immutable
Lets see whether list,tuple dictionary are mutable or immutable
List and dictionaries are mutable whereas Tuples are immutable
>>List
vegetables=["onion","strawberry","drumstick","beans"]
vegetables["strawberry"]="brinjal"
>>dictionary
Marks={"surya":99,"vijay":98,"Ajith":96}
Marks["Ajith"]=97
if we try to change element in tuple it will give an error:(
Adding an element
we can add a element in list by using append(),insert()
>>list1=["Apple","Orange","Mango"]
>>list1.append("Guava")
['Apple','Orange','Mango','Guava']
>>list1.insert(2,"Guava")
['Apple','Orange','Guava','Mango']
Unlike list we cannot add a element in dictionary using append(),insert() we can add a element in the dictionary by defining a value along with key eg: dict1[key]='value'
>>dict1={"Chocolate":"Dairy Milk","Brand":"cadbourys"}
>>dict1["Rupees"]=100
{'Chocolate':'Dairy Milk','Brand':'cadbourys','Rupees':100}
Unlike list and dictionary we cannot add a element in tuple as it is immutable.
Removing an element
We learned how to add elements in list vs dictionary vs tuple , now lets see how to remove an element.
We can remove an element from list by del() (removes element at the specified index,but not returns the value),remove() (removes by element value),pop()(removes the element at the specified index and returns the value).
>>list1=["red","orange","blue","green"]
>>del list1[2]
>>list1
['red','orange','green']
>>list2=["tiger","snake","giraffee","goat"]
>>list2.remove("giraffee")
>>list2
['tiger','snake','goat']
>>list3=["milk","Sugar","rice"]
>>list3.pop(1)
sugar
['milk','rice']
Like as list, we can remove elements in dictionary by using del(),pop() method but removes by the specified key name:
>>dict1={"Name":"Ananth","weight":68,"Height":180}
>>del dict1["Height"]
>>print(dict1)
{'Name':Ananth','weight':68}
>>dict2={"Vehicle":"Bike","Model":"Bullet 350","price":260000}
>>dict1.pop("price")
>>print(dict2)
{'vehicle':'Bike','Model':'Bullet'}
Unlike list and dictionary we cannot directly remove an element using index value.
Some methods
Sort() / sorted()
We can use sort() method in list to sort the elements in the list.
In dictionary we can use this sorted() method to sort the keys in the dictionary by default.
The elements in the tuple cannot be sorted as it is immutable
>>list1=["anushya","nikitha","dhanush","banerjii"]
>>list1.sort()
['anushya','banerjii','dhanush','nikitha']
>>dict1={1:"dhoni",4:"virat",2:"rahul",3:"atlee"]
>>print(sorted(dict1.keys()))
[1,2,3,4]
count()
The count() method returns the number of times the specified element occurs in list and tuple.
In dictionary the count() method is not defined.
>>Numbers=[2,3,4,6,3,7,8,2]
>>print(Numbers.count(3))
2
>>tuple1=(5,5,2,3,4,9,2)
>>print(tuple1.count(5))
2
Conclusion
And finally we have learned creating, manipulating, removing and some functions in list vs tuple vs dictionary. I think we have covered the major difference in list vs tuple vs dictionary. and I hope you would have got a clarification on using tuple vs dictionary vs list.Then what are you waiting for? just go and practice what you learned:)
keep coding!!!