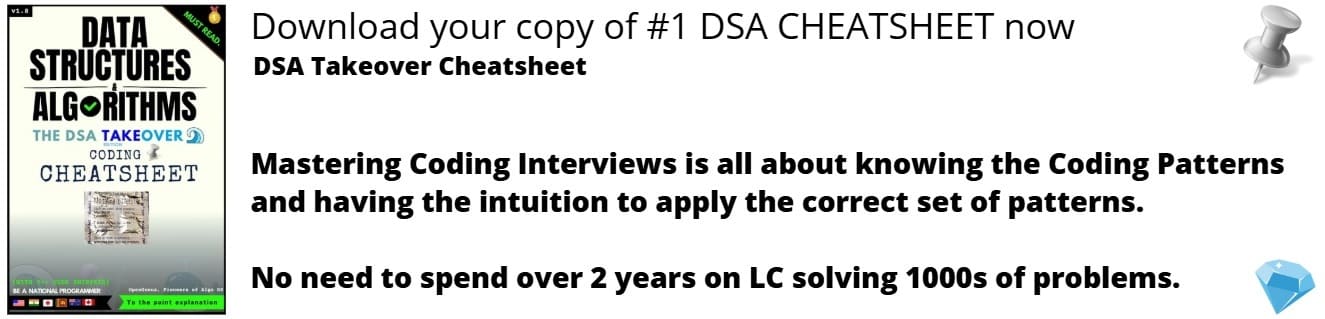
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Table of contents
- Detecting feature support in Javascript
- Detecting CSS support using @supports
- Using Modernizr to detect feature support
Sometimes you want to support a large variety of browsers, while still being able to use features provided by newer browsers. Other times, different browsers might provide different ways of doing the same thing. In both these cases, you’ll need to detect which features are supported by some browser and adapt accordingly. By checking whether a feature is supported or not, and executing a different block of code for each scenario, you can make your website more adaptable and reach more browsers (and people using the browsers).
Detecting feature support in Javascript
To detect the availability of a feature, you can simply type out the variable that a browser provides the features through. If the feature is not available, the variable will turn out to be undefined.
Here is a demonstration. If you type navigator.geolocation
it will give the value undefined on browsers that don’t support geolocation, and provide an object to use the geolocation API in others. To handle both cases, your code might look like :
if (navigator.geolocation) {
// supports geolocation
}
else {
// doesn’t support geolocation
}
It is recommended to use the in
keyword to check for availability, as it works for strict mode too. Therefore, the if-clause above would change to
if(‘geolocation’ in navigator)
And the final code then would be :
if ('geolocation' in navigator) {
// geolocation is supported, so use it
}
else {
// Geolocation not supported, do something else
}
This works because any variable name is undefined by default until we go ahead and define it. It's not only the programmer who defines variables, browsers too define some variables beforehand and provide their features through these variables. For example, think of a random feature name - I’ve thought of ‘timeMachine’. If you type out timeMachine
in a javascript console, it will give back undefined
. However, some browser from the future might decide to implement a time machine feature, and provide it through the variable timeMachine
. In that browser, the variable timeMachine
will not be undefined
anymore, because it has decided to define it. Similarly, browsers which can provide geolocation features decide to provide the option through navigator.geolocation
which remains undefined
on older browsers.
Checking properties on HTML elements
Features apply to HTML elements in certain situations. This happens in case of flexbox, which will only apply to an HTML element, or canvas, which also relies on <canvas>
element. To detect feature support in this case, you’ll first need to create and access the html element in code. To create elements, use document.createElement
. Here is a way to create a div, and check whether it supports the justify-content property. Note how Javascript switches to camel case, and justify-content
becomes justifyContent
.
let div = document.createElement(‘div’)
if (div.style.justifyContent) { // justify-content becomes justifyContent in javascript
}
else{
// feature is not supported. Do something else
}
The case to check for canvas is just slightly different. For browsers that support canvas, the canvas element will have some special methods, like getContext
. In other browsers, it will behave like a default element (yes, you can create html tags of your own), and will not have the getContext property set. Because default elements don't have a method getContext
, simply checking for presence of the method allows you to check whether canvas is just another default element to this browser, or something more.
let canvas = document.createElement(‘canvas’);
if (canvas.getContext) {
// canvas is not just another random tag
// because random tags don't have a getContext property
}
else{
}
Detecting CSS Support using @supports
You can also check for CSS support directly within CSS syntax using @supports. Everything possible with this technique is also possible with the Javascript technique listed above, but using @supports will help you to keep your style concerns within the CSS file, and help you write cleaner, structured code.
@supports takes a feature declaration in brackets following which you can add the styles you want to apply if the feature is supported. Here is an example to detect support for display:flex
and add some styles to a .flexBox
element if it is supported :
@supports (display: flex) {
.flexBox{
display : flex;
flex-direction : column;
}
}
similarly, for cases with no flex support, you can write
@supports not (display: flex) {
.flexBox {
/** Fallback when flexbox not supported */
}
}
You can also combine the different styles with and
for cases where you want all of the rules to be supported, or with or
incase you want support for at least one. Here is the syntax to check for both flex and backdrop-filter support.
@supports (backdrop-filter: blur) and (display: flex) {
}
And here is the syntax to check for support of at least one of the two.
@supports (backdrop-filter: blur) or (display: flex) {
}
Using Modernizr to detect feature support
Instead of manually using the different ways above for different scenarios, you can also use the Modernizr library to have a single way to detect feature support.
To do this, first download Modernizr from https://modernizr.com/ . At the time of writing, the website asks you to pick the features you want to detect, and then generates a custom JS file for you. Download this custom JS file after picking out your feaures.
Now, add the .js file via a script tag to your HTML. Be sure to add it before other script tags which use Modernizr. Now, edit your html and add a class of no-js to the root html element, like so.
<!doctype html>
<html class='no-js'>
<head>
<!-- more stuff in here -->
<script src='modernizr-custom.js'></script>
</head>
<body>
</body>
</html>
Now, if you examine your html element in the browser, you’ll see that Modernizr has inserted some other classes into the html element.
You can now target these classes directly to apply styles if a certain feature is available. For example, if flexbox is not available, the html element will have a no-flexbox class and if it supports modern flexbox, it will have a flexbox class . In your CSS code, you can handle the situation without flexbox in the following manner :
.flexbox .myBox{
display : flex;
}
.no-flexbox .myBox{
display : grid ; /* use grid on .myBox when flexbox isn’t available
}
You can also chain multiple classes to get the same effect as the @supports and
.no-flexbox.grid div{
// change the div styles when grid is available but flexbox is not
}
Using modernizr in javascript
In javascript, Modernizr provides a Modernizr object, which contains features as keys, and true/false as values indicating whether the feature is supported. For example, to check whether flexbox is supported using Javascript, you can just check for the value of Modernizr.flexbox. Your code might look as follows
if (Modernizr.flexbox) {
// do something if flexbox is supported
}
With this article at OpenGenus, you must have the complete idea of how to Detect if feature is supported in Browser using HTML.