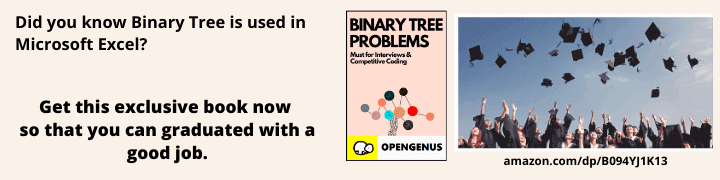
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
We all know that C is a general-purpose and Procedural programming language. And for most of us, It is the entry point or basic to learn other programming languages. In this article we'll discuss a bit about the Standard Header Library "stdio.h". Firstly we'll start by explaining about the structure of C program. Secondly, we discuss up on the Introduction to Header file(as we stick specific to "stdio.h"). Later, we look into specific attributes of Header file like - Built-in functions, Library Variables and Macro.
In short, stdio.h stands for standard input and output and is used to provide utilities supporting input and output features in C Programming Language.
Following are the sections of this article:-
1. Structure of C Program
We'll discuss the Structure of C program with a small example code. Let us consider the following example,
// Structure of C Program
#include <stdio.h> // Header Files Inclusion
int main(void) // Main Method Declaration
{ // Begin of Scope/Body of Function main()
printf("OpenGenus is Awesome!"); // Printing the value to console
// return's value depending upon the return type of the function
return 0;
} // End of Scope/Body of Function main()
/* We can view the output at console as,
OpenGenus is Awesome!
*/
From the above example, Generally Header Files consists many features so that, by including or importing the Header Files("stdio.h"
) we can consume the functionality. Basically these functions are called as "inbuilt" or "built-in" functions in C programming.
The prototype or signature and data definitions of these functions are present in their respective header files. And these Header files may also contain macros and variables specific to the library. In C program, the program execution starts from main()
and its signature is having a return type of integer(from our example). It also consists of body of the function inside which we include our custom logic.
In this article we are discussing in brief up on the "Header Files Inclusion" section for "stdio. h"
.
2. Introduction to "stdio.h"
A header file in C is the one in which it contains function declarations/ definitions, variables and macro definitions to be shared between several source files and has a filename with extension ".h". Header file are of two types,
- Built-In
- User defined
Syntax to include any Header file, irrespective of its type
#include <filename.h>
Here #include
is a preprocessing directive(which informs the C compiler to include those specific files for the program). And <filename.h>
- a Header filename can be different as per the requirement for the specific functionality.
In many programming language, they have their own convention for getting the input from the user or sending the processed output to the user at the console. Similarly in C language we have this header file - "stdio.h".
Lets split the keyword into a small individual, So that we can bring a meaning to it later. The name of header file is "stdio. h"
, i.e -
std
- Standardio
- Input / Output
Hence, Its clear that this header file in C program is used for performing Basic or Standard Input/Output operations.
In other words, when we require Input/Output functionality in our program it can be achieved by including this header file at the beginning of the program.
3. Built-In Functions
As discussed in the previous section (section 1), Header files do have many functions. Built-in C functions in respect of "stdio. h"
file are as follows.
- Formatted Input / Output Functions
- printf(): To print the values onto the output screen.
- scanf(): To read a character, string, numeric data from keyboard.
- sprint(): Writes formatted output to string.
- sscanf(): Reads formatted input from a string.
- fprintf(): To Write formatted data to a file.
- fscanf(): To Read formatted data from a file.
- File Operation Functions
- fopen(): To Open a file.
- fclose(): Closes an opened file.
- remove(): To Delete a file.
- fflush(): To Flush a File Buffer.
- Character Input / Output Functions
- getc(): It reads character from a file.
- gets(): It reads line of string from keyboard.
- getchar(): It reads character from keyboard.
- puts(): It writes line of string to output console.
- putchar(): It writes a character to console.
- putc(): Writes a character to a file.
There are couple of more Functions that are available in this Header file. Above mentioned are the common Built-In Functions for the same.
4. Library Variables
Under this Header we have few specific variables, which we can define them for the ease of programming in our implementing code. A variable that is specific to the library are called as "Library Variables".
- Following are the variables defined in the header "stdio.h",
- size_t: This variable is result of the
sizeof
keyword and data type - unsigned integral type.- FILE: An object type, which is suitable for storing information for a file stream.
- fpos_t: An object type, which is suitable for storing any position in a file.
5. Macros
A macro is a small snippet of code that is given a name and an expression which performs a tiny task.
Syntax to define a macro is as follows,
#define name expression
Here #define
- is a preprocessor directive.
name
- can be any descriptive name.
expression
- can be any small operation, that performs a repetitive calculation and returns result.
- Following are the Macros defined in the header "stdio.h",
- NULL: It contains the value of a null pointer constant.
- BUFSIZ: It is an integer, That represents the size of the buffer used by the setbuf function.
- EOF: It is a negative integer, That represents that the end-of-file has been reached.
- FILENAME_MAX: It is an integer, That represents the longest length of a char array suitable for holding the longest possible filename.
- TMP_MAX: It is the maximum number of unique filenames that the function tmpnam can generate.
Above are the common macros which are used, If we include "stdio.h"
in the implemented program.
6. Conclusion
Finally we are at the end of this article up on the discussion of Basic of "stdio.h". Further more we can think of exploring each and every Built-in function, Library variable and the Macro of the header file("stdio.h"
). Similarly, we have more Header files in C programming language and they serve different functionality, feature and purpose
Hope it was an informative post. Thanks!