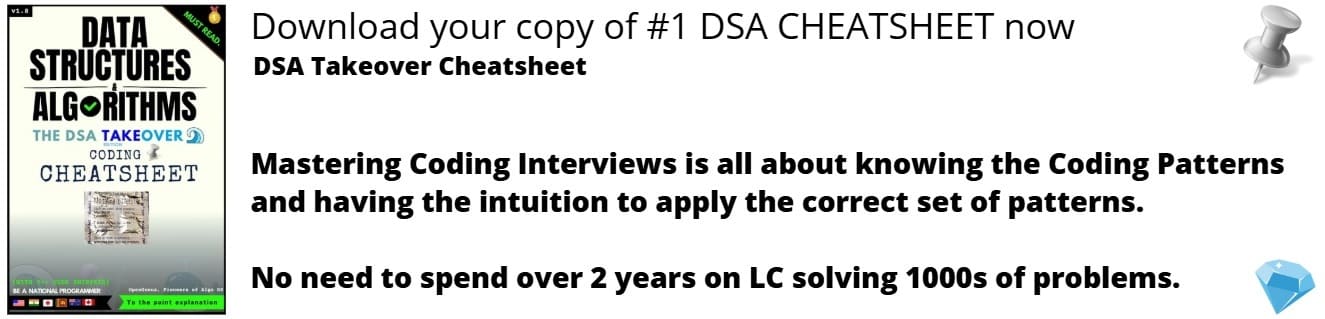
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 20 minutes | Coding time: 4 minutes
A variable is a designated location in memory that is used to store data. Variable has name and a memory location.If we declare a variable in C, it means that we are asking the operating system to reserve a memory partition with that variable name.
In this article, we will take a look into variables of different data types in C, how to get memory address and pointer to a variable and others.
Syntax
data_type variable_name = value;
Examples:
int b = 3;
char x = 'X';
float f = 3.24;
Rules for declaring a variable name
- Variable name can be a collection of uppercase letters, lowercase letters digits and underscores.
- Commas & blanks are not allowed.
- The first character of the name should be uppercase letter or underscore.
- No special symbols other than underscore are allowed.
Types of variables
Basic types of variables:
- Char
- int
- float
- Double
- void
char for Character
Typically stores a single character.
#include<stdio.h>
void main()
{
char x = 'X';
printf("%c",x);
}
int for Integers
Used to store integer values.
#include<stdio.h>
void main()
{
int a = 10;
printf("%d",a);
}
Output:
10
float for Floating point precision numbers
Used to store decimal values with single precision.
#include<stdio.h>
void main()
{
float a = 1.2;
printf("%f",a);
}
Output
1.200000
double for double precision numbers
Used to store decimal value with double precision.
#include<stdio.h>
void main()
{
double a = 3.12;
printf("%f",a);
}
Output
3.120000
Based on the scope there are two types of variable in C programme
- Local variable
- Global variable
What are Local Variables?
- Local variable exit only within the function.
- Outside functions cannot access this variable.
Example:
#include<stdio.h>
void main()
{
int a, b; //local variable declaration
a = 2; //initialization
b = 5; //initialization
printf("%d", a + b);
}
Output
7
What are Global Variables?
- Global variables exit throughout the programme.
- These variables can be access from anywhere in the programme.
- The variable is defined outside the main function.
Example:
#include<stdio.h>
int a = 5; //global variable declaration
void main()
{
int b = 2;
printf("%d", a + b);
}
Output
7
How to find Size of a variable in C?
sizeof operator is used to know the amount of memory allocated to a variable.
#include<stdio.h>
void main()
{
int a = 10;
printf("%d",sizeof(a));
}
output
4
Pointers to variables in C
- Pointers are variables which is used to store the address of another variable.
- Pointers can be belong to any data type such as int, float, char etc.
- Pointer allocate memory dynamically.
syntax
data_type *var_name
Example:
int *a;
char *c;
#include<stdio.h>
void main()
{
int *a, b;
b = 10;
a = &b; // address of varible b is assigned to pointer a
printf("%d",*a);
}
Output
10
How to printing memory address of variable in C?
There are two ways to obtain the address of a variable:
- Using "address of"(&) operator
- Using pointer variable
"address of" (&) operator
#include<stdio.h>
void main()
{
int a;
int *value = &a;
printf("Address of a is %p",*value);
}
output
Address of a is 0x7fff
pointer variable
Pointer variable will return the value of another variable.
#include<stdio.h>
void main()
{
float a;
float *value = &a;
printf("Address of a is: %p",value);
}
Output:
Address of a is: 0x7fff23c35e04