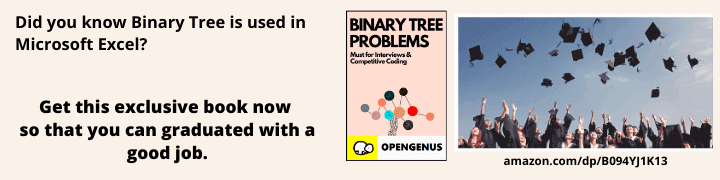
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
The shutil module in Python offers a range of high level operations and functions to work with files and collections of files. The functionalities provided are copying a file, moving a file, disk usage, directory removal and many more.
List of functions covered in this article:
- copyfile
- copymode
- copystat
- copy
- copy2
- copytree
- rmtree
- move
- disk_usage
copyfileobj
This function is used to copy the contents of the file or file-like object from the source to the destination.
Syntax:
copyfileobj(fsrc, fdst,length)
Parameter:
- fsrc : A file-like object of the source file.
- fdst :A file-like object of the destination file.
- lenght(optional): An integer number denoting the buffer size.
Return type :No return type.
Example:
#importing shutil module
import shutil
src="Your source file name or path of the file."
dst="Your destination file name or path of the file."
#opening the source file in read mode and get the file object in fsrc
fsrc=open(src,'r')
#opening the destination file in write mode and get the file object in fdst
fdst=open(dst,'w')
#using copyfileobj to copy file object from fsrc to fdst
shutil.copyfileobj(fsrc, fdst)
#closing file objects
f1.close()
f2.close()
copyfile
This function is used to copy the contents of the source file to the destination file in the most efficient way possible.This function does not copy the metadata of the source file to the destination file.
Syntax:
copyfile(src, dst, *, follow_symlinks=True)
Parameters:
- src : A string containing the path of the source file.
- dst : A string containing the path of the destination file.
- follow_symlinks (optional) :This parameter represents the symbolic link.Default value of this parameter is True.If it is set to False and the source represent a symbolic link then instead of creating a file a new symbolic link is created.
Note: The ‘*’ in parameter list indicates that all following parameters are keyword-only parameters and they can be provided using their name, not as positional parameter.
Return type: A string representing the path of the newly created file.
Example:
import shutil
imort os
pth="path to folder containg destination file."
src="Path of the source file."
dst="Path to thre destination file."
#Checking destination folder before copying file.
print("Destination folder before copying:",os.listdir(path))
#Copying the file and storing it's path in dpath
dpath=shutil.copyfile(src,dst)
print("Psth of the new file:"+dpath)
#Checking destination folder after copying file.
print("Destination folder before copying:",os.listdir(path))
copymode
This function is used to copy the permission bits of the source file to the destination file.The content and ownership of the source and destination file remain
unchanged.
Syntax :
copymode(src, dst, *, follow_symlinks=True)
Parameter :
- src : A string containing the path of the source file.
- dst : A string containing the path of the destination file.
- follow_symlinks (optional) :This parameter represents the symbolic link.Default value of this parameter is True.If it is set to False and the source represent a symbolic link then instead of creating a file a new symbolic link is created.
Note: The ‘*’ in parameter list indicates that all following parameters are keyword-only parameters and they can be provided using their name, not as positional parameter.
Return type : No return type.
Example:
import os
import shutil
src = "Path of the source file."
dst = "Path of the destination file."
#Checking the permission bits of source and destination before modecopy is used.
print("Permission bits before modecopy is used:")
print("Source:", oct(os.stat(src).st_mode)[-3:])
print("Destination:", oct(os.stat(dest).st_mode)[-3:])
# Using copymode to copy permission bits of src to dst
shutil.copymode(src,dst)
#Checking the permission bits of destination path after using copymode function.
print("Updated permission bits of destination:", oct(os.stat(dest).st_mode)[-3:])
copystat
The copystat function can be said as a better version of the copymode function as it allows the user to copy the permission bits,last access time, last modification time, and flags from the source file to the destination file.
Syntax :
copystat(src, dst, *, follow_symlinks=True)
Parameters:
- src : A string containing the path of the source file.
- dst : A string containing the path of the destination file.
- follow_symlinks (optional) :This parameter represents the symbolic link.Default value of this parameter is True.If it is set to False and the source represent a symbolic link then instead of creating a file a new symbolic link is created.
Note: The ‘*’ in parameter list indicates that all following parameters are keyword-only parameters and they can be provided using their name, not as positional parameter.
Return type: No return type.
Example:
#time module in this program is used to get the last modification time ,and last access time of a file.
import os
import shutil
import time
src = "Path of the source file."
dst = "Path of the destination file."
#checking the permission bits,last access time,last modification time and
#flags value of source and destination files before using copystat.
print("Metadata of source file before using copystat function:")
print("Permission bits:", oct(os.stat(src).st_mode)[-3:])
print("Last access time:", time.ctime(os.stat(src).st_atime))
print("Last modification time:", time.ctime(os.stat(src).st_mtime))
print("Metadata of destination file before using copystat function:")
print("Permission bits:", oct(os.stat(dest).st_mode)[-3:])
print("Last access time:", time.ctime(os.stat(dest).st_atime))
print("Last modification time:", time.ctime(os.stat(dest).st_mtime))
# print("User defined Flags:", os.stat(dest).st_flags)
#Using copystat to copy the permission bits last access time,
#last modification time and flags value from source to destination
shutil.copystat(src,dst)
#Checking the permission bits last access time, last modification time
#and flags value of destination.
print("Metadata of destination after using copystat:")
print("Permission bits:", oct(os.stat(dest).st_mode)[-3:])
print("Last access time:", time.ctime(os.stat(dest).st_atime))
print("Last modification time:", time.ctime(os.stat(dest).st_mtime))
copy
This function is used to copy a file from the source to the destination.It copies the file data and the file’s permission mode.Other metadata, like the file’s creation and modification times, is not preserved.
Syntax:
copy(src, dst, *, follow_symlinks=True)
Parameter:
- src : A string containing the path of the source file.
- dst : A string containing the path of the destination file.
- follow_symlinks (optional) :This parameter represents the symbolic link.Default value of this parameter is True.If it is set to False and the source represent a symbolic link then instead of creating a file a new symbolic link is created.
Note: The ‘*’ in parameter list indicates that all following parameters are keyword-only parameters and they can be provided using their name, not as positional parameter.
Return type: It returns a string containing the path of the newly copied/created file.
Example:
import os
import shutil
path="Path of the destination folder"
# List files and directories in destination folder before copying.
print("List of files and directories in destination folder/directory before copying file:",os.listdir(path))
src="Path of source file."
dst="Path of destination file."
#Checking the file permission of the source file
perm = os.stat(source).st_mode
print("File Permission mode:", perm, "\n")
# Copy the content of source file to destination file.
dpath=shutil.copy(src,dst)
#List of files and directories in destination folder.
print("Destination files and directory list:",os.listdir(path))
#Checking the file permission of the destination
perms = os.stat(destination).st_mode
print("File Permissions:", perm)
#Checking the path of newly copied/created file
print("Destination path:", dpath)
copy2
This function is used to copy a file from the source to the destination.It copies the file data and the file’s permission mode as well as other metadata, like the file’s creation and modification times, is also preserved.
Syntax:
copy2(src, dst, *, follow_symlinks=True)
Parameter:
- src : A string containing the path of the source file.
- dst : A string containing the path of the destination file.
- follow_symlinks (optional) :This parameter represents the symbolic link.Default value of this parameter is True.If it is set to False and the source represent a symbolic link then instead of creating a file a new symbolic link is created.
Note: The ‘*’ in parameter list indicates that all following parameters are keyword-only parameters and they can be provided using their name, not as positional parameter.
Return type: It returns a string containing the path of the newly copied/created file.
Example:
import os
import shutil
path="Path of the destination folder"
#Checking list of files and directories in destination folder/directory
#before copying file.
print("list of files and deirectories in destination folder/directory before copying file:",os.listdir(path))
src="Path of source file."
dst="Path of destination file."
#checking the metadeta of source file before using copy2.
mdata = os.stat(source)
print("Metadata:", mdata,"\n")
# Copy the content of source file to destination file.
dpath=shutil.copy2(src,dst)
#Checking list of files and directories in destination folder/directory
#after copying file.
print("After copying file:")
print(os.listdir(path))
#checking the metadata of the destination file after using copy2.
mdata=os.stat(destination)
print("Metadata:",mdata)
#Print path of newly copied/created file
print("Destination file path:",dpath)
copytree
This function is used to recursively copy the whole directory tree rooted at the source directory to the destination directory.The destination directory should not already exist and it is created at the time of copying the files.
Syntax:
copytree(src, dst, symlinks=False, ignore=None,
copy_function=copy2, ignore_dangling_symlinks=False)
Parameters:
- src : A string containing the path of the source directory.
- dst : A string containing the path of the destination directory.
- symlinks : If symlinks is true, symbolic links in the source tree are represented as symbolic links in the new tree and the metadata of the original links will be copied as far as the platform allows; if false or omitted, the contents and metadata of the linked files are copied to the new tree.
- ignore :If ignore is given, it must be a callable that will receive as its arguments the directory being visited by copytree(), and a list of its contents, as returned by os.listdir(). Since copytree() is called recursively, the ignore callable will be called once for each directory that is copied.
- copy_function :The default value of this parameter is copy2. We can use other copy function like copy, copytree, etc for this parameter.
- ignore_dangling_symlinks : It's value when set to True is used to put a silence on the exception raised if the file pointed by the symlink does not exist.
Return Type: It returns a string containing the path of the newly created directory.
Example:
import os
import shutil
path="Path containing the destination directory."
#Checking files and directories in the path
print("files and directories before copying:",os.listdir(path))
src="Path of the source directory"
dst="path of the destination directory"
# Copying the directory tree of source to the destination
dest=shutil.copytree(src,dst)
#Checking files and directories in the path
print("File and directories after copying files:",os.listdir(path))
#Checking path of newly created file/directory.
print("Destination path:", destination)
rmtree
This function is used to delete an entire directory tree given in the path.
Syntax:
rmtree(path,ignore_errors=False,onerror=None)
Parameter:
- path : A string containing the path of the file/directory to be removed.
- ignore_errors :If ignore_errors is true, errors resulting from failed removals will be ignored.
- oneerror: If ignore_errors is false or omitted, such errors are handled by calling a handler specified by onerror.
Return type: No return value
Example:
import shutil
imoprt os
dpath="path to the directory containing the directory/file to be removed."
path="path of the directory/file to be removed"
#Checking files and directories in the dpath before removal of file/directory.
print("files and directories before removal:",os.listdir(path))
# removing directory/file
shutil.rmtree(path)
#Checking files and directories in the dpath after removal of file/directory.
print("files and directories after removal:",os.listdir(path))
move
This function is used to recursively move a file or directory (src) to another location (dst).If the destination is an existing directory, then src is moved inside that directory. If the destination already exists but is not a directory, it may be overwritten depending on os.rename() semantics.
Syntax:
move(src, dst, copy_function=copy2)
Parameters:
- src : A string containing the path of the source directory/file.
- dst : A string containing the path of the destination directory/file.
- copy_function :The default value of this parameter is copy2. We can use other copy function like copy, copytree, etc for this parameter.
Return type: A string representing the path to the newly created/moved file/directory.
Example:
import os
import shutil
path ="path containing the destination directory"
#Checking list of files and directories in path
print("Before moving file:",os.listdir(path))
src="path of the source file"
dst="path of the destination file"
# Moving the content of source to destination
dpath=shutil.move(source, destination)
#checking the list files and directories in path.
print("After moving file:",os.listdir(path))
#Printing path of newly created file/directory
print("Destination path:",dpath)
disk_usage
This function is used to get disk usage statistics about the given path as a named tuple with the attributes total, used and free, which are the amount of total, used and free space, in bytes.
Syntax:
disk_usage(path)
Parameter:
- path: A string representing the path of a file or directory.
Return type:This function returns a named tuple with the attributes total, used and free which are the amount of total, used and free space, in bytes.
Example:
import os
import shutil
path = "Path of any file/directory of which the disk usage you wan tto get."
# Using shutil.disk_usage()
dskusage=shutil.disk_usage(path)
# Print disk usage of the target file/directory
print(dskusage)
With this article at OpenGenus, you will have a good idea of using shutil module in Python. Use it in your work and enjoy.