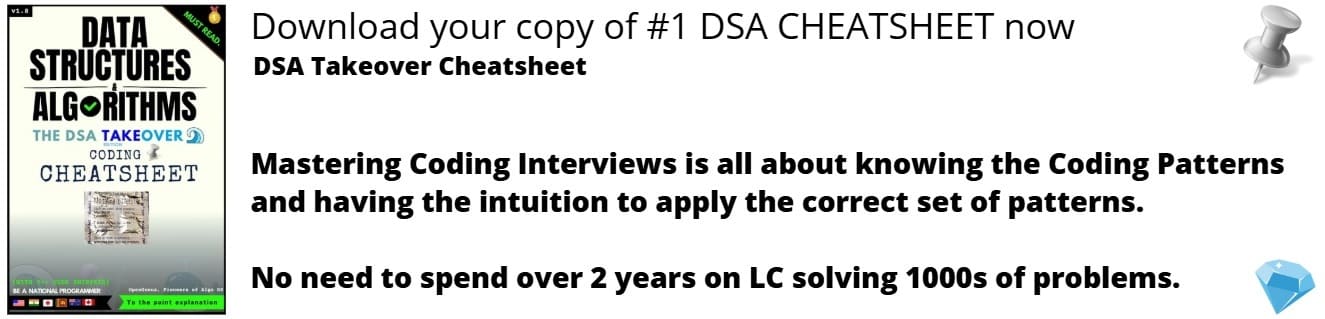
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explored how to develop a Python script to control cursor and stimulate actions like clicking, scrolling, click and drag.
Table of contents:
- Introduction to PyAutoGui
- Determine the screen resolution
- Moving the mouse cursor
- Getting the current position of mouse cursor
- Click the mouse cursor
- Scrolling the mouse cursor
- Click and drag the mouse cursor
Introduction to PyAutoGui
PyAutoGui is a Python module for automation with the Graphical User Interface (GUI). It can be used to programmatically control the mouse cursor. To install just run the following command in the terminal.
pip install pyautogui
Determine the screen resolution
By using the pyautogui.size()
function, we can determine the screen resolution of the user running the python program, this allow us to determine the max position the mouse cursor can travel.
import pyautogui
width_height = pyautogui.size()
print(width_height)
print(width_height[0])
print(width_height[1])
output
Size(width=1920, height=1080)
1920
1080
Moving the mouse cursor
The pyautogui.moveTo()
function will move the mouse cursor to the specific location on the screen. The duration=1
sets out the speed in which the mouse cursor is moving.
import pyautogui
# move to specific position
pyautogui.moveTo(100, 100, duration=1)
pyautogui.moveTo(200, 100, duration=1)
pyautogui.moveTo(200, 200, duration=1)
pyautogui.moveTo(100, 200, duration=1)
The pyautogui.move()
function will move the mouse cursor to the relative position where the mouse cursor is currently located at.
import pyautogui
# move to relative position of current mouse cursor
pyautogui.move(100, 0, duration=1)
pyautogui.move(0, 100, duration=1)
pyautogui.move(-100, 0, duration=1)
pyautogui.move(0, -100, duration=1)
Getting the current position of mouse cursor
We can use the pyautogui.position()
function to retrieve the current position of mouse cursor.
import pyautogui
position = pyautogui.position()
print(position)
print(position[0])
print(position[1])
An alternative is to run the pyautogui.mouseInfo()
function. This will load the MouseInfo window (see image below) and it provides the current position of mouse cursor.
import pyautogui
pyautogui.mouseInfo()
Click the mouse cursor
The pyautogui.click()
function will simulate the clicking of the mouse at the specific (x,y) coordinates.
import pyautogui
pyautogui.click(320,41)
Scrolling the mouse cursor
The pyautogui.scroll()
function will simulate the scrolling of the mouse cursor.
import pyautogui
# scroll up
pyautogui.scroll(20)
# scroll back down
pyautogui.scroll(-20)
Click and drag the mouse cursor
We can use the pyautogui.dragTo()
function to click and drag the mouse cursor. In the example below we are trying to draw a circle in our paint
software. First we import the time
module, this allow us to implement a cooldown timer, which will allow the user sufficient time to terminate the program by moving the mouse cursor to one of the 4 corners of the screen.
We also import the math
module, to perform some calculation of the (x,y) coordinates of a circle.
Once we have the relevant coordinates, we will use the pyautogui.click()
function to make our paint
window active. Next, we will use the pyautogui.dragTo()
function to click and drag on the paint
window to draw the circle based on the coordinates we had calculated.
# Click and drag the mouse
import pyautogui
import math
import time
# 5 seconds cooldown to allow user to terminate the program by moving the mouse to one of the 4 corners
time.sleep(5)
# Radius
R = 100
# measuring screen size
(x,y) = pyautogui.size()
# locating center of the screen
(X,Y) = (int(x/2), int(y/2))
#click the paint window tab
pyautogui.click(300,1064)
# offsetting by radius
pyautogui.moveTo(X,Y+R)
# converts angle(degrees) to radians
# Radians = π * angle / 180
# x = radius * Sin(Radian(angle))
# y = radius * Cos(Radian(angle));
for i in range(361):
if i%6==0: #adjust speed
pyautogui.dragTo(X+R*math.sin(math.radians(i)), Y+R*math.cos(math.radians(i)))
With this article at OpenGenus, you must have the complete idea of using Python to control the mouse cursor.