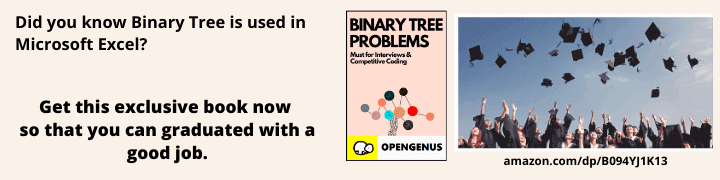
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
A complex number is a number that can be written in the form x+yi where x and y are real numbers and i is an imaginary number.
- Therefore a complex number is a combination of:
- real number.
- imaginary number.
Example:
6+2i //here i=√-1
//6 is real part and 2i is imaginary
Representation of complex numbers in C
In C language, we can represent or can work on complex numbers by using
I) structure, a C feature
II) <complex.h>
library
Representing complex number using structure
In this, we will create a structure of complex number which will hold the real part and imaginary part.
Example:
struct complex
{
int real, imag;
};
here, struct is a keyword for creating a structure and complex is the name of the structure.
real is variable to hold the real part and imag is a variable to hold the imaginary part.
real and imag can be of any data type (int, float, double).
Representing complex numbers using <complex.h> library
<complex.h> library contains many inbuilt functions which helps in creation or calculation of complex numbers.
#include<complex.h>
As we know that the complex numbers are represented as x+yi .
Depending on type of x and y there are three data types in C for complex numbers:
-double complex
-float complex
-long double complex
Example of initialization of complex numbers:
double complex c1=5.0+2.0*I; //I is imaginary part
double complex c2=7.0-5.0*I;
It provides inbuilt exponential functions, power functions, trigonometric functions, and some manipulation function.
**Manipulation functions**
creal() :computes the real part of the funtion.
crealf() //for float
creall() //for long double.
cimag() :computes the imaginary part of the function.
cimagf() //for float
cimagl() //for long double
**Exponential functions**
cexp() :computes the complex base-e exponential
cexpf() //for float
cexpl() //for double
**Trigonometric functions**
csin() :computes the complex sine
csinf() //for float
csinl() //for double
ccos() :computes the complex cosine
ccosf() //for float
ccosl() //for double
ctan() :computes the complex tangent
ctanf() //for float
ctanl() //for double
casin() :computes the complex arc sine
casinf() //for float
casinl() //for double
cacos() :computes the complex arc cosine
cacosf() //for float
cacosl() //for double
catan() :computes the complex arc tangent
catanf() //for float
catanl() //for double
**Power function**
cpow() :computes the complex power function
cpowf() //for float
cpowl() //for double
csqrt() :computes the complex square root
csqrtf() //for float
csqrtl() //for double
Operation on complex numbers
Addition of two complex numbers(using structure)
In this program we will add two complex numbers which will be entered by the user.The user will input the real and imaginary parts of two complex numbers and prints the result.
Here we will use structure to store a complex number.
#include<stdio.h> //standard input output library
struct complex //structure for coplex number
{
double real,imag; //variables holding real and imaginary part of type double
};
int main()
{
struct complex x,y,c;
printf("enter the value of x and y for first complex number: ");
scanf("%lf%lf",&x.real, &x.imag);
printf("enter the value of x and y for second complex number: ");
scanf("%lf%lf",&y.real, &y.imag);
c.real=x.real+y.real; //addition of real part
c.imag=x.imag+y.imag; //addition of imaginary part
printf("Sum of complex numbers: %.2lf%+.2lfi",c.real,c.imag);
return 0;
}
OUTPUT:
enter the value of x and y for first complex number: 4 6
enter the value of x and y for second complex number: 2 3
Sum of complex numbers: 6.00+9.00i
Addition of two complex numbers(using <complex.h> library)
In this program we will add two complex numbers using <complex.h> library.
In this we will compute the real part and imaginary part of the function using creal() and cimag() functions respectively.
#include <stdio.h> //standard input output library
#include <complex.h> //standard complex number library
int main() {
double complex c1=5.0+3.0*I; //declaration of a complex number
double complex c2=7.0-4.0*I;
printf("Addition of complex numbers:\n");
printf("values of complex number c1:c1=%.2lf+%.2lfi\n", creal(c1), cimag(c1)); //computing real and imaginary part of c1
printf("values of complex number c2:c2=%.2lf+%.2lfi\n", creal(c2), cimag(c2)); //computing real and imaginary part of c2
double complex sum =c1+c2;
printf("The sum: c1+c2= %.2lf%+.2lfi\n", creal(sum), cimag(sum));
return 0;
}
OUTPUT:
Addition of complex numbers:
values of complex number c1: c1=5.00+3.00i
values of complex number c2: c2=7.00+-4.00i
The sum: c1+c2=12.00-1.00i
Subtraction of two complex numbers(using structure)
In this program we will subtract two complex numbers which will be entered by the user.The user will input the real and imaginary parts of two complex numbers and prints the result.
Here we will use structure to store a complex number.
#include<stdio.h> //standard input output library
struct complex //structure for coplex number
{
double real,imag; //variables holing real and imaginary part of type double
};
int main()
{
struct complex x,y,c;
printf("enter the value of x and y for first complex number: ");
scanf("%lf%lf",&x.real, &x.imag);
printf("enter the value of x and y for second complex number: ");
scanf("%lf%lf",&y.real, &y.imag);
c.real=x.real-y.real; //subtraction of real part
c.imag=x.imag-y.imag; //subtraction of imaginary part
printf("Subtraction of complex numbers: %.2lf%+.2lfi",c.real,c.imag);
return 0;
}
OUTPUT:
enter the value of x and y for first complex number: 4 6
enter the value of x and y for second complex number: 3 2
Subtraction of complex numbers: 1.00+4.00i
Subtraction of two complex numbers(using <complex.h> library)
In this program we will subtract two complex numbers using <complex.h> library.
In this we will compute the real part and imaginary part of the function using creal() and cimag() functions respectively.
#include <stdio.h> //standard input output library
#include <complex.h> //standard complex number library
int main() {
double complex c1=5.0+3.0*I; //declaration of a complex number
double complex c2=7.0-4.0*I;
printf("Subtraction of complex numbers:\n");
printf("values of complex number c1: c1=%.2lf+%.2lfi\n", creal(c1), cimag(c1)); //computing real and imaginary part of c1
printf("values of complex number c2: c2=%.2lf+%.2lfi\n", creal(c2), cimag(c2)); //computing real and imaginary part of c2
double complex difference = c1-c2;
printf("The difference: c1-c2 = %.2lf %+.2lfi\n", creal(difference), cimag(difference));
return 0;
}
OUTPUT:
Subtraction of complex numbers:
values of complex number c1: c1=5.00+3.00i
values of complex number c2: c2=7.00+-4.00i
The difference: c1-c2=-2.00+7.00i
Multiplication of two complex numbers(using structure)
In this program we will multiply two complex numbers which will be entered by the user.The user will input the real and imaginary parts of two complex numbers and prints the result.
Here we will use structure to store a complex number.
//multiplication formula in complex numbers
c.real=x.real*y.real-x.imag*y.imag;
c.imag=x.imag*y.real+x.real*y.imag;
#include<stdio.h> //standard input output library
struct complex //structure for coplex number
{
int real,imag; //variables holing real and imaginary part of type integer
};
int main()
{
struct complex x,y,c;
printf("enter the value of x and y for first complex number: ");
scanf("%d%d",&x.real, &x.imag);
printf("enter the value of x and y for second complex number: ");
scanf("%d%d",&y.real, &y.imag);
c.real=x.real*y.real-x.imag*y.imag;
c.imag=x.imag*y.real+x.real*y.imag;
printf("Multiplication of complex numbers: %d+%di",c.real,c.imag);
return 0;
}
OUTPUT:
enter the value of x and y for first complex number: 2 4
enter the value of x and y for second complex number: 5 1
Multiplication of complex numbers: 6+22i
Multiplication of two complex numbers(using <complex.h> library)
In this program we will multiply two complex numbers using <complex.h> library.
In this we will compute the real part and imaginary part of the function using creal() and cimag() functions respectively.
#include <stdio.h> //standard input output library
#include <complex.h> //standard complex number library
int main() {
double complex c1=5.0+3.0*I; //declaration of a complex number
double complex c2=7.0-4.0*I;
printf("Multiplication of complex numbers:\n");
printf("values of complex number c1: c1=%.2lf+%.2lfi\n", creal(c1), cimag(c1)); //computing real and imaginary part of c1
printf("values of complex number c2: c2=%.2f+%.2lfi\n", creal(c2), cimag(c2)); //computing real and imaginary part of c2
double complex product =c1*c2;
printf("The product: c1*c2=%.2lf%+.2lfi\n", creal(product), cimag(product));
return 0;
}
OUTPUT:
Multiplication of complex numbers:
values of complex number c1: c1=5.00+3.00i
values of complex number c2: c2=7.00+-4.00i
The product: c1*c2=47.00+1.00i
Now we will perform all the operations in a single code using structure.
#include <stdio.h>
#include <stdlib.h>
struct complex
{
double real, imag; //real and imag of type double
};
int main()
{
int choice, a, b, z;
struct complex x, y, c;
while(1)
{
printf("Enter your choice\n"); //choices for operations
printf("Press 1. Addition.\n");
printf("Press 2. Subtra tion\n");
printf("Press 3. Multiplication\n");
printf("Press 4. Division\n");
printf("Press 5. Exit\n");
scanf("%d", &choice);
if (choice == 5)
exit(0);
if (choice >= 1 && choice <= 4)
{
printf("Enter the value of x and y for first complex number:");
scanf("%lf%lf", &x.real,&x.imag);
printf("Enter the value of x and y for second complex number:");
scanf("%lf%lf", &y.real,&y.imag);
}
if (choice == 1) //addition
{
c.real = x.real + y.real;
c.imag = x.imag + y.imag;
if (c.imag >= 0)
printf("Sum of the complex numbers = %.2lf+%.2lfi", c.real, c.imag);
else
printf("Sum of the complex numbers = %.2lf %.2lfi", c.real, c.imag);
}
else if (choice == 2) //subtraction
{
c.real = x.real - y.real;
c.imag = x.imag - y.imag;
if (c.imag >= 0)
printf("Difference of the complex numbers = %.2lf+%.2lfi", c.real, c.imag);
else
printf("Difference of the complex numbers = %.2lf %.2lfi", c.real, c.imag);
}
else if (choice == 3) //multiplication
{
c.real = x.real*y.real - x.imag*y.imag;
c.imag = x.imag*y.real + x.real*y.imag;
if (c.imag >= 0)
printf("Multiplication of the complex numbers = %.2lf+%.2lfi", c.real, c.imag);
else
printf("Multiplication of the complex numbers = %.2lf %.2lfi", c.real, c.imag);
}
else if (choice == 4) //division
{
if (y.real == 0 && y.imag == 0)
printf("Division by 0 + 0i isn't allowed.");
else
{
a = x.real*y.real + x.imag*y.imag;
b = x.imag*y.real - x.real*y.imag;
z = y.real*y.real + y.imag*y.imag;
if (a%z == 0 && b%z == 0)
{
if (b/z >= 0)
printf("Division of the complex numbers = %.2lf+%.2lfi", a/z, b/z);
else
printf("Division of the complex numbers = %d %di", a/z, b/z);
}
else if (a%z == 0 && b%z != 0)
{
if (b/z >= 0)
printf("Division of two complex numbers = %.2lf+ %.2lf/%.2lfi", a/z, b, z);
else
printf("Division of two complex numbers = %.2lf%.2lf/%.2lfi", a/z, b, z);
}
else if (a%z != 0 && b%z == 0)
{
if (b/z >= 0)
printf("Division of two complex numbers = %.2lf/%.2lf+%.2lfi", a, z, b/z);
else
printf("Division of two complex numbers = %.2lf %.2lf/%.2lfi", a, z, b/z);
}
else
{
if (b/z >= 0)
printf("Division of two complex numbers = %.2lf/%.2lf + %.2lf/%.2lfi",a, z, b, z);
else
printf("Division of two complex numbers = %.2lf/%.2lf %.2lf/%.2lfi", a, z, b, z);
}
}
}
else
printf("Invalid choice.");
printf("\nPress any key to enter choice again...\n");
}
}
OUTPUT:
Enter your choice
Press 1. Addition.
Press 2. Subtra tion
Press 3. Multiplication
Press 4. Division
Press 5. Exit
1
Enter the value of x and y for first complex number:2 5
Enter the value of x and y for second complex number:3 7
Sum of the complex numbers = 5.00+12.00i
Press any key to enter choice again...
Enter your choice
Press 1. Addition.
Press 2. Subtra tion
Press 3. Multiplication
Press 4. Division
Press 5. Exit
4
Enter the value of x and y for first complex number:3 6
Enter the value of x and y for second complex number:2 7
Division of two complex numbers = 48.00/53.00 + -9.00/53.00i
Press any key to enter choice again...
Enter your choice
Press 1. Addition.
Press 2. Subtra tion
Press 3. Multiplication
Press 4. Division
Press 5. Exit
5
Now we will perform all the operations using <complex.h> library
#include <stdio.h> //standard input output library
#include <complex.h> //standard complex number library
int main()
{
double complex c1 = 5.0+3.0*I;
double complex c2 = 7.0-4.0*I;
printf("values of complex number c1: c1 = %.2lf + %.2lfi\n", creal(c1), cimag(c1)); //computing real and imaginary part of c1
printf("values of complex number c2: c2 = %.2lf + %.2lfi\n", creal(c2), cimag(c2)); //computing real and imaginary part of c2
double complex sum = c1+c2; //addition
printf("The sum: c1+c2 = %.2lf %+.2lfi\n", creal(sum), cimag(sum));
double complex difference = c1-c2; //subtraction
printf("The difference: c1-c2 = %.2lf %+.2lfi\n", creal(difference), cimag(difference));
double complex product = c1*c2; //multiplication
printf("The product: c1*c2 = %.2lf %+.2lfi\n", creal(product), cimag(product));
double complex quotient = c1/c2; //division
printf("The quotient: c1/c2 = %.2lf %+.2lfi\n", creal(quotient), cimag(quotient));
return 0;
}
OUTPUT:
values of complex number c1: c1 = 5.00 + 3.00i
values of complex number c2: c2 = 7.00 + -4.00i
The sum: c1+c2 = 12.00 -1.00i
The difference: c1-c2 = -2.00 +7.00i
The product: c1*c2 = 47.00 +1.00i
The quotient: c1/c2 = 0.35 +0.63i