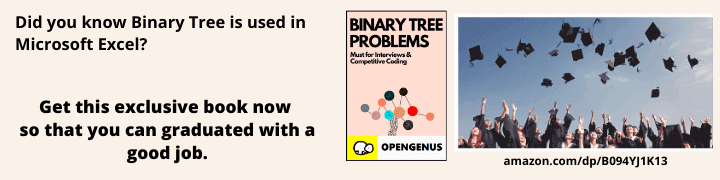
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 30 minutes | Coding time: 10 minutes
In this article, we will learn how to upload any file from the client side to a folder in the server by buidling a Node.js app. To upload a file in Nodejs we shall be using 3 modules i.e http,fs and formidable modules.
- http : for server activities i.e transfer data over the Hyper Text Transfer Protocol (HTTP).
- fs : to save the uploaded file to a location at server.
- formidable : to parse html form data.
If the above modules are not installed already, we should install it using NPM(Node package Manager).NPM is a package manager for Node.js packages, or modules if we like.
Create a folder let's say FileUpload where we will saving all our files and installing our modules.
Run the following commands, in Terminal, to install the respective modules :
npm install http
npm install fs
npm install formidable
The following commands will do the job for you installing the modules we require for uploading file to server.
So,let's start by creating a HTML form, let's name it Upload_File.html,which will include input tags for fill upload.
<form action="fileupload" method="post" enctype="multipart/form-data">
<input type="file" name="filetoupload">
<input type="submit" value="Upload">
</form>
our form will look something like this :
<!DOCTYPE html>
<html>
<head>
<title>Upload File</title>
<style>
body{text-align:center;}
form{display:block;border:1px solid black;padding:20px;}
</style>
</head>
<body>
<h1>Upload files to Node.js Server</h1>
<form action="fileupload" method="post" enctype="multipart/form-data">
<input type="file" name="filetoupload">
<input type="submit" value="Upload">
</form>
</body>
</html>
Now we will be creating a HTTP Server that listens at port 8086 (which can be changed anytime ), let's name it nodejs-upload-file.js .
http.createServer(function (req, res) {
if (req.url == '/uploadform') {
// if request URL contains '/uploadform'
// fill the response with the HTML file containing upload form
} else if (req.url == '/fileupload') {
// if request URL contains '/fileupload'
// using formiddable module,
// read the form data (which includes uploaded file)
// and save the file to a location.
}
}).listen(8086);
We will be saving the file to our Directory we created at starting i.e FileUpload using formidable module.Once file is uploaded, we may respond with "File upload is successful".
Intially files are saved to a temporary location which can be easily changed using fs.rename() method, with the new path we want.
var form = new formidable.IncomingForm();
form.parse(req, function (err, fields, files) {
// oldpath : temporary folder to which file is saved to
var oldpath = files.filetoupload.path;
var newpath = upload_path + files.filetoupload.name;
// copy the file to a new location
fs.rename(oldpath, newpath, function (err) {
if (err) throw err;
// you may respond with another html page
res.write('File uploaded and moved!');
res.end();
});
});
Our File will look something like this :
var http = require('http');
var fs = require('fs');
var formidable = require('formidable');
// html file containing upload form
var upload_html = fs.readFileSync("upload_file.html");
// replace this with the location to save uploaded files
var upload_path = "E:\FileUpload";
http.createServer(function (req, res) {
if (req.url == '/uploadform') {
res.writeHead(200);
res.write(upload_html);
return res.end();
} else if (req.url == '/fileupload') {
var form = new formidable.IncomingForm();
form.parse(req, function (err, fields, files) {
// oldpath : temporary folder to which file is saved to
var oldpath = files.filetoupload.path;
var newpath = upload_path + files.filetoupload.name;
// copy the file to a new location
fs.rename(oldpath, newpath, function (err) {
if (err) throw err;
// you may respond with another html page
res.write('File uploaded and moved!');
res.end();
});
});
}
}).listen(8086);
Run the script from your directory using cmd :
node nodejs-upload-file.js
Open your Browser and hit the url -
http://localhost:8086/uploadform
As soon as you click the url you will find your html page at the following URL.
Click on browse and select the File you'd like to upload and Click Upload. We will be routed to /upload url where we can see our message "File upload is successful".
We can see the File we uploaded in our Directory FileUpload.
congrats🎉 we just learnt to use formidable, fs and http modules for uploading a file to Node.js Server.🎉