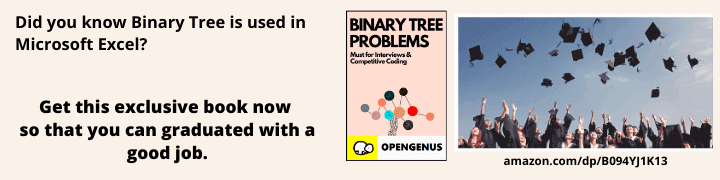
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, I have explained about Typecasting in the C language. The definition, categories of Typecasting, Why it is used and How, along with many examples so that it will be easy to understand.
Typecasting simply refers to changing the data type of one variable to another .
For example, changing an int variable to a double, char to int etc. But before we talk about typecasting, we need to first properly understand the concept of data types and their different properties.
We know that in any programming language, there are some basic data types like int, double, float, char etc. These different data types must be used while declaring any variable, and we can say that the properties of those data type are then transferred to the variable.
For example, each data type requires different amounts of memory to store it. Data types that store numbers also have different range of values that can be stored.
Some of the common data types in C are:
- char - This is used to store a single character like 'a', 'b', '%', '&' etc.
- int - It is used to store an integer value like 128, 5, -45 etc.
- float - It is used to store a floating point value i.e decimals. It has 6 digits after the decimal point.
For eg: 13.240000, 3.200000, 6.000000 etc.
These data types have different memory requirements and can store different range of values as specified below:
Data type | Memory (in bytes) | Range |
---|---|---|
short | 2 | -32,768 to 32,767 |
int | 4 | -2,147,483,648 to 2,147,483,647 |
long | 8 | -2,147,483,648 to 2,147,483,647 |
char | 1 | -128 to 127 |
float | 4 | |
double | 8 |
In C, during the declaration of any variable, it must be declared along with a data type to indicate what type of a variable it has to be. Should it be an integer, a floating point, or a character?
int a = 10;
char v ='8';
float s = 13.456;
char variables should be initialized with single quotation marks. Note that 8 is written within quotation marks here. Hence it's not an integer but a single character.
Now that we've looked into some basic data types, we can move forward to the concept of Typecasting. As we've seen, typecasting, or type conversion, simply means changing the data type of a variable. Sometimes, the compiler automatically performs type conversion without any instructions from the user. Whereas at other times, the programmer gives instructions for typecasting.
Hence, we can classify Typecasting into two categories:
1) Implicit Typecasting :
This is when the compiler automatically or implicity converts the data type of a variable. Thus, it is also known as automatic type conversion.
Implicit conversions generally occur when there is more than one data type in an expression.They can be performed in only one direction as given in the image.
According to this image, a data type can be implicitly converted to any other data type mentioned above it.
For example, int can be implicitly converted to a float, and char can be converted to int.
Let us look at these examples with a sample code:
int a = 10;
char b = 'b';
a = a + b;
printf("a is %d\n",a);
float c = a + 20.5;
printf("c is %f\n",c);
Can you guess what the output would be? Do you think it will give an error?
Let us walk through the code-
10 is stored into the int variable a, and 'b' is stored into the variable b as a character. When we perform (a + b) , b is implicitly converted into int. When a char is converted into an int, it's ASCII value is taken into consideration.
All the characters have ASCII values that range from 0 to 127. Each number within this range represents a different character.
The ASCII value of 'b' is 98.
Therefore, (a+b) would give us 10 + 98 i.e 108.
Similarly, when we add a and 20.5 and store it in c, a gets implicitly converted to float and added with 20.5
Hence, the output of the code will be:
a is 108
b is 128.500000
Implicit conversions also take place when we use a format specifier of a different data type rather than the one we declared the variable with.
Format specifier is used during input and output. %d, %c, %f are some examples of format specifiers.
char a ='A';
printf("a is %d ",a);
In the above block of code, a char variable is printed using %d format specifier. %d is used for int variables. Hence, while printing, the char is implicitly converted to int, and we'll get the ASCII value of the char as output.
a is 65
2) Explicit Typecasting
There may be some situations in which we, as programmers, may have to perform some type conversions. This is known as explicit typecasting.
For this, we need to follow the syntax-
variable = (type)expression
Here, the value of the expression will get converted to the data type specified in the brackets.
For example, consider the following block of code:
float a = 1.734;
int b = (int)a + 2;
printf("b is %d\n",b);
Output:
b is 3
Here, in the second line, we're explicitly typecasting a from float to int. When we typecast any value from float or double to int, all the digits after the decimal points are truncated. Thus, 1.734 gets converted to 1, which is then added with 2, giving us the output as 3.
The most common example where explicit typecasting is used is during the division of 2 integers. Let's take a look -
Consider the following code:
int a = 4, b = 2, c= 5;
float d, e;
d = a/b;
e = c/b;
printf("d is %f\n",d);
printf("e is %f\n",e);
We would expect the output to be 2 and 2.5 respectively.
However, the output comes out to be:
d is 2.000000
e is 2.000000
How can the value of 4/2 and 5/2 both be 2?
This is because 5 and 2 are both int variables. Thus, the result after the division will also be in int format, such that any digits after decimal point are truncated.
So, 2.5 is converted to 2.
But what if we want the real division of two numbers along with the decimal part? This is where explicit typecasting comes into picture.
In such cases, we need to typecast the expression -
e = (float)c/b
Try it out and check whether it works.
int a = 4, b = 2, c= 5;
float d, e;
d = a/b;
e = (float)c/b;
printf("d is %f\n",d);
printf("e is %f\n",e);
Output:
d is 2.000000
e is 2.500000
As you can see, now we get the output in the proper decimal form.
Thus, Typecasting is substantially used in many such cases where the programmer would like to change the data type of any variable or expression.
The compiler also implicitly converts data types, without us even knowing it, but it proves to be convenient for us!