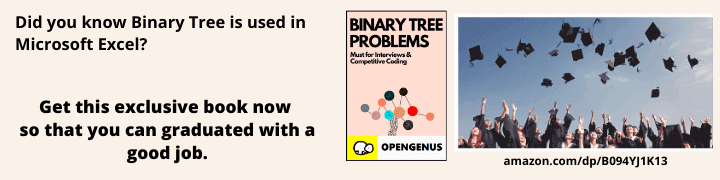
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In Python, date and time are not represented as specific data types, but a special module called datetime module can be imported in order to work with date and time as objects.
Installation
This is an inbuilt module, hence it does not require any specific installation.
How it works
The classes in this module are used for manipulating dates and times. These classes provide a number of functions which can be used to work with them. As these are datetime objects in Python, when you manipulate them, you are actually manipulating objects and not any strings or timestamps.
Types of objects in the datetime module
The objects in this module can be categorised into 2 types:
- aware
- naive
1. aware-
An aware object represents a specific moment in time which cannot be assumed. This object can locate itself relative to other aware objects. It takes help of applicable algorithmic and political time adjustments, such as time zone and daylight saving time information.
2. naive-
A naive object does not contain enough information to precisely locate itself relative to other objects. Depending on the program, a naive object represents Coordinated Universal Time (UTC), local time, or other timezone time. Naive objects are thus easy to understand and work with, but at the cost of ignoring certain aspects of reality.
Classes
There are 6 types of classes in datetime module:
- date
- time
- datetime
- timedelta
- tzinfo
- timezone
1. date-
An idealized naive date, in accordance with the current Gregorian calendar.
Attributes- year, month and day.
2. time-
An idealized time, independent of any particular day.
Attributes- hour, minute, second, microsecond, and tzinfo.
A time object is aware if both of these are true:
- t.tzinfo is not None
- t.tzinfo.utcoffset(None) does not return None.
Otherwise, it is naive.
3. datetime-
A combination of a date and a time.
Attributes- year, month, day, hour, minute, second, microsecond, and tzinfo.
A datetime object is aware if both of these are true:
- d.tzinfo is not None
- d.tzinfo.utcoffset(d) does not return None
Otherwise, it is naive.
4. timedelta-
A duration expressing the difference between two date, time, or datetime instances, precise to microsecond resolution.
These objects don't have any distinction between aware and naive.
5. tzinfo-
For programs which require aware objects, datetime and time objects have an optional time zone information attribute, tzinfo. This attribute can be set to an instance of a subclass of the abstract tzinfo class. These tzinfo objects capture information like the offset from UTC time, the time zone name, and daylight savings.
These provide a customizable notion of time adjustment, like accounting for time zone and/or daylight saving time, to the datetime and time classes.
6. timezone-
It is a concrete tzinfo class supplied by the datetime module. The timezone class can represent simple timezones with fixed offsets from UTC, like UTC itself or North American EST and EDT timezones. Support of timezones at deeper levels of detail depends on the program.
Properties of datetime objects
- They are immutable
- They are hashable i.e they can be used as dictionary keys.
- They support efficient pickling via the pickle module
Date class
An object of this class represents a date in the format YYYY-MM-DD in an idealized Gregorian calendar.
Functions:
Constructor:
class datetime.date(year, month, day)
Class methods:
-
date.today()
Return the current local date. -
date.fromtimestamp(timestamp)
Return the local date corresponding to the POSIX timestamp. -
date.fromordinal(ordinal)
Return the date corresponding to the proleptic Gregorian ordinal, where January 1 of year 1 has ordinal 1. -
date.fromisoformat(date_string)
Return a date corresponding to a date_string given in the format YYYY-MM-DD -
date.fromisocalendar(year, week, day)
Return a date corresponding to the ISO calendar date specified by the year, week and day.
Instance methods:
-
instace.replace(year=self.year, month=self.month, day=self.day)
Return a date with the same value, except for those parameters which are explicitly given new values by specifying the keyword arguments. -
instance.timetuple()
Return a time.struct_time such as returned by time.localtime(). -
instance.toordinal()
Return the proleptic Gregorian ordinal of the date. -
instance.weekday()
Return the day of the week as an integer, where Monday is 0 and Sunday is 6. -
instance.isoweekday()
Return the day of the week as an integer, where Monday is 1 and Sunday is 7. -
instance.isocalendar()
Return a 3-tuple, (ISO year, ISO week number, ISO weekday). -
instance.isoformat()
Return a string representing the date in ISO 8601 format, YYYY-MM-DD. -
instance.__ str __()
For a date d, str(d) is equivalent to d.isoformat(). -
instance.ctime()
Return a string representing the date. -
instance.strftime(format)
Return a string representing the date, controlled by an explicit format for the string. Format codes for hours, minutes or seconds will have value 0. -
instance.__ format __(format)
Return a string representing the date, but specifying a format string for a date object in formatted string literals or when using str.format().
Attributes:
Class attributes:
-
date.min
The earliest representable date, date(MINYEAR, 1, 1). -
date.max
The latest representable date, date(MAXYEAR, 12, 31). -
date.resolution
The smallest possible difference between non-equal date objects, timedelta(days=1).
Instance attributes (read-only):
-
instance.year
Range between MINYEAR and MAXYEAR inclusive. -
instance.month
Range between 1 and 12 inclusive. -
instance.day
Range between 1 and the number of days in the given month of the given year.
Implementation
import time
from datetime import date
today = date.today()
print(today)
my_birthday = date(today.year, 6, 24)
print(my_birthday)
if my_birthday < today:
my_birthday = my_birthday.replace(year=today.year + 1)
print(my_birthday)
time_to_birthday = abs(my_birthday - today)
print(time_to_birthday)
'''OUTPUT:
2020-04-30
2020-06-24
2020-06-24
55 days, 0:00:00
'''
Time class
A time object represents a local time of day, independent of any particular day, and subject to adjustment via a tzinfo object.
Functions:
Constructor:
class datetime.time(hour=0, minute=0, second=0, microsecond=0, tzinfo=None, *, fold=0)
Class methods:
- time.fromisoformat(time_string)
Return a time corresponding to a time_string in one of the formats emitted by time.isoformat(). It supports strings in the format:
HH[:MM[:SS[.fff[fff]]]][+HH:MM[:SS[.ffffff]]]
Instance methods:
-
instace.replace(hour=self.hour, minute=self.minute, second=self.second, microsecond=self.microsecond, tzinfo=self.tzinfo, * fold=0)
Return a time with the same value, except for those parameters which are explicitly given new values by specifying the keyword arguments.
To create a naive time from an aware time, without conversion of the time data, tzinfo=None can be specified. -
instance.isoformat(timespec='auto')
Return a string representing the time in ISO 8601 format. -
instance.__ str __()
For a time t, str(t) is equivalent to t.isoformat(). -
instance.strftime(format)
Return a string representing the time, controlled by an explicit format for the string. -
instance.__ format __(format)
Return a string representing the time, but specifying a format string for a time object in formatted string literals or when using str.format(). -
instance.utcoffset()
If tzinfo is None, returns None, else returns self.tzinfo.utcoffset(None), and raises an exception if the latter doesn’t return None or a timedelta object with magnitude less than one day. -
instance.dst()
If tzinfo is None, returns None, else returns self.tzinfo.dst(None), and raises an exception if the latter doesn’t return None, or a timedelta object with magnitude less than one day. -
instance.tzname()
If tzinfo is None, returns None, else returns self.tzinfo.tzname(None), or raises an exception if the latter doesn’t return None or a string object.
Class attributes:
-
time.min
The earliest representable time, time(0, 0, 0, 0). -
time.max
The latest representable time, time(23, 59, 59, 999999). -
time.resolution
The smallest possible difference between non-equal time objects, timedelta(microseconds=1).
Instance attributes (read-only):
-
instance.hour
In range(24). -
instance.minute
In range(60). -
instance.second
In range(60). -
instance.microsecond
In range(1000000). -
instance.tzinfo
The object passed as the tzinfo argument to the time constructor, or None. -
instance.fold
In [0, 1]. Used to disambiguate wall times during a repeated interval in case of daylight savings or when the UTC offset for the current zone is decreased for political reasons. 0 represents the earlier of the two moments with the same wall time represented and 1 for the later.
Datetime class
A datetime object is a single object containing all the information from a date object and a time object.
Functions:
Constructor:
class datetime.datetime(year, month, day, hour=0, minute=0, second=0, microsecond=0, tzinfo=None, *, fold=0)
Class methods:
-
datetime.today()
Return the current local datetime, with tzinfo None. -
datetime.now(tz=None)
Return the current local date and time. -
datetime.utcnow()
Return the current UTC date and time, with tzinfo None. -
datetime.fromtimestamp(timestamp, tz=None)
Return the local date and time corresponding to the POSIX timestamp. -
datetime.utcfromtimestamp(timestamp)
Return the UTC datetime corresponding to the POSIX timestamp, with tzinfo None. -
datetime.fromordinal(ordinal)
Return the datetime corresponding to the proleptic Gregorian ordinal. -
datetime.combine(date, time, tzinfo=self.tzinfo)
Return a new datetime object whose date components are equal to the given date object’s, and whose time components are equal to the given time object’s. -
datetime.fromisoformat(date_string)
Return a datetime corresponding to a date_string in one of the formats emitted by date.isoformat() and datetime.isoformat(). It supports strings in the format:
YYYY-MM-DD[ * HH[:MM[:SS[.fff[fff]]]][+HH:MM[:SS[.ffffff]]]]
where * can match any single character.
-
datetime.fromisocalendar(year, week, day)
Return a datetime corresponding to the ISO calendar date specified by year, week and day. The non-date components are populated with their normal default values. -
datetime.strptime(date_string, format)
Return a datetime corresponding to date_string, parsed according to format specified by codes.
Instance methods:
-
instance.date()
Return date object with same year, month and day. -
instance.time()
Return time object with same hour, minute, second, microsecond and fold. tzinfo is None. -
instance.timetz()
Return time object with same hour, minute, second, microsecond, fold, and tzinfo attributes. -
instance.replace(year=self.year, month=self.month, day=self.day, hour=self.hour, minute=self.minute, second=self.second, microsecond=self.microsecond, tzinfo=self.tzinfo, * fold=0)
Return a datetime with the same attributes, except for those parameters which are explicitly given new values by specifying the keyword arguments.
To create a naive datetime from an aware datetime with no conversion of date and time data, tzinfo=None can be specified. -
instance.astimezone(tz=None)
Return a datetime object with new tzinfo attribute tz, adjusting the date and time data so the result is the same UTC time as itself, but in tz’s local time. -
instance.utcoffset()
If tzinfo is None, returns None, else returns self.tzinfo.utcoffset(self), and raises an exception if the latter doesn’t return None or a timedelta object with magnitude less than one day. -
instance.dst()
If tzinfo is None, returns None, else returns self.tzinfo.dst(self), and raises an exception if the latter doesn’t return None or a timedelta object with magnitude less than one day. -
instance.tzname()
If tzinfo is None, returns None, else returns self.tzinfo.tzname(self), raises an exception if the latter doesn’t return None or a string object, -
instance.timetuple()
Return a time.struct_time such as returned by time.localtime(). -
instance.utctimetuple()
If datetime instance d is naive, this is the same as d.timetuple() except that tm_isdst's value is enforced to 0 because DST is never in effect for a UTC time.
If d is aware, d is normalized to UTC time, by subtracting d.utcoffset(), and a time.struct_time for the normalized time is returned, tm_isdst is again enforced to 0. -
instance.toordinal()
Return the proleptic Gregorian ordinal of the date. -
instance.timestamp()
Return POSIX timestamp corresponding to the datetime instance. -
instance.weekday()
Return the day of the week as an integer, where Monday is 0 and Sunday is 6. -
instance.isoweekday()
Return the day of the week as an integer, where Monday is 1 and Sunday is 7. -
instance.isocalendar()
Return a 3-tuple, (ISO year, ISO week number, ISO weekday). -
instance.isoformat(sep='T', timespec='auto')
Return a string representing the date and time in ISO 8601 format:
YYYY-MM-DDTHH:MM:SS.ffffff, if microsecond is not 0
and
YYYY-MM-DDTHH:MM:SS, if microsecond is 0.
If utcoffset() does not return None, a string is appended, giving the UTC offset:
YYYY-MM-DDTHH:MM:SS.ffffff+HH:MM[:SS[.ffffff]], if microsecond is not 0
and
YYYY-MM-DDTHH:MM:SS+HH:MM[:SS[.ffffff]], if microsecond is 0.
-
instance. str ()
For a datetime instance d, str(d) is equivalent to d.isoformat(' '). -
instance.ctime()
Return a string representing the date and time. -
instance.strftime(format)
Return a string representing the date and time, controlled by an explicit format for the string. -
instance.__ format __(format)
Return a string representing the date and time, but specifying a format string for a datetime object in formatted string literals or when using str.format().
Class attributes:
-
datetime.min
The earliest representable datetime, datetime(MINYEAR, 1, 1, tzinfo=None). -
datetime.max
The latest representable datetime, datetime(MAXYEAR, 12, 31, 23, 59, 59, 999999, tzinfo=None). -
datetime.resolution
The smallest possible difference between non-equal datetime objects, timedelta(microseconds=1).
Instance attributes (read-only):
-
instance.year
Range between MINYEAR and MAXYEAR inclusive. -
instance.month
Range between 1 and 12 inclusive. -
instance.day
Range between 1 and the number of days in the given month of the given year. -
instance.hour
In range(24). -
instance.minute
In range(60). -
instance.second
In range(60). -
instance.microsecond
In range(1000000). -
instance.tzinfo
The object passed as the tzinfo argument to the datetime constructor, or None. -
instance.fold
In [0, 1]. Used to disambiguate wall times during a repeated interval in case of daylight savings or when the UTC offset for the current zone is decreased for political reasons. 0 represents the earlier of the two moments with the same wall time represented and 1 for the later.
Timedelta class
A timedelta object represents a duration, the difference between two dates or times.
Functions:
Constructor:
class datetime.timedelta(days=0, seconds=0, microseconds=0, milliseconds=0, minutes=0, hours=0, weeks=0)
Instance methods:
- instace.total_seconds()
Return the total number of seconds contained in the duration. Equivalent to td / timedelta(seconds=1). The division form is used directly (e.g. td / timedelta(microseconds=1)) for other time units.
Attributes:
Class attributes:
-
timedelta.min
The most negative timedelta object, timedelta(-999999999). -
timedelta.max
The most positive timedelta object, timedelta(days=999999999, hours=23, minutes=59, seconds=59, microseconds=999999). -
timedelta.resolution
The smallest possible difference between non-equal timedelta objects, timedelta(microseconds=1).
Instance attributes (read-only):
-
instance.days
Range between -999999999 and 999999999 inclusive -
instance.seconds
Range between 0 and 86399 inclusive -
instance.microseconds
Range between 0 and 999999 inclusive
Timezone class
It is a subclass of tzinfo, each instance represents a timezone defined by a fixed offset from UTC.
These objects cannot be used to represent timezones of locations where different offsets are used in different days of the year or where historical changes have been made to civil time.
Functions:
Constructor:
class datetime.timezone(offset, name=None)
Instance methods:
-
instace.utcoffset(dt)
Return the fixed value specified when the timezone instance is constructed. -
instance.tzname(dt)
Return the fixed value specified when the timezone instance is constructed. -
instance.dst(dt)
Always returns None because DST is never in effect for a UTC time. -
instance.fromutc(dt)
Return dt + offset.
Attributes:
Class attributes:
- timezone.utc
The UTC timezone, timezone(timedelta(0)).
Tzinfo class
This is an abstract base class, so it should not be instantiated directly. A subclass of tzinfo should be defined to capture information about a particular time zone.
An instance of (a concrete subclass of) tzinfo can be passed to the constructors for datetime and time objects. These objects' attributes correspond to local time, and the tzinfo object supports methods which reveal offset of local time from UTC, the name of the time zone, and DST offset, relative to the date or time object passed.
Functions
-
tzinfo.utcoffset(dt)
Return total offset of local time from UTC. -
tzinfo.dst(dt)
Return the daylight saving time (DST) adjustment, as a timedelta object or None if DST information is unknown. -
tzinfo.tzname(dt)
Return the time zone name corresponding to the datetime object dt, as a string. -
tzinfo.fromutc(dt)
It is called from the default datetime.astimezone() implementation. dt.tzinfo is self, and dt’s date and time data express UTC time. This function adjusts the date and time data and returns an equivalent datetime object in self’s local time.
Implementation
from datetime import time, tzinfo, timedelta
class TZ1(tzinfo):
def utcoffset(self, dt):
return timedelta(hours=1)
def dst(self, dt):
return timedelta(0)
def tzname(self,dt):
return "+01:00"
def __repr__(self):
return f"{self.__class__.__name__}()"
t = time(12, 10, 30, tzinfo=TZ1())
print(t)
print(t.isoformat())
print(t.dst())
print(t.tzname())
print(t.strftime("%H:%M:%S %Z"))
print('The {} is {:%H:%M}.'.format("time", t))
'''OUTPUT:
12:10:30+01:00
12:10:30+01:00
0:00:00
+01:00
12:10:30 +01:00
The time is 12:10.
'''
str Format Codes
Questions
Consider the following code:
tz=tzinfo(timedelta(hours = 1))
t=time(12, 10, 30, tzinfo = tz)
print(t)
What will the output for the above code?
With this article at OpenGenus, you must have the complete idea of handling date and time in Python using datetime module. Enjoy.