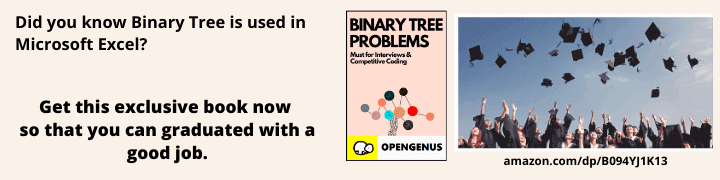
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have compared the differences with Dynamic Memory Allocation and Static Memory Allocation in C Programming Language. We cover the basics of memory and the different types of memory allocation as well.
Table of contents:
- Comparison of Static and Dynamic memory allocation
- What is memory?
- How does a C program uses Memory?
- Static Memory Allocation
- Problems faced in Static memory allocation
- Functions used for Dynamic memory allocation
Comparison of Static and Dynamic memory allocation
STATIC MEMORY ALLOCATION | DYNAMIC MEMORY ALLOCATION |
---|---|
1. It is done before the program execution | 1. It is done during the program execution |
2. Uses Stack for managing static memory allocation | 2. Uses Heap for managing dynamic memory allocation |
3. Variables get allocated permanently | 3. Variables are not allocated permanently |
4. No memory reusability | 4. There is memroy resuability. |
5. It is less efficient | 5. It is more efficient |
6. The execution is faster than dynamic memory allocation |
6. The execution is slower than static memory allocation |
7. Memory remains allocated from start till the end of the program. |
7. Memory can be released at any time during the program. |
What is Memory?
Memory is a collection of small units called Bytes. It is used to store data, like programs, images, audio, etc. Managing memory in a C program is very interesting as it tests the intellect of the programmer in managing work even with limited memory.
How does a C program uses Memory?
For a C program, the memory is divided into 3 parts:
- Stack: Local variables that are created by functions are stored here.
- Heap: It is the memory space located between Stack and Permanent storage area.
- Permanent storage area: The program instructions and global and static variables are stored here.
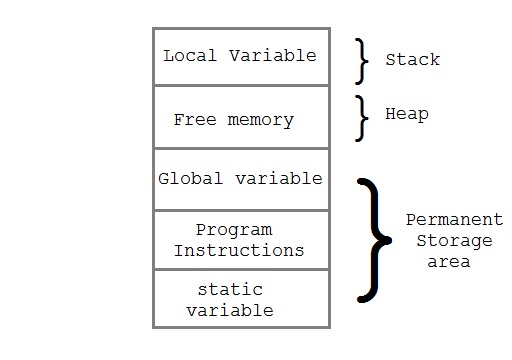
Static Memory Allocation
The memory allocated during the runtime is called Static Memory.
The memory allocated is fixed and cannot be increased or decreased during run time.
The memory allocation and deallocation is done by compiler automatically.
Variables get allocated permanently.
Example:
int main(){
int arr[5]={1,2,3,4,5};
}
Problems faced in Static memory allocation
- While implementing Static memory allocation or allocating memory during compile time then programmer have to fix the size at the time of declaration. Thus programmer cannot increase or decrease the size of the array at run time.
- If the values stored by the user in the array at run time is less than the size specified then there will be wastage of memory.
- If the values stored by the user in the array at run time is more than the size specified then the program may crash or misbehave.
Dynamic Memory Allocation
The process of allocating memory at the run time is known as Dynamic memory allocation.
The memory space that is located between Stack and Permanent storage area, which is called Heap, is used for Dynamic memory allocation during the execution of the program.
Here, the size of the heap keeps changing when the program is executed due to creation and death of variables that are local to functions and blocks.
Everything is done during Run-time or Execution-time.
Memory size can be relocated if needed.
There is no memory wastage.
The functions used for Dynamic memory allocation:
- malloc: Allocating a block of memory
- calloc: Allocating multiple blocks of memory
- free: Releasing the used space
- It is not the pointer that is being released but rather what it points to.
- To release an array of memory that was allocated by calloc we need only to release the pointer once. It is an error to attempt to release elements individually.
- realloc: Altering the size of a block
The malloc function reserves a block of memory of specified size and returns a pointer of type void.We can assign it to any type of pointer.
SYNTAX:
ptr=(cast-type *) malloc(byte-size);
EXAMPLE:
x=(int *) malloc(100 *sizeof(int));
Here, a memory space equivalent to "100 times the size of an int" bytes is reserved and the address of the first byte of the memory allocated is assigned to the pointer x of type of int.
It can also be used to allocate the space for complex data types such as Structures.
Remember, the malloc allocates a block of contiguous bytes. The allocation can fail if the space in the heap is not sufficient to satisfy the request. If it fails, it returns a NULL.
It is normally used for storing the derived data types such as Arrays and Structures. It allocates multiple blocks of storage, each of the same size, and then sets all bytes to zero.
SYNTAX:
ptr=(cast-type *)calloc(n,elem-size);
It allocates contiguous space for n blocks, each of size elem-size bytes. All bytes are initialized to zero and a pointer to the first byte of the allocated region is returned. If there is not enough space, a NULL pointer is returned.
As already used memory is physically used by our system, as a programmer it is our responsibility to release that block of memory at the end of the program or when it is no longer required. It is very important when the storage is limited.
In between the program execution, it is very likely to be the case when we do not require the allocated memory. So we can free/release that block of memory for future use, using the free function. Using this feature, brings out the optimality in the program.
SYNTAX:
free (ptr);
Here, ptr is a pointer to a memory block, which has already been created by malloc or calloc.
Use of invalid pointer may cause system crash.
Points to remember:
It is likely that we later want to change the previously allocated memory as it was not sufficient. Also it can be possible that the memory size already allocated is much larger tha necessary and we want to reduce it. For both the purposes, we can use the function realloc.
EXAMPLE:
Original allocation was-
ptr=malloc(size)
then reallocation of space may be done by-
ptr=realloc(ptr, newsize);
With this article at OpenGenus, you must have a clear idea of Dynamic vs Static memory allocation in C.