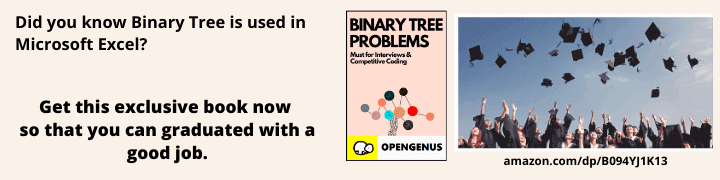
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explored Array in JavaScript in depth along with multi-dimensional array and different array methods like splice(), push() and more.
Table of Content
1. Introduction
2. Array Structure
2.1. Literal Representation
2.2. Array() constructor
3. Array Types
3.1. One-dimensional
3.2. Multidimensional
4. Methods
4.1. Push()
4.2. Unshift()
4.3. Splice()
4.4. Pop()
4.5. Length
4.6. forEach()
4.7. From()
4.8. isArray()
4.9. Array.of()
4.10. Map()
4.11. Filter()
5. Final Considerations
Reference
1. Introduction
An array is a structure that allows the storage of variables, objects, and functions.
In an array, elements of different types can be stored at the same time.
Access to the array can be done in several ways.
In this article, I'll describe some ways to create and manipulate arrays.
2. Array Structure
The array is represented by different types of syntax.
2.1. Literal Representation
The array is represented by square bracket symbols, which may or may not contain any value.
In Figure 1, there is an example of an empty array (with no value).
var arr = []
Figure 1. The literal representation of an empty array.
The array of the following example (Figure 2), on the other hand, contains 3 values, JavaScript, Java, Kotlin.
var arr = [JavaScript, Java, Kotlin]
Figure 2. The literal representation contains values.
2.2. Array() constructor
Another syntax for declaring the array is using the **new** constructor. In Figure 3, the constructor was used to create an array with 4 numbers, returning the value contained in position two, which would be *[2]*.
var num = new Array(0, 1, 2, 3);
console.log(num[2]);
Figure 3. Array developed with the new constructor.
The constructor array can also be of the empty type (contains no value) (Figure 4).
var num = new Array();
Figure 4.Array developed with the constructor without containing a value.
Or, the array can specify its size and thus allocate memory.
In Figure 5, the number of elements specified was 5.
var arr = new Array(5)
Figure 5. Memory allocation using the new constructor.
The recommendation is to use the literal array when possible, as it is the most practical way, with greater readability and speed of implementation.
3. Array Types
There are three types of array:
3.1. One-dimensional
This type of array stores variables of any type except another array.
In Figure 6, the one-dimensional array returned: [Quartz, Natrolite, Serpentine].
var arr = ["Quartz", "Natrolite", "Serpentine"];
console.log(arr);
Figure 6. One-dimensional array.
3.2. Multidimensional
The multidimensional array is that array that stores other arrays as an element.
In this section, we deal with the array of the bidimensional type.
In Figure 7, the *arrB array stores two other arrays. The code returns [['JavaScript', 'C', 3, 'C++'], ['Java', 'Kotlin', 2, 'Python']].
var arrB = [];
arrB[0] = ["JavaScript", "C", 3, "C++"];
arrB[1] = ["Java", "Kotlin", 2, "Python"];
console.log(arrB);
Figure 7. Two-dimensional array.
4. Methods
For manipulating arrays, there are several types of commands. In this section, we will cover some types. For more information, visit the MDN and w3schools websites.
4.1. Push()
The push() method adds elements into the array.
To illustrate (Figure 8), the string "OpenGenus" was added to the list. The end result ['GitHub', 'LinkedIn', 'OpenGenus']
const list = ["GitHub", "LinkedIn"];
list.push("OpenGenus");
console.log(list)
Figure 8. Adding an element to the end of the array with push().
4.2. Unshift()
In unshift(), the string is added to the beginning of the list.
In Figure 9, the string "OpenGenus" has been added to the beginning of the list, returning ['OpenGenus', 'GitHub', 'LinkedIn'].
const list = ["GitHub", "LinkedIn"];
list.unshift("OpenGenus");
console.log(list)
Figure 9.Adding an element at the beginning of the array with unshift().
4.3. Splice()
The splice() method allows you to remove or even overwrite elements within the array.
This case is observed in Figure 10, in which the zero position element was eliminated by adding 3 elements ('Blue', 'Red', 'Yellow') to the list. The final result: ['Blue', 'Red', 'Yellow', 'Orange', 'Black'].
It should be noted that the position where the exchange was performed (zero) and the number of elements that be eliminated (one) were indicated.
var colors = ["White", "Orange", "Black"];
colors.splice(0, 1,"Blue", "Red", "Yellow");
console.log(colors)
Figure 10. Eliminating string elements with splice().
4.4. Pop()
The pop() method deletes the last element of the array and returns that removed element.
To exemplify, the number 6 was removed from the list, which then returned with the pop() command (Figure 11).
var num = [0, 1, 2, 3, 4, 5, 6]
var removed = num.pop();
console.log(removed);
Figure 11. Removal and return of the element with the pop() method.
4.5. Length
The length method lets you know the number of elements contained in the array.[8]
In Figure 12, length controls the timing of the loop break that occurs with for.
var colors = ["Blue","Red", "Yellow"];
for (i = 0; i < colors.length; i++){
console.log("The color " + i + " is " + colors[i] );
}
Figure 12. Using length with for.
The result (Figure 13) obtained by executing the code (Figure 12):
The color 0 is Blue
The color 1 is Red
The color 2 is Yellow
Figure 13. Result generated in the implementation of the code in Figure 12.
4.6. forEach()
The forEach() method iterates through the elements of the array and executes a callback function for each item.
The callback is a type of function that is only executed after another function has been processed.
In the example (Figure 14), we used forEach() with the prototype property. This property allows the creation of properties with characteristics of the function.
In this example, we created a prototype (par()) to return the pairs' numbers in a new array.
An empty array was also created with forEach() to read the variable and add elements that meet the specification through the push() method. The result generated was [12, 34, 46, 68, 100].
Array.prototype.par = function() {
var pairNum = [];
this.forEach(num => {
if (num % 2 === 0 )
pairNum.push(num);
})
return pairNum;
};
var arrNum = [11, 12, 33, 34, 45, 46, 67, 68, 89, 100];
var arrPar = arrNum.par();
console.log(arrPar);
Figure 14. Code example with forEach method and prototype property.
4.7. From()
The from's method is used when you want to create an array from an object that is not an array.
In Figure 15, the letters of the word Java were transformed into an array. Returning as a result ['j', 'a', 'v', 'a'].
var test = "Java"
var arr = Array.from(test);
console.log(arr);
Figure 15. Example with from() method.
4.8. isArray()
The isArray() method is used when you want to find out if a variable or constant is an array object.
If the result is positive, the code returns "true", otherwise "false".
In Figure 16, the string list returned false because the content does not set an array object.
var str = "Python, Java, SQL, Linux";
console.log(Array.isArray(str));
Figure 15. Example with isArray() method.
4.9. Array.of()
The Array.of() method returns a new array with the elements contained within parentheses.
In Figure 16, the Array.of method returns [3, 2, 1, 0] as a result.
var arr = Array.of(3, 2, 1, 0,);
console.log(arr);
Figure 16. Example with Array.of() method.
4.10. Map()
The map() method performs a callback function for each item and returns processing to a new array.
In example 17, the list elements are capitalized with the toUpperCase() command and the map method returns a new array, resulting in ['PINK', 'RED', 'SALMON', 'ROSE'].
var colors = [ 'pink', 'red', 'salmon', 'rose'];
var upper = colors.map(nome => nome.toUpperCase());
console.log(upper);
Figure 17. Creating a new array with the map() method.
4.11. Filter()
The filter() method, as the name implies, filters the information provided, returning values that meet the specifications.
In Figure 18, was asked for the numbers that are greater than and equal to 18. The result is the array [19].
var num = [15, 19, 16, 11];
console.log(num.filter(check));
function check(num) {
return num >= 18;
}
Figure 18. Example of using the filter() method in JavaScript.
5. Final Considerations
The JavaScript language is widely used for allowing interaction between the user and the interface.
The array is an important tool for storing data of various types. In this article at OpenGenus, we discussed some methods for manipulating and creating arrays but there are several other methods, which can be consulted.