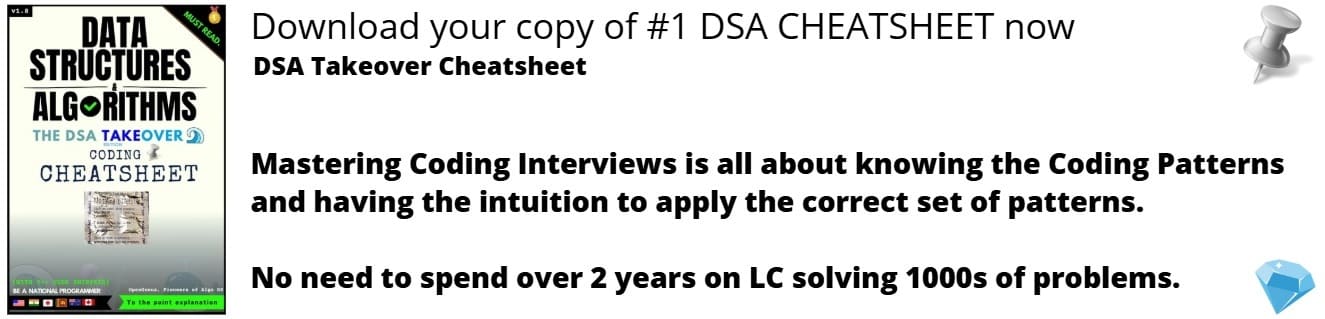
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 25 minutes | Coding time: 45 minutes
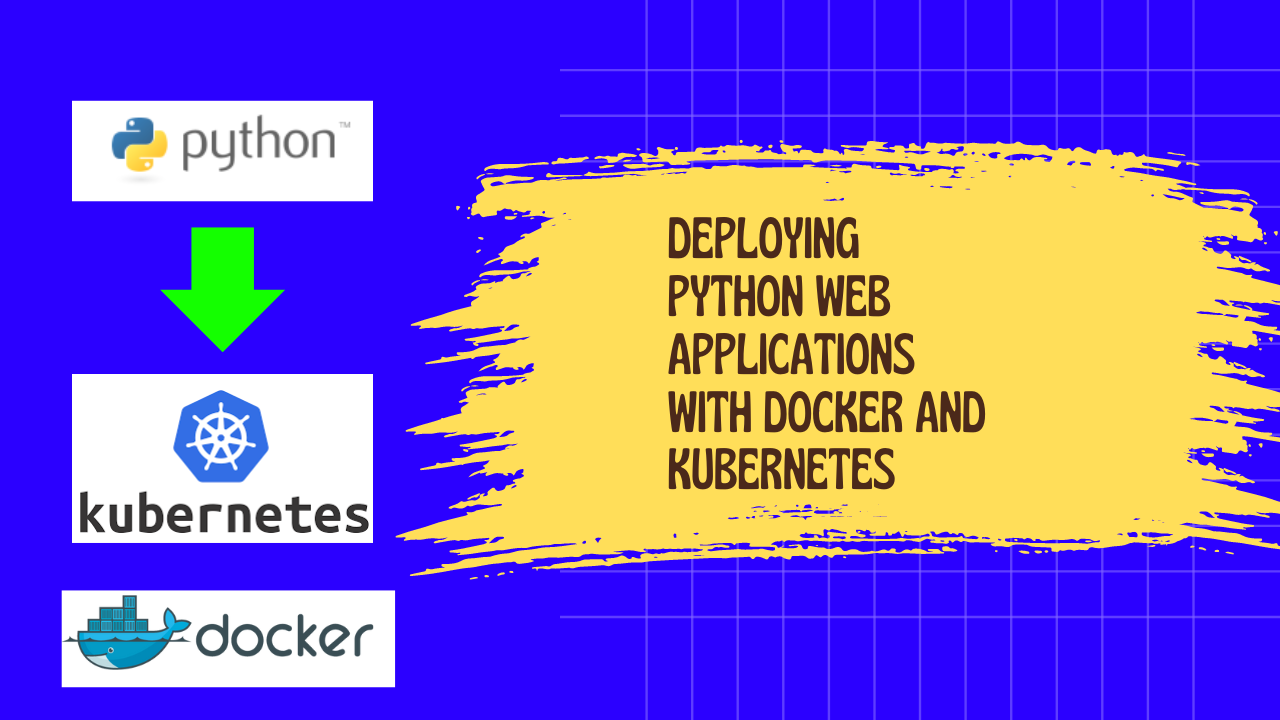Introduction
Deploying Python web applications has become increasingly efficient with the advent of containerization technologies like Docker and orchestration tools like Kubernetes. This OpenGenus.org guide will take you through the process of containerizing a Python web application using Docker and deploying it on a Kubernetes cluster. By the end, you will have a solid understanding of how to create, manage, and scale your applications in a cloud-native environment.
Setting Up Docker
Installing Docker
Docker is a platform designed to make it easier to create, deploy, and run applications by using containers. Containers allow a developer to package up an application with all parts it needs, such as libraries and other dependencies, and ship it all out as one package.
1. Download Docker: Visit Docker's official website and download Docker Desktop for your operating system.
2. Install Docker: Follow the installation instructions for your OS. For example, on macOS, drag the Docker icon to your Applications folder.
Verifying Docker Installation
To verify that Docker is installed correctly, open a terminal and run:
docker --version
You should see the Docker version information, confirming that Docker is installed and running.
Dockerizing a Python Application
Creating a Sample Python Web Application
Let's create a simple Flask application. First, set up a project directory:
mkdir flask_app
cd flask_app
Inside this directory, create a file named 'app.py':
from flask import Flask
app = Flask(__name__)
@app.route('/')
def hello_world():
return 'Hello, Docker!'
if __name__ == '__main__':
app.run(host='0.0.0.0')
Creating a Dockerfile
A Dockerfile is a text document that contains all the commands to assemble an image. Create a file named Dockerfile in the same directory as 'app.py':
# Use an official Python runtime as a parent image
FROM python:3.8-slim
# Set the working directory
WORKDIR /app
# Copy the current directory contents into the container at /app
COPY . /app
# Install any needed packages specified in requirements.txt
RUN pip install flask
# Make port 80 available to the world outside this container
EXPOSE 80
# Define environment variable
ENV NAME World
# Run app.py when the container launches
CMD ["python", "app.py"]
Building the Docker Image
Build the Docker image using the Dockerfile:
docker build -t flask-app .
Running the Docker Container
Run the Docker container using the image you just built:
docker run -p 4000:80 flask-app
Your application should be accessible at 'http://localhost:4000'.
Setting Up Kubernetes
Installing Minikube
Minikube is a tool that lets you run Kubernetes locally. To install Minikube:
1.Download Minikube: Follow the instructions for your OS on the Minikube installation guide.
2.Start Minikube: Once installed, start Minikube with:
minikube start
Verifying Minikube Installation
Verify your Minikube installation by running:
kubectl cluster-info
This command should display information about your Kubernetes cluster.
Deploying with Kubernetes
Creating a Kubernetes Deployment
Create a deployment YAML file named 'deployment.yaml':
apiVersion: apps/v1
kind: Deployment
metadata:
name: flask-app-deployment
spec:
replicas: 3
selector:
matchLabels:
app: flask-app
template:
metadata:
labels:
app: flask-app
spec:
containers:
- name: flask-app
image: flask-app
ports:
- containerPort: 80
Creating a Kubernetes Service
Create a service YAML file named 'service.yaml' to expose your deployment:
apiVersion: v1
kind: Service
metadata:
name: flask-app-service
spec:
type: NodePort
selector:
app: flask-app
ports:
- protocol: TCP
port: 80
targetPort: 80
nodePort: 30001
Applying the Deployment and Service
Apply the deployment and service using 'kubectl':
kubectl apply -f deployment.yaml
kubectl apply -f service.yaml
Accessing the Application
To access the application, get the Minikube IP and open it in your browser:
minikube ip
Your application should be accessible at 'http://<minikube_ip>:30001'.
Applications
Docker and Kubernetes are widely used in various applications, including:
1.Microservices Architecture: Simplifying the deployment and management of microservices.
2.CI/CD Pipelines: Automating the build, test, and deployment processes.
3.Scalable Web Applications: Managing web applications with high availability and scalability.
4.Development Environments: Providing consistent and isolated development environments.
Complexity
Time Complexity
1.Deployment Time Complexity: Dependent on the complexity and size of the application; typically O(1) for running existing container images.
2.Scaling Complexity: O(1) for adding more replicas in Kubernetes.
Space Complexity
1.Docker Image Size: Dependent on the base image and application size.
2.Resource Allocation: Kubernetes manages resource allocation efficiently.
Key Takeaways
Key Takeaways
- Docker simplifies the packaging of Python applications by containerizing them.
- Kubernetes orchestrates containerized applications, providing scalability and high availability.
- Minikube allows local Kubernetes development, simulating a full Kubernetes environment.
- Deploying a Flask application with Docker and Kubernetes involves creating Dockerfiles, Kubernetes deployment, and service configurations.
- Docker and Kubernetes are essential tools for modern DevOps practices, enhancing development and deployment workflows.
Conclusion
Deploying Python web applications with Docker and Kubernetes offers a powerful, scalable, and efficient way to manage your applications. By containerizing your application with Docker, you ensure consistency across different environments, simplifying both development and deployment processes. Kubernetes, on the other hand, provides the orchestration capabilities needed to manage, scale, and maintain these containers in a production environment.
In this guide, we covered the essential steps to get you started:
- Creating a Dockerfile to containerize your Python application.
- Building and running Docker images to verify functionality.
- Setting up Kubernetes with Minikube to create a local development cluster.
- Deploying and managing your application on Kubernetes, including setting up deployments and services.
By leveraging these technologies, you can achieve greater flexibility and control over your application's lifecycle, from development to production. Docker and Kubernetes not only streamline deployment processes but also provide the tools necessary for modern DevOps practices, enabling continuous integration and continuous delivery (CI/CD).
As you continue to explore and implement these tools, you will uncover more advanced features and capabilities, such as monitoring, logging, and automated scaling. These will further enhance your ability to deliver robust and reliable applications. Keep experimenting, and donβt hesitate to dive deeper into the rich ecosystems of Docker and Kubernetes.
Happy deploying!