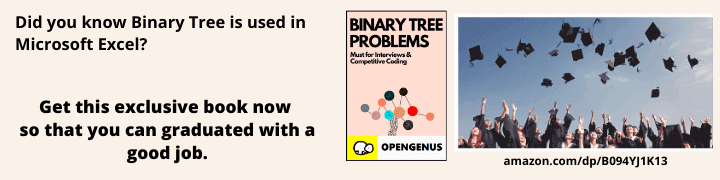
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 20 minutes | Coding time: 5 minutes
Printf() and Scanf() are inbuilt library functions in C language that perform formatted input and formatted output functions. These functions are defined and declared in stdio.h header file. The 'f' in printf and scanf stands for 'formatted'. So, both the functions printf() and scanf() use codes within a format string to specify how output or input values should be formatted.
To use printf() and scanf(), you need to import the header file:
#include <stdio.h>
Printf()
It prints anything between double quotes with format specifiers %c, %d,%f, etc. to show a value of a variable or a constant and can also have escape sequence characters like \n, \t for new-line and horizontal tab respectively.
Syntax:
printf("(string) %format_specifier (string)",variable);
Example for printing an integer:
printf("The Value of A is: %d", a);
Example:
Complete code:
#include<stdio.h>
int main()
{
int a = 10;
printf("The Value of A is: %d",a);
return 0;
}
Output:
The Value of A is: 10
Scanf()
It allows us to read one or multiple values entered by the user through the keyboard at the console. We can place as many format specifiers as many inputs we want with or without a format.
Syntax:
scanf(%format_specifier, &pointer_to_variable);
Example:
This is the complete example of taking an integer as input:
#include<stdio.h>
int main()
{
int a;
printf("Enter value for A" );
scanf("%d",&a);
return 0;
}
Output:
// The value entered by the user will be stored in variable a
Format Specifiers
To display and taking in specific values with scanf() and printf() functions, use specific format specifiers as follows:
Format specifier | Description |
---|---|
%d | Integer |
%f | Float |
%c | Character |
%s | String |
%u | Unsigned Integer |
%ld | Long Integer |
Code for considering character
In this C code, we take a character as input and print that character.
#include<stdio.h>
int main()
{
char a;
printf("Enter value for A" );
scanf("%c",&a);
printf("The value you entered is : %c", a);
return 0;
}
This will give you an idea for using it with other datatypes.
Basic Code for Printf() and Scanf()
#include<stdio.h>
int main()
{
int a,b,sum;
printf("Enter value for A" );
scanf("%d",&a);
printf("Enter value for B" );
scanf("%d",&b);
sum = a+b;
printf("The Sum of A & B is :",&sum);
return 0;
}
Basic Differences in Printf() and Scanf()
Few Differences between printf and scanf are:-
-
printf() function outputs data to the standard output i.e. to the console . while scanf() function reads data from the standard input i.e. input devices
-
printf() rarely uses pointer in a few cases but scanf() always uses a pointer to assign value to the given variable.
-
printf() and scanf() both have the same return type of integer. but, printf() returns the no. of characters it has successfully printed on the console whereas scanf () returns 0,1 or EOF based on the format specifier provided