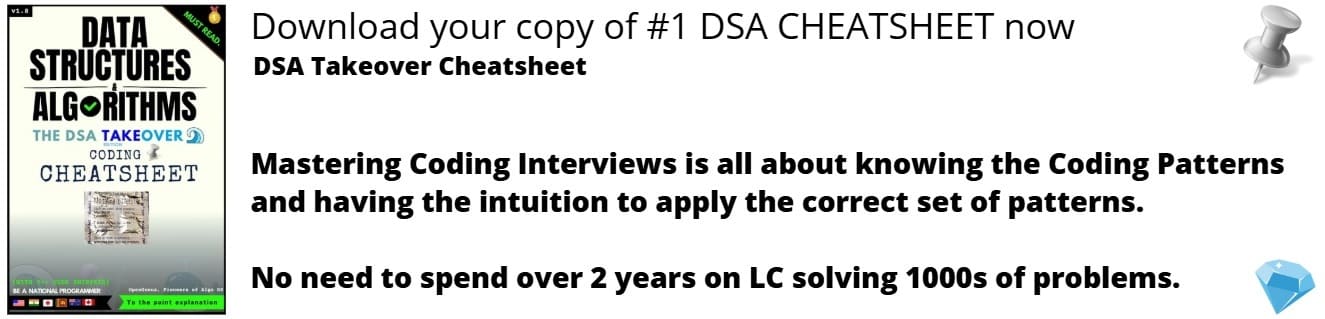
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 20 minutes | Coding time: 5 minutes
In this article we will discuss about Abstract base classes in Python. Abstract classes are an implementation of Abstraction in Object Oriented approach.
Introduction
An abstract class is one that is not used to create objects i.e. it cannot be instantiated. An abstract class is designed only to act as a base class (to be inherited by other classes). It is a design concept in program development and provides a base class upon which other classes may be built.
It enforces abstraction which refers to the act of representing essential features without including the background details or explanations.
NOTE- A class that is derived from an abstract class cannot be instantiated unless all of its abstract methods are overridden.
Example
The following can be implemented using the given pseudocode
Pseudocode
#defining and abstract class with empty implementation
class vehicle:
def milaege():
pass
#sub-classes inheriting the abstract class vehicle
class twoWheeler(vehicle):
def milaege(self):
print("Milaege>20kmpl");
class fourWheeler(vehicle):
def milaege(self):
print("Milaege>8Kmpl");
#checking if classes are sub-class of vehicle class or not
issubclass(twoWheeler,vehicle);
issubclass(fourWheeler,vehicle);
Output
Milaege>20kmpl
Milaege>8kmpl
True
True
The above code is a simple representation for implementing abstract classes. But, if we initialize the object of class vehicle in the same code then the class will become a concrete(normal) class instead of abstract base class. This is due to the property that an abstract class cannot be instantiated.
Explicit implementation
Explicit implementation is necessary to ensure that,
- abstract class cannot be instantiated.
- abstract class does not have any implementation and if any, it must be overridden by its subclases.
Python contains ABC (package: AbstractBaseClass) present within python standard library abc which provides explicit implementation of abstract base classes.
It can be imported using :
from abc import ABC
Python also uses decorater @abstractmethod in order to enforce abstraction. It can be imported using following command:
import abstractmethod
Implementation
from abc import ABC, abstractmethod
#creating abstract class using abc package
class vehicle(ABC):
def __init__(self, value):
self.value = value
super().__init__()
#wraping up abstract method using decorator
@abstractmethod
def milaege:
print("Better milaege is expected...")
#sub-classes inheriting the abstract class vehicle
class twoWheeler(vehicle):
def milaege(self):
print("Milaege>20kmpl");
class fourWheeler(vehicle):
def milaege(self):
print("Milaege>8Kmpl");
def main():
inst1.twoWheeler() #instance 1 created
inst1.milaege()
inst2.fourWheeler() #instance 2 created
inst2.milaege()
if __name__=="__main__":
main()
Output
Milaege>20kmpl
Milaege>8kmpl
The above code represents the explicit implementation of abstract class in python.
Error
Now if we try to create an instance of base class vehicle in main :
inst3.vehicle() #Creating an instance of abstract class vehicle
inst3.milaege()
The above code will result into an error as we cannot create an instance of abstract class vehicle.
Applications
We use abstract class whenever we need a basic attribute or method common to all other classes without having to specifying it again and again. Thus an abstract class provides :
1.Basic functionality
2.Code reusability
3.compact and faster coding.
Questions
- Difference between Interfaces and abstract classes in python.
Python supports multiple inheritance and thus we can implement abstract classes. But python does not have a keyword interface as in java or C# and thus not provide any kind of interface implementation.
- Is it necessary for abstract class to have abstract method?
No, an abstract class may not have an abstract method. It is although suggested that an abstract class may contain an abstract method.
- Can we create an instance of abstract class?
We cannot create an instance of an abstract class. If there is any instance of a base class then it is treated as a normal class.
- Can an abstract class contain constructors?
Yes, an abstract class can contain constructors just like any other normal class.