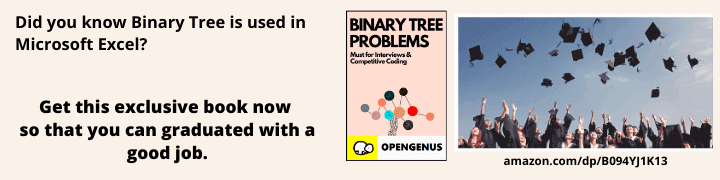
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, Python Function Arguments are explored in detail with examples including Positional argument, keyword argument, default argument and variable length argument.
Table of Contents |
---|
1)Glance of Arguments |
2)Positional arguments |
3)Keyword arguments |
4)Default arguments |
5)Variable length arguments |
1) Glance of Arguments
Let's start by defining what function arguments are.
A user-defined function takes required information in the form function arguments.
The words arguments and parameters are used interchangeably.
A function can take any number of arguments from zero to many and these are enclosed in parantheses and are seperated by commas.
Syntax:
function(arg1,agr2,arg3,.....,argN)
Let's look at some examples.
Example:
def func():
print("Hello World!")
func()
The 'func' function takes in zero arguments and just prints the message "Hello World!" upon calling.
Hello World!
Example:
def add(num1,num2,num3):
return num1+num2+num3
print(add(2,3,4))
The 'add' function takes in three numbers as arguments and returns the sum of those three numbers.
9
Example:
def greet(name):
print("Hello",name)
greet("opengenus")
The 'greet' function takes in a name as argument and prints the greeting.
Hello opengenus
In python,there's no need for defining datatypes of function arguments.
There are various types of arguments that can be used in python.
Let's study them in detail.
2)Positional arguments
Positional arguments can also be termed as Required arguments.
Here, the position of the arguments passed matters.
The arguments passed in the function call must be passed correctly in order as per function definition.
For example,
def function(name,age):
print('Hello',name,',your age is',age)
function('opengenus',25)
In above snippet the arguments are passed correctly in the function call as per the function definition. Hence, correct output will be obtained.
Hello opengenus ,your age is 25
What if arguments are not passed in the correct order?
Let's see it through an example.
def function(name,age):
print('Hello',name,',your age is',age)
function(25,'opengenus')
In the above snippet, the arguments defined in the function definition are name and age in order but in the function call the arguments are passed incorrectly, age is passed first and then name.
Hence, the output produced would be inappropriate
Hello 25 ,your age is opengenus
Sometimes passing arguments incorrectly may generate errors.
Let's look at it through an example.
def function(name,age):
if age>=18:
print('Hello',name,',You are eligible for voting')
else:
print('Hello',name,',You are not eligible for voting')
function(25,'opengenus')
Here, we'll check whether a person is eligible for voting or not. What if the arguments passed are wrong? The 'str' and 'int' objects can't be compared. Hence, an exception arises
TypeError: '>=' not supported between instances of 'str' and 'int'
The number of arguments passed in the function call must match with that of function definition.
For example,
def multiply(num1,num2,num3):
return num1*num2*num3
print(multiply(5,6,7))
Here the number of arguments passed in the function call is same that of function definition.
210
Let's see what happens if less or more number of arguments are passed in the function call.
def multiply(num1,num2,num3):
return num1*num2*num3
print(multiply(5,6,7,8))
The function definition has three arguments but four were passed in the funtion call.Hence, it generates an exception.
TypeError: multiply() takes 3 positional arguments but 4 were given
3)Keyword arguments
In this type of arguments, while passing values in the function call, the values can be passed using keywords.
Syntax:
function(arg1=val1,arg2=val2,arg3=val3,....,argN=valN)
Example:
def function(name,age):
print('Hello',name,',your age is',age)
function(name='opengenus',age=25)
Hello opengenus ,your age is 25
Here position of the values passed in the function call doesn't matter.The values can be passed in any order provided keywords are used.
def function(name,age):
print('Hello',name,',your age is',age)
function(age=25,name='opengenus')
The code will generate same output as above.
Hello opengenus ,your age is 25
The number of parameters in the function call and the function definition must be same otherwise an error will be generated.
def add(num1,num2,num3):
print('First number is:',num1)
print('Second number is:',num2)
print('Third number is:',num3)
add(num3=5)
TypeError: add() missing 2 required positional arguments: 'num1' and 'num2'
Positional arguments and keyword arguments can be used together. In such cases, Keyword arguments must follow Positional arguments.
def add(num1,num2,num3):
print('First number is:',num1)
print('Second number is:',num2)
print('Third number is:',num3)
add(3,4,num3=5)
First number is: 3
Second number is: 4
Third number is: 5
Positional arguments cannot be followed by Keyword arguments.
def add(num1,num2,num3):
print('First number is:',num1)
print('Second number is:',num2)
print('Third number is:',num3)
add(num3=5,3,4)
SyntaxError: positional argument follows keyword argument
4)Default arguments
Python allows function arguments to have default values.
Passing the value for the argument with default value in the function call is optional.
Syntax:
def function(arg1=defaultvalue1,arg2=defaultvalue2,....,argN=defaultvalueN):
-----
-----
Example:
def greet(name='Guest'):
print("Hello",name)
greet("opengenus")
greet()
In the first function call a name is passed, hence it greets with the name.
In the second function call no name is passed, hence it greets with the default value of the argument.
Hello opengenus
Hello Guest
Default argument and Non-default arguments can be used together.
Any number of arguments can have default values but, Default arguments must follow non-default arguments.
def add(num1,num2=4,num3=5):
print('First number is:',num1)
print('Second number is:',num2)
print('Third number is:',num3)
add(3)
First number is: 3
Second number is: 4
Third number is: 5
Non-default arguments cannot follow Default arguments.
def add(num1=3,num2,num3):
print('First number is:',num1)
print('Second number is:',num2)
print('Third number is:',num3)
add(4,5)
SyntaxError: non-default argument follows default argument
5)Variable length arguments
This type of arguments are used when we don't know the number of values to be passed to the function in advance.
In Variable length arguments, a variable number of arguments from zero to many can be passed to the function.
These can also be termed as Arbitrary arguments.
Syntax:
function(*args)
*args (Variable length Non-Keyword Arguments)
In this, a variable number of non-keyworded arguments can be passed to the function.
Example:
Suppose we want to add some arbitrary number of integers, we can use variable length arguments.
def add(*args):
summation=0
for arg in args:
summation+=arg
return summation
print(add(1,2,3,4))
10
**kwargs (Variable length Keyword Arguments)
A special form of variable length arguments where arbitrary number of keyworded arguments can be passed to the function.
Internally the Keyworded variable length arguments will be stored in the form of a dictionary.
Example:
Suppose we want to pass some details of a person, we can pass using Variable length keyword arguments
def details(**kwargs):
for k,v in kwargs.items():
print(k,'->',v)
details(name='opengenus',age=25,course='computer')
name -> opengenus
age -> 25
course -> computer
Variable length arguments can be combined with Positional arguments and Keyword arguments. In such cases,
Variable length arguments are followed by Positional arguments.
Example:
def function(num,*args):
print(num,args)
function(1,2,3,4,5)
1 (2, 3, 4, 5)
Keyword arguments are followed by Variable length arguments.
Example:
def function(*args,num):
print(num,args)
function(2,3,4,5,num=1)
1 (2, 3, 4, 5)
Positional arguments cannot be followed by Variable length arguments.
Example:
def function(*args,num):
print(num,args)
function(1,2,3,4,5)
TypeError: function() missing 1 required keyword-only argument: 'num'
*args and **kwargs can be used together.
Example:
def details(*args,**kwargs):
print(args)
for k,v in kwargs.items():
print(k,'->',v,end=' ')
details(1,2,3,name='opengenus',age=25,course='computer')
(1, 2, 3)
name -> opengenus age -> 25 course -> computer
With this article at OpenGenus, you must have the complete idea of Python Function Arguments.