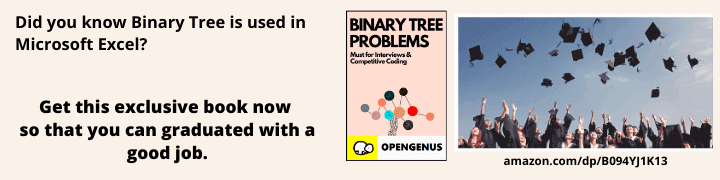
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we will learn about the structure pointers , in C/C++ we have a feature to use pointers with Structure. Like pointers to other data types we can use pointer to structure.
What are Structures?
Structures are a group of variables declared under one single name . It is done when we have a similar characteristics of the entities.
Like details of a student reading in coaching centre .Each of the student will be having a first name, last name, Date of birth, Sex , Contact Number , Address.
So , as all the students will have these information , so they are grouped under a single structure and it contains the details of all other variables inside it.
How to declare a structure pointer?
Similar to assigning pointers of other datatypes (that is by placing * before declaring the pointer), the pointer is directly assigned the address of the structure.
The process is bit different and it will be clear by the following example.
For example, we can assume a structure named address (holding details of the address), the following declaration means ptr_adr is a pointer of the type address
struct address * ptr_adr;
Now our pointer has been declared and this pointer can be assigned to any address we declare.
Important point to note that it is not necessary in C++ to use the struct key word while declaring the pointer but in C it is mandatory.
Why to use structure pointers?
Basically there are 2 primary reasons why we use structure pointers :-
- Passing structure data type as a reference to a function , as if we pass the pointer the function using the pointer can actually use that and modify the contents of the Structure that is being pointed by the pointer .
- To declare and create dynamic data structures , usually the structures are using dynamic memory allocation .When the structure is declared dynamically a pointer is returned having the address location where the node has been formed.Now this pointers has to be assigned to some variable to access that memory location and do all kinds of operations.
What if we don’t use struct pointers?
- One of the major drawbacks is that if we don’t use pointers, then we have to pass structure as a argument to functions . When the structure has less number of members and is small in size the overhead in copying the arguments between the function calls is less . But as the size of the structures would increase the overhead in copying these structure between function call would really be very costly in terms of time taken to copy the contents on to the stack.
- Thus it is large overhead which can be easily avoided if we use pointers because if we use pointers then there is no over head of copying these structure rather a just pointer is copied between function call thus directly saves a lot of time.
- On top of that if don’t use pointers then a copy of the structure is passed in the function instead of passing the actual structure(usual function call concept of call by value),so if we pass a structure and some operations are performed on the structure then that copy of the structure is only modified , so after the function call is over the original structure remains unaffected that it doesn’t get modified by the operations performed in the function because although we passed the structure during function a copy of that structure was passed instead of the original structure.
- But all these problems are solved if we use pointer because then we can directly modify the original structure.
- The above explanation clearly states the advantage of using pointer over not using pointer.
How exactly to assign the address of structure to pointer?
struct student {
char firstname[80],lastname[80],address[100];
int age;
} ulrich;
The variable ulrich is struct type variable .
struct student *s; // here we are declaring a structure pointer using the
//keyword struct struct is not necessary in C++
then
s= &ulrich;
Here in the above statement the address of the structure Ulrich is assigned to s structure type pointer .
Now all operations and modifications can be done using this pointer only.
How to access the members of the structure that being referenced using pointer?
To access the members of the structure referenced using the pointer we use the operator “->”.This operator is called as arrow operator. Using this we can access all the members of the structure and we can further do all operations on them.
To explain this we will continue with the above example.
s->age=18;
Important point to note:-
Alternatively if we don’t use the “->” we can first deference the pointer then we can access the member variables using the “.” operator .
Example
*(s).age=18;
Here we access the member age of the structure using the pointer s in both of the above cases.
To learn all the concepts of structure pointer we will be using this given below code which is code for timer .
/* Display a software timer. */
#include <stdio.h>
#define DELAY 128000
struct new_timer { int hr; int min; int sec;
};
void display(struct new_timer *t); void update(struct new_timer *t); void delay(void);
int main(void)
{
struct new_timer curtime;
curtime.hr = 0; curtime.min = 0; curtime.sec = 0;
for(;;) {
update(&curtime);
display(&curtime);
}
return 0; }
void update(struct new_timer *t) {
t->sec++; if(t->sec==60) {
t->sec = 0;
t->min++; }
if(t->min==60) { t->min = 0; t->hr++;
}
if(t->hr==24) t->hr = 0;
delay(); }
void display(struct new_timer *t) {
printf("%02d:", t->hr); printf("%02d:", t->min); printf("%02d\n", t->sec);
}
void delay(void)
{
long int t;
/* can be changed as per need */
for(t=1; t<DELAY; ++t) ;
}
Explanation of the code:-
The structure mnew_timer is declared and a variable of type is declared in main() named curtime now other functions involved in time update and time display are being called using the pointer of the variable curtime.
The delay function can be modified use as per our needs.