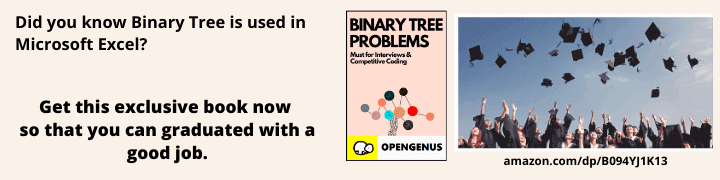
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explained how to delete an array or delete an element in an array in C++. We can covered the case of static and dynamic memory allocated array along with the basics of array.
Table of contents:
- Basic of Array and different operations
- Delete() in C/ C++
- Delete an element in array
- Delete statically allocated array in C++
- Delete dynamically allocated array in C++
- Time complexity to delete an array
Now we going to explore the whole process of deleting an array in C++.
Here are some key points regarding deletion process:-
- Deleting an array is similar to assigning undefined to that particular element .
- Delete the element to be deleted and move the other elements to the left to keep all elements together.
- If array is static memory allocated, memory cannot be deleted. Memory is released when program terminates.
- If array is dynamic memory allocated, extra memory can be deleted by using realloc.
- The time complexity to delete an element is O(N) time.
Let's start with discussing what is array, what are types of array and about the declaration process of array.
Basic of Array and different operations
INTRODUCTION OF ARRAY
An array is a group of relatable data types ,which are invoke by the common names and all being of same types and size. An array is a collection of elements of similar data type. This collection is finite, and the elements are stored at adjacent memory locations.
- The syntax of array declaration is:-
Data type array_name[size_of_array];
Order of representation :-
1. Row major order - The row major order of representation of arrays is an order in which the elements are stored in row wise manner in an array.
2. Column major order - The column major order of representation of arrays is an order in which the elements are stored in column wise manner in an array.
DECLARATION OF ARRAY
Array variables are declared equitably to variables of their data type, except that the variable name is followed by one pair of square [ ] brackets for each dimension of the array. Uninitialized arrays must have the dimensions of their rows and columns listed within the square brackets.
Dimensions used when declaring arrays in C must be positive integral constants or constant expressions.
There are different ways in which we can declare an array. It can be done by specifying its type and size, by initializing it or both.
- Array declaration by specifying size
we can declare an array of user specified sizeint arr1[10]
int arr2[n]
- Array declaration by initializing elements
compiler created an array of size 4int arr[] = {10, 20, 30, 40}
- Array declaration by specifying size and initializing elements
compiler created an array of size 6, initalized first 4 element as spcified by the user and rest element as {0,0}.int arr[6] = {10, 20, 30, 40,}
TYPES OF ARRAY :-
Array can of following types :-
- Static or (1D) array.
- Dynamic or (2D-3D) array.
-
Static array
The static array is the most common form of array used. It is a type of array whose size cannot be altered. static array stores their values on the stack, and their size must be known at compile time.
It has a local scope. Thus it can be used only within a block in which it is declared. If the static array is not exceptionally initialized, it's elements get initialized with the default value which is zero for any arithmetic types(int, float, char) and NULL for pointers. A static array has a lifetime till the execution end of program. Thus, a static array defined within a function is not shattered when control leaves that function and the value of this array is applicable the next time the function is called. -
Dynamic array
A dynamic array is an array with vast improvement: automatic resizing. One impediment of array is that they're fixed size. It means that you need to specify the number of element you want your array will hold ahead of time. A dynamic array expands as we add more elements in it. So we don't need to determine to size ahead of time.
When we designate a dynamic array, dynamic array implementation makes an elemental fixed-sized array. As unused memory is applicable on heap, so whenever memory provision comes at run time it is designated using heap data structure as it is pool of free memory space. In C++, we have operator know as new which return address designated at heap to that variable.
Basic operations:-
Following are the basic operations supported by an array.
-
Traverse
The method of processing each element in the array exactly once is known as traversal, and this whole operation is known as traversing.
In array, traversing operation starts from first element in the array and end at last element of the array.
Algorithm:-
STEP 1. [Initialization] Set l = LB.
STEP 2. Repate step 3 and 4 while l<=UB.
STEP 3. [Processing] process the A[l] element.
STEP 4. [Increment the counter] l=l+1
[End of the loop of step 2]
Here, l is counter variable
UB - Upper Bond, LB - Lower Bond, A[] is linear array. -
Insertion
Insert operation is to insert one or more data element into an array. Based on our reqiurement, a new element can be added in the beginning end, or any given index of array.
Suppose there are N element in an array and we want to insert a new element between first and second element. We have to move last N-1 element down in order to create space for a new element.
Algorithm
STEP 1. [Initialization]Set l = N
STEP 2. [Loop]Repeat step 3 and step 4 while l>=P
STEP 3. [Move lth element downward]Set A[l+1] = A[l]
STEP 4. [Decrement counter]Set l=l-1
[End of loop]
STEP 5. [Insertion element]Set A[P]=X
STEP 6. Set N=N+1
STEP 7. Exit
Here, P is position; N is number of element; A[] is array -
Deletion
This operation is use to delete an element from specific position from one dimensional array.
In order to delete an element from array we have to delete an element from specific position and then shift remaining elements upward to take vacant space of the deleted element.
Algorithm;-
STEP 1. [Initialization]Set X = A[P]
STEP 2. [Loop]Repeat for l = P to N-1
STEP 3. [Moving l+1 th element upward]Set A[P] = X
[End of loop]
STEP 4. [Reset the number N]Set N = N-1
STEP 5. Exit -
Search
Search operation is use to find out the location of the element if it is present in the given collection of data element.
Suppose element to be seached in an array be X. Then we have to start from the beginning and have to compare X with each element. This operation will be continued until the element is found or array is finished.
Algorithm:-
STEP 1.
SREP 2.
S -
Update
Update oeration refers to updating an exsiting element from the array at the given index.
Basically update operation is use when we want want to update any element in an array. Suppose we want to update the exsiting element in the list of array, then with the help of updating operation we can update the new value in the existing element of an listed array easily.
Delete() in C/ C++
Delete is an operator which is used to ravage array and non-array(pointer) objects which are made by new statement. C uses malloc() and calloc() function to designate memory dynamically at run time and uses free() function to free up the dynamically allocated memory.
C++ supports these funtion and also have some operator new and delete that execute the task of designating and freeing-up the memory in a better and easier way.
Question
What is a delete operator?
Delete an element in array
Step by step detailed logic to discard element from array :-
- Move to the stated location which you want to remove in given array.
- Copy the next element to the present element of array, Which is you need to perform array[i] = array[i + 1].
- Repeat above steps till you reached to the last element of array.
- Finally decline the size of array by one.
Algorithm For Deletion Operation in Array :-
Consider LA as a liner array with N elements and K is a positive integer such that K<=N.
- Start
- Set J = K
- Repeat step 4 and 5 while J < N
- Set LA[] = LA[J + 1]
- Set J = J+1
- Set N = N-1
- Stop
Now through this program the process of deleting the element from array will be more cleared :-
#include<iostream> //_____(1)
using namespace std; //____(2)
int main()
{
int a[50],x,n,i,j,b[50]; //___(3)
cout<<"How many elements of array you want to create"; //___(4)
cin>>n; //___(5)
cout<<"\nEnter elements of array\n"; //___(6)
for(i=0,j=0; i<n; ++i) //___(7)
cin>>a[i]; //____(8)
cout<<"\Enter element to delete:"; //___(9)
cin>>x; //___(10)
for(i=0,j=0;i<n;++i) //___(11)
{
if(a[i]!=x) //___(12)
b[j++]=a[i]; //__(13)
}
if(j==n) //__(14)
{
cout<<"\nSorry!!!Element is not in the array"; //___(15)
exit(0);
}
else
{
cout<<"\nNew array is"; //___(16)
for(i=0;i<j;i++) //___(17)
cout<<b[i]<<" "; //_____(18)
}
return 0;
}
Output:-
How many elements of Array you want to create? 5
Enter elements of Array
14 8 3 6 9
Enter element to delete:6
New Array is 14 8 3 9
According to this program :-
(1) It is stand for input output stream. It is basically a header file with C++ standard library.
(2) Using namespace std means you are going to use any class or function from "std" namespace.
(3) Here we define the size of array list a-b which is [50] and what variables we need also defined at this stage.
(4) Here we ask for input from the user.
(5) Here we let the values be "n" which user going to provide.
(6) Here we again ask the user to input the array list.
(7) Here we use "For loop" which will execute upto n times from (0 to n-1),and (++i) means that the value i increased by 1 after execution of particular statement.
(8) Here we put the i which in array list of size a[50] -> i .
(9) Here we ask user for input. That how many values user want to delete.
(10) Here we let the value of "x" be the value which user want to delete.
(11) Here we use "For loop" which will execute upto n times from (0 to n), and (++i) means that the value i increased by 1 after execution of particular statement.
(12) Here we use "IF loop" which will only pass to next statement after processing the present statement, which says if the input value to be deleted is not present in the list then the value will incremented to next value (J++).
(13) Here the incremented value equally added in the array list.
(14) Here we again use "IF loop" and check j==n where n is the input by the user.
(15) Here if J is not equal to n the output will be, there is not the same array list provided by the user, and exit
(16) After using "ELSE loop" we will make the condition for if J is equal to n, then the output will the new array, with the correct user input.
(17) Here with applying "For loop" we going to delete the specific value provided by the user.
(18) Then the output will be the b[50] array list without the element which user want to delete.
Delete statically allocated array in C++
A static memory allocated array in C++ looks like:
int array[100];
This array cannot be deleted explicitly and will exist throughout the program. This is because static memory exist throughout the program and is deleted automatically once the program process terminates.
Delete dynamically allocated array in C++
A dynamic memory allocated array in C++ looks like:
int* array = new int[100];
A dynamic memory allocated array can be deleted as:
delete[] array;
If we delete a specific element in a dynamic memory allocated array, then the total number of elements is reduced so we can reduce the total size of this array. This will involve:
array = (int *)realloc(array, sizeof(int) * (N-1));
This deletes an element in true sense.
Time complexity to delete an array
The time complexity to delete an element is O(N) time.
This is because the other elements are shifted in fill the position of the deleted element. This takes linear time. If we use realloc() in C++, the real memory use is reduced but the elements are moved to a new location so it is an overhead.
This is detailed explanation of process of deleting an array, through this program you can delete element from array easily. With this article at OpenGenus, you must have the complete idea to delete an array or delete an element in an array in C++.
I hope you all will able to understand the process of deleting an array.
THANK YOU.