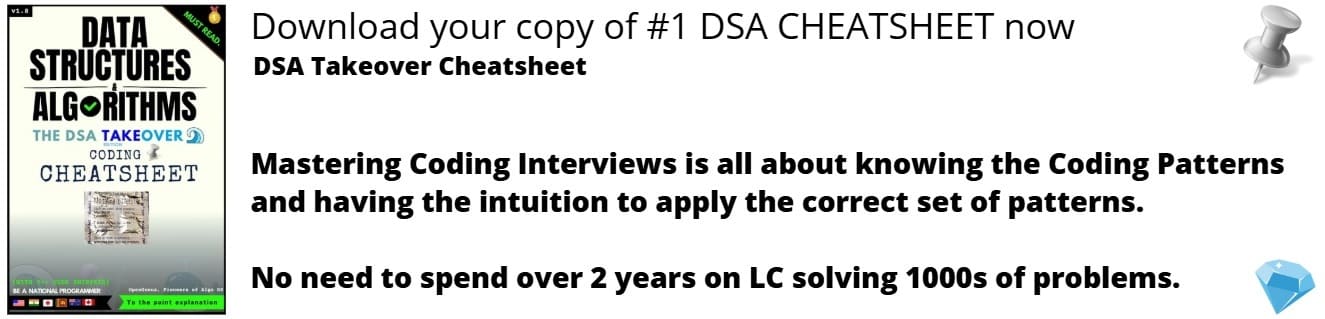
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
The “try” and “else” statements are used in Python for flow control.
An Exception, also known as run time error, is an event which occurs during the execution of a Python program. When that error occurs, it interrupts the Python program and generates an error message by printing a “Traceback” to the console with information about the exception and how it was raised.
In order to prevent the program interrupting, “catching” the exception is important. “Catching” here refers to a block of code executed once an exception is raised. The “except” statement contains this said block of code.
In other words, the “try” statement exists to give flow control to exceptions by passing series of instructions to the program in order to handle the raised error.
Exceptions can be raised in many ways, such as by passing invalid arguments to functions, performing certain illegal operations or intentionally/explicitly.
The “else” statement on the other hand contains the rest of the program code to be executed in the absence of errors.
Exception Types
Exception | Cause of Error |
---|---|
AssertionError | Raised when an assert statement fails. |
AttributeError | Raised when attribute assignment or reference fails. |
EOFError | Raised when the input() function hits end-of-file condition. |
FloatingPointError | Raised when a floating point operation fails. |
GeneratorExit | Raise when a generator's close() method is called. |
ImportError | Raised when the imported module is not found. |
IndexError | Raised when the index of a sequence is out of range. |
KeyError | Raised when a key is not found in a dictionary. |
KeyboardInterrupt | Raised when the user hits the interrupt key (Ctrl+C or Delete). |
MemoryError | Raised when an operation runs out of memory. |
NameError | Raised when a variable is not found in local or global scope. |
NotImplementedError | Raised by abstract methods. |
OSError | Raised when system operation causes system related error. |
OverflowError | Raised when the result of an arithmetic operation is too large to be represented. |
ReferenceError | Raised when a weak reference proxy is used to access a garbage collected referent. |
RuntimeError | Raised when an error does not fall under any other category. |
StopIteration | Raised by next() function to indicate that there is no further item to be returned by iterator. |
SyntaxError | Raised by parser when syntax error is encountered. |
IndentationError | Raised when there is incorrect indentation. |
TabError | Raised when indentation consists of inconsistent tabs and spaces. |
SystemError | Raised when interpreter detects internal error. |
SystemExit | Raised by sys.exit() function. |
TypeError | Raised when a function or operation is applied to an object of incorrect type. |
UnboundLocalError | Raised when a reference is made to a local variable in a function or method, but no value has been bound to that variable. |
UnicodeError | Raised when a Unicode-related encoding or decoding error occurs. |
UnicodeEncodeError | Raised when a Unicode-related error occurs during encoding. |
UnicodeDecodeError | Raised when a Unicode-related error occurs during decoding. |
UnicodeTranslateError | Raised when a Unicode-related error occurs during translating. |
ValueError | Raised when a function gets an argument of correct type but improper value. |
ZeroDivisionError | Raised when the second operand of division or modulo operation is zero. |
Error Handling Examples
- AssertionError:
In programming, the “assert” statement is used to declare that a particular condition while coding is true. While executing the program, if the condition is True, the next line of code is executed. However, if the condition is False, the program stops running and returns an AssertionError exception.
Like other exceptions, this exception can be handled manually by the user by printing a custom error message as defined by the user, or it can be handled with the default exception handler. The following examples better illustrate this.
Example 1 (Manual AssertionError Exception Handling)
** Code:
#Handling it manually
try:
x = 1
y = 0
assert y != 0, "Invalid Operation"
print(x / y)
#the errror_message provided by the user gets printed
except AssertionError as msg:
print(msg)
** Output:
Invalid Operation
Example 2 (Default AssertionError Exception Handling)
** Code:
#Roots of a quadratic equation
import math
def quad(a, b, c):
try:
assert a != 0, "Not a quadratic equation as coefficient of x ^ 2 can't be 0"
D = (b * b - 4 * a*c)
assert D>= 0, "Roots are imaginary"
r1 = (-b + math.sqrt(D))/(2 * a)
r2 = (-b - math.sqrt(D))/(2 * a)
print("Roots of the quadratic equation are :", r1, "", r2)
except AssertionError as msg:
print(msg)
quad(-1, 5, -6)
quad(1, 1, 6)
quad(2, 12, 18)
** Output:
Roots of the quadratic equation are : 2.0 3.0
Roots are imaginary
Roots of the quadratic equation are : -3.0 -3.0
- AttributeError:
An AttributeError occurs when an attribute reference or assignment fails. In other words, an AttributeError can be raised for a user-defined class when the user tries to make an invalid attribute reference.
For example, if while working with integers we were to use methods related with strings, the program while raise an AttributeError as integers do not support methods related with strings.
As Python is case-sensitive, spelling variations in methods or variables will raise an AttributeError.
Example 1 (Default AttributeError Exception Handling)
** Code:
#Handling it manually
try:
x = 1
y = 0
assert y != 0, "Invalid Operation"
print(x / y)
except AssertionError as msg:
print(msg)
** Output:
Invalid Operation
- EOFError:
An EOF (short for End of File) Error occurs in Python when the interpreter has reached the end of the program before executing all the code. It is usually raised as a result of failing to declare statements for loops, or failing to close parenthesis or curly brackets whilst writing blocks of code.
Example 1 (Default EOFError Exception Handling)
** Code:
try:
n = int(input())
print(n * 10)
except EOFError as e:
print(e)
** Output:
EOF when reading a line
- FloatingPointError:
Although wrongly considered a “bug”, the FloatingPoint Error occurs when the internal representation of floating-point numbers, which uses a fixed number of binary digits (typically 53 bits) to represent a decimal number, cannot represent decimal numbers in binary exceeding this limit; thus leading to small round-off errors.
It is a common programming issue which arises when dealing with math problems which require exact precision. It also occurs when using such numbers inside conditional statements.
Example 1 (Manual FloatingPointError Exception Handling)
** Code:
try:
a = 10
b = 3
c = a / b
print(c)
except FloatingPointError:
print("Floating point error has occurred")
** Output:
Floating point error has occurred
- ImportError:
An ImportError is raised when a module or member of a module cannot be imported or does not exist.
Example 1 (Manual ImportError Exception Handling)
** Code:
try:
import nibabel
except ImportError:
print(“Such module does not exist!”)
** Output:
Such module does not exist!
- IndexError:
An IndexError can occur when working with any object that is “indexable” (such as strings, tuples, and lists) and occurs when trying to access an element from such an object that is not present in the object.
For example if we have a list of 10 elements, the index is in the range 0 to 9. Trying to access an element with index 10 or more will therefore cause the program to raise an IndexError with the message “IndexError: list index out of range”.
For example:
Example 1 (Manual IndexError Exception Handling)
** Code:
try:
a = [‘a’, ‘b’, ‘c’]
print(a[4])
except IndexError:
print(“Index out of range!”)
else:
print(“Success! No error raised!”)
** Output:
Index out of range!
- KeyError:
In Python, a KeyError exception is raised when trying to access a key that is not in a dictionary.
Example 1 (Manual KeyError Exception Handling)
** Code:
try:
a = [1:‘a’, 2:‘b’, 3:‘c’]
print(a[4])
except KeyError:
print(“Key Error raised!”)
else:
print(“Success! No error raised!”)
** Output:
Key Error raised!
- KeyboardInterrupt:
A KeyboardInterrupt is generated when the user/programmer interrupts the normal execution of a program by pressing the “Ctrl + C” or “Del” keys accidentally or intentionally.
Example 1 (Manual KeyboardInterrupt Exception Handling)
** Code:
try:
inp = input()
print(‘Press Ctrl + C or Interrupt the Kernel:’)
except KeyboardInterrupt:
print(‘Caught KeyboardInterrupt’)
else:
print(‘No exception occurred’)
** Output:
Caught KeyboardInterrupt
- NameError:
A NameError occurs using an undefined variable, function, or module.
Example 1 (Manual NameError Exception Handling)
** Code:
#The try block will generate a NameError, because x is not defined:
try:
print(x)
except NameError:
print("Variable x is not defined")
else:
print("Something else went wrong")
** Output:
Variable x is not defined
- OSError:
While working with the OS module, an OSError is raised when an OS (Operating System) specific system function returns a system-related error, including I/O (Input/Output) failures such as “file not found” or “disk full”.
Example 1 (Manual OSError Exception Handling)
** Code:
# importing os module
import os
r, w = os.pipe()
try :
print(os.ttyname(r))
except OSError as error :
print("File descriptor is not associated with any terminal device")
** Output:
File descriptor is not associated with any terminal device
- OverflowError:
The OverflowError in Python indicates that an arithmetic operation has exceeded the limits of the Python runtime at that particular instance.
Example 1 (Manual OverflowError Exception Handling)
** Code:
try:
import math
print(math.exp(1000))
except OverflowError:
print(“OverFlow Exception Raised.”)
else:
print(“Success, no error!”)
** Output:
OverFlow Exception Raised.
- RuntimeError:
A RuntimeError is raised after a program has been successfully compiled. It is produced by the runtime system if something goes wrong while the program is running. Most runtime error messages include information about where the error occurred and what functions were being executed.
Runtime Errors are usually discovered during the debugging process before the software is released. In cases where the program has been distributed to the public, developers often release patches, or small updates designed to fix the errors.
The following are a variety of common runtime errors: Logical Errors, Input/Output Errors, Undefined Object Errors, Division By Zero Errors, etc.
Example 1 (Manual RuntimeError Exception Handling)
** Code:
# Python program to handle simple runtime error
#Python 3
a = [1, 2, 3]
try:
print ("Second element = %d" %(a[1]))
# Throws error since there are only 3 elements in array
print ("Fourth element = %d" %(a[3]))
except:
print ("An error occurred")
** Output:
Second element = 2
An error occurred
- SyntaxError:
Syntax errors are raised as a result of mistakes encountered while using Python, usually as a result of incorrect spellings. Syntax errors can also be raised as a result of omitting keywords, omitting symbols, as well as the presence of an empty block of code.
Example 1 (Manual SyntaxError Exception Handling)
** Code:
try:
a = 8
b = 10
c = ab
except SytaxError:
print(“Wrong syntax!”)
** Output:
Wrong syntax!
- IndentationError:
IndentationError occurs when spaces or tabs are not properly placed. It is usually raised as a result of poor indentation practices observed whilst working with statements, user-defined functions or classes.
Example 1 (Manual IndentationError Exception Handling)
** Code:
try:
if(a<3):
print("gfg")
except IndentationError:
print(“An indentation was expected!”
** Output:
An indentation was expected!
- TabError:
The TabError is raised as a result of inconsistent use of tabs and spaces while indenting code in the same block.
Example 1 (Manual TabError Exception Handling)
** Code:
numbers = [3.50, 4.90, 6.60, 3.40]
def calculate_total(purchases):
try:
total = sum(numbers)
return total
except TabError:
print(“Inconsistent use of tab spaces”)
total_numbers = calculate_total(numbers)
print(total_numbers)
** Output:
Inconsistent use of tab spaces
- TypeError:
A TypeError is raised when an operation is performed on an incorrect/unsupported object type. In other words, it as an exception that is raised when using inappropriate data type objects in an operation. For example, summing a string and an integer value will raise a TypeError as they are incompatible.
Example 1 (Manual TypeError Exception Handling)
** Code:
try:
geek = "Geeks"
num = 4
print(geek + num + geek)
except TypeError:
print(“Must be strings”)
** Output:
Must be strings
- UnicodeEncodeError:
The UnicodeEncodeError occurs while encoding a unicode string due to the presence of a non-presented character thereby causing the coding-specific encode() to fail.
Example 1 (Manual UnicodeEncodeError Exception Handling)
** Code:
try:
str(u'éducba')
except UnicodeEncodeError:
print(“Error encoding”)
** Output:
Error encoding
- ValueError:
A ValueError, which usually occurs in mathematical operations, is raised when a function receives a wrong value for a valid argument. For example, entering a float input in a function programmed to receive an integer input will raise a ValueError.
Example 1 (Manual ValueError Exception Handling)
** Code:
def add_numbers(num1, num2):
result = 0
try:
result = int(num1) + int(num2)
except ValueError:
print('Must be an integer!')
else:
return result
add_numbers(10, 20)
add_numbers(1, a)
** Output:
30
Must be an integer!
- ZeroDivisionError:
ZeroDivisionError is a built-in Python exception raised whenever a number is divided by 0.
Example 1 (Manual ZeroDivisionError Exception Handling)
** Code:
def divide(x, y):
try:
# Floor Division : Gives only Fractional
# Part as Answer
result = x // y
except ZeroDivisionError:
print("Sorry! You are dividing by zero ")
else:
print("Your answer is: ", result)
#Look at parameters and note the working of the Program
divide(3, 2)
divide(3, 0)
** Output:
Your answer is: 1
Sorry! You are dividing by zero
Else Statement Examples
In the previous examples we saw how different types of exceptions were caught. However, achieving an error-free program is the end goal of any programmer. As such, the following example gives a brief description of what occurs when the program runs error-free.
Example 1 (Successful 'try' execution)
** Code:
#No exception will be raised so the code will be executed without interruption
try:
print("Hello")
except:
print("Something went wrong")
else:
print("Nothing went wrong")
** Output:
Hello
Example 2 (Successful 'else" execution)
** Code:
#No exception will be raised so the code will be executed without interruption
try:
x = 2
if(x>2):
print("Hello")
except:
print("Something went wrong")
else:
print("Nothing went wrong")
** Output:
Nothing went wrong
Comparing both examples it can be seen that the outputs vary. This is as a result of the conditions being met in both cases. In the first example the condition for the try statement is satisfied as such it is executed. However, in Example 2 it can be seen that the condition (x>2) is not satisfied as such the "else" block of code is executed. It is worthy to note that this change in flow control is only possible in the absence of errors (exceptions).
Key Notes
- The "try" block is used to test a block of code for errors.
- The "except" block is used to handle the error.
- The "else" block is used to execute code in the absence of error.
Questions
- What is catching?
- What statement is used in handling exceptions?
- How many types of exceptions are there?