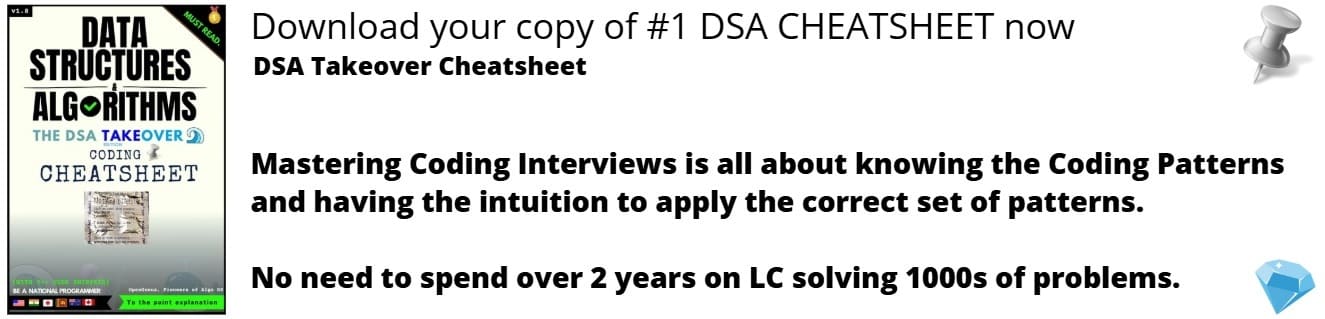
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
The keyword typedef is used to define new data type names in C/C++. Here we should not not mistaken that we are creating any new data type, we should carefully note that we are just giving new names to the data types already available to us by C/C++.
It really helps in writing codes which are more close to the machine because instead of writing long repeated lines. We can just use typedef and rest other will be done by the compiler.
It should be noted it is job of the compiler to do all the necessary replacements .
Another benefit of using typedef is that the code looks really well documented and it is a must when size of the code increases.
The common syntax of the typedef statement used during code :-
typedef type anyname;
It should be noted that the datatype that we are using over here is any of the available datatypes available in C/C++.
Another important fact to keep in mind that when we use typedef then the existing datatype for which new typedef is used is not absolute rather it should be noted that the new typed name give is just an alternative to the already existing datatype.
For example, if we replace the float we use in bank transactions is being replaced by some generic commonly used term like cost for double(a generic datatype).
We are using the word cost here so that it just gives an overall idea of its use in the real world.
typedef double cost;
Now when we use this statement then compiler will recognise that cost is just a alternative for the data type double.
cost amountleft;
Pay attention here amountleft is a variable of type double created by typedef cost.
Now one more trick to keep in mind is that the word used for alternative of data type (here cost) can be further used as an alternative of typedef.
That is we can continue the chain like this where a typedef variable can be used to created new typedef variable.
typedef cost accountmoney;
So , when we write the above code then the compiler just recoznizes that it
(accountmoney) it is further more one more alternative for the data type double as the the cost is originally of data type double.
Thus from above text we can infer that the typedef keyword makes the codes for handy and increases the readibility.
Pointers to Functions
Yes, you read it write it is possible to point functions using pointers.
It is one of the most amusing capabilities of C/C++.
How is this Possible?
Now , we all know that the functions are not varibales so we cannot use pointers like variables because pointers point to memory location of the variable.
But it should be noted although function is not a variable but function has some physical location in memory .
Now as we do in case of normal variables , once we point to that memory location having the function we can just call that using the pointer.
Simplest form of example:-
int (*a) (int);
Note that 'a' is not a function rather an pointer to function.
Now let's learn the process in the above code we just declared the pointer but it has not been intialized . So, it has to be intialized that is the address of the function is to be assigned to the pointer.
int myfunc(int z) {
return z+1;
}
Now it is the function to be pointed by the pointer.
a=myfunc;
Calling of the function
Here after assigning address to the function pointer we can call the function after dereferencing it.
int result = (*a)(3);
In the above code we are calling the function by defrencing it with an argument of our choice.
Why to use Function pointer?
- Now the function helps passing fucntions as arguments to other functions.
- Many functions having same signature , so we can use function pointer to point all these functions with same signature .
#include <stdio.h>
int adder(int a, int b) {
return a + b;
}
int minus(int a, int b) {
return a - b;
}
typedef int myfunction(int a, int b);
int callerfunction(myfunction *p, int a, int b) {
return p(a, b);
}
int main(void) {
int result;
result = callerfunction(&adder, 15, 20);
printf("Add Result: %d\n", result);
result = callerfunction(&minus, 15, 20);
printf("Substract Result: %d\n", result);
return 0;
}
Results Of the above code
Add Result: 35
Substract Result: -5
After reading all this I hope we are able to figure it out that it be really handy if we would use typedef function.
#include<stdio.h>
void upton(int n)
{
for (int i = 1; i <= n; ++i)
printf("%d\n", i);
}
void nth(int n)
{
printf("%d\n, n);
}
Notice they both are having similar signature.
So we can create function pointer which would be able to point both the functions .
It can be done by the method given below.
typedef void (*showall)(int);
This showall pointer can be used to point both the functions as signature is similar.
showall sh = &upton;
void (*shh)(int) = &upton;
//Notice that
Now lets call the function -
sh(99); // Prints all numbers up to n
(*sh)(99); // Prints all numbers up to n
So , from the above I sure it would be really clear that function pointer really make our life easy , otherwise the calling of functions using pointer to function would be really cumbersome.
Finally the whole above code in action using Typedef
#include<stdio.h>
void upton(int n)
{
for (int i = 1; i <= n; ++i)
printf("%d\n", i);
}
void nth(int n)
{
printf("%d\n", n);
}
typedef void (*showall)(int);
int main(){
showall sh = &upton;
void (*shh)(int) = &upton;
sh(99); // Prints all numbers up to n
(*shh)(99); // Prints all numbers up to n
return 0;
}
OUTPUT OF THE CODE is Numbers upto n(here 99) will be printed in both cases.
If still doubt about capabilities of typedef function the below code make evrything clear:-
int myfunction1(void (*showal)(int), int z){
//any code the function
showal(y);
return z+10;
}
int myfunction2(showall sh, int z){
//any code the function
sh(y);
//code
return z+10;
}
In the above above code notice how the typedef is saving alot hardwork . And the way typdef improves the readability of the code. Similar to passing function pointers as argument to functions and helping. The typedef also improves the code alot when a function return a function pointer.