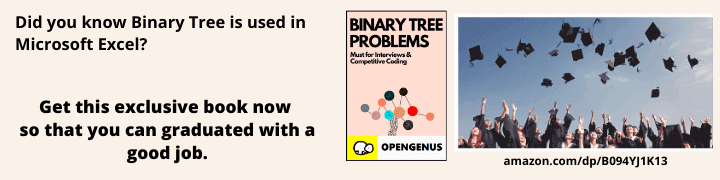
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
As programmers, we often write codes that contain instructions to interact with various input/output devices. In a programming language, the term "output" means to display some data (remember the first "Hello World!" program you started out with?) or write to an output device (could be a monitor, printer or a file).
One of the most powerful general purpose programming languages, C, provides a set of built-in functions to output the required data.
Here, we will discuss the various ways to print in C:
- Formatted and Unformatted Output Functions
- Printing using Formatted Function - printf()
- putchar()
- puts()
I] Formatted and Unformatted Output Functions
The input and output operations in C fall into 2 main categories :
a) Formatted I/O functions:
- As the name suggests, the input or the output data can be modified/formatted as per user-requirements using formatted I/O functions.
- These functions contain format specifiers in their syntax (We will explore more on this in a bit).
- They are more user-friendly and can be used for all data-types.
- Examples : printf(),scanf()
b) Unformatted I/O functions:
- These functions are the most basic form of input and output and they do not allow modifying the input/output in the user-desired format.
- Unformatted I/O transfers data in its raw form(binary representation without any conversions).
- They are simple, efficient and compact. They are mainly used for character or string data types.
- Examples : getch(), putchar(), gets() , puts(), etc.
Well, now that we have got the basics covered, it's time to explore the output functions in detail!
Let's start with the most commonly used function in C to print data (yes, you guessed it right!) : printf()
II] Printing using Formatted Function - printf() :
What happens when the printf() function is called? Let's understand the syntax of the printf() function.
Syntax : printf("format-string",expression...);
Let's break down the above syntax and understand each term properly :
a) format-string consists of :
- The data(text) to be printed as output.
- Format specifiers(optionally present) : Subsequences preceded by the % sign.
Eg : %d,%i, etc.
b) expression :
- For every format specifier present in the printf statement, there must be one matching expression.The arguments present can be any expression of the correct type, but most of the time they are variables whose values have been already computed.
- These expressions are further converted to strings according to the instructions in the corresponding place-holder (format specifier).
- This is then mixed with the regular text in the format-string and the whole string is outputted to the screen.
To understand this better, let's look at an example.
Given, two integers a = 5 and b = 7, display their sum as output.
int a = 5;
int b = 7;
printf("%i + %i = %i \n", a,b,(a+b));
Can you guess what the output would be?
Let us walk through the above snippet of code -
The 3 arguments : a, b and (a+b) will be converted to strings using default formatting. Hence the output of the code will be:
5 + 7 = 12
Did you notice the "%i" used in the above example? That is a format specifier to print integer values.
A format specifier follows the prototype :
%[flags][width][.precision][length]specifier
The specifier character is what defines the type of the corresponding expression in the printf statement.
Let's look at more specifiers that can be used with the printf statement :
1) for character expression - %c:
#include<stdio.h>
int main(void)
{
char ch = 'G';
printf("character = %c",ch);
return 0;
}
The out put of the above code snippet is :
character = G
2) for integer expression - %d or % i:
#include<stdio.h>
int main(void)
{
int num1 = 20;
int num2 = 30;
printf("Number1 = %i\n",num1);
printf("Number2 = %d",num2);
return 0;
}
The out put of the above code snippet is :
Number1 = 20
Number2 = 30
NOTE : While printing an integer, there is no difference between %i and %d. There's a subtle difference while using %i and %d in scanf(), where scanf() assumes the integer to have base 10 for %d and incase of %i, it detects the base of the integer.
3) for floating-point expression -
The floating-point constants or the real constants can be written in two forms - Fractional form or the exponential form.
i) %f :
To print the floating-point constant in the fractional form.
ii) %e, %E: To print the floating-point constant in the exponential form. The only difference between %e and %E is that printf will print the 'e' of the exponential form in lowercase when %e is used and uppercase when %E is used.
iii) %g, %G: To print the floating-point constant in the exponential form when it is very big or very small. Else, it will print the floating-point constant in the fractional form. The only difference between %g and %G is that printf will print the 'e' of the exponential form in lowercase when %g is used and uppercase when %E is used.
iv) %a, %A: The %a formatting specifier is new in C99. It prints the floating-point number in hexadecimal form. This is not something that is user-friendly, but it's very handy for technical use cases.
#include <stdio.h>
int main()
{
float num = 256.78;
printf("Number in floating-point: %f\n",num);
printf("Number in exponential form(lowercase 'e'): %e\n",num);
printf("Number in exponential form(uppercase 'e'): %E\n",num);
/* %g here yields the same result as %f since the number is not very small/very big */
printf("Number in floating-point: %g\n",num);
/* %G here yields the same result as %f since the number is not very small/very big */
printf("Number in floating-point: %G\n",num);
printf("Number in hexa-decimal of floating-point (lowercase): %a\n",num);
printf("Number in hexa-decimal of floating-point (uppercase): %A\n",num);
return 0;
}
The out put of the above code snippet is :
Number in floating-point: 256.77999
Number in exponential form(lowercase 'e'): 2.567800e+02
Number in exponential form(uppercase 'e'): 2.567800E+02
Number in floating-point: 256.78
Number in floating-point: 256.78
Number in hexa-decimal of floating-point (lowercase): 0x1.00c7aep+8
Number in hexa-decimal of floating-point (uppercase): 0X1.00C7AEP+8
4) for Unsigned Octal representation of an integer expression - %o
5) for Unsigned Hexadecimal representation of an integer expression - %x, %X
#include <stdio.h>
int main()
{
int num = 154;
printf("octal representation of %d = %o\n",
num,num);
printf("hexadecimal representation(lower-case) of
%d = %x\n", num,num);
printf("hexadecimal representation(upper-case) of
%d = %X\n", num,num);
return 0;
}
The out put of the above code snippet is :
octal representation of 154 = 232
hexadecimal representation(lower-case) of 154 = 9a
hexadecimal representation(upper-case) of 154 = 9A
6) for strings - %s
#include <stdio.h>
int main()
{
char str[] = "OpenGenus Internship";
printf("%s",str);
return 0;
}
The out put of the above code snippet is :
OpenGenus Internship
Always remember to use the correct specifier that matches the expression. The use of any other letter may result in undefined behavior.
III] Printing using Unformatted functions :
Let's explore two unformatted functions putchar() and puts() for printing data to stdout.
putchar() :
i) This unformatted function in C writes an unsigned character to the stdout.
ii) Syntax : int putchar(int char)
A) If you observe the syntax of the putchar() function, notice it accepts one parameter (a character). This character is written out to the stdout.
Hence it is mandatory to pass a character to the putchar() function.
B) Also, notice this function returns a non-negative integer if successful. On encountering an error, it returns EOF.
Let's look at examples which illustrate the use of putchar() function :
#include<stdio.h>
int main(void)
{
//storing the charachter to be written to stdout
char char = 'D';
//transfers data to stdout using the putchar() function
putchar(char);
return 0;
}
The output of the above code snippet will be as follows :
D
Let's print odd numbers from 1 to 9 using the putchar() function.
Note : putchar() can output only one character to the stdout at a time. Hence to print the odd numbers from 1 to 9, we will make use of the for loop.
#include <stdio.h>
int main()
{
char ch = '1';
// Write the Character to stdout
for (ch = '1'; ch <= '9'; ch=ch+2)
putchar(ch);
return (0);
}
The output of the above code snippet will be as follows :
13579
puts() :
i) This unformatted function in C writes a string(doesn't include the null character) to the stdout.
ii) Syntax : int puts(const char * str)
A) If you observe the syntax of the puts() function, notice it accepts one parameter (a string literal). This string is written out to the stdout.
Hence it is mandatory to pass a string literal to the puts() function.
B) Also, notice this function returns a non-negative integer if successful. On encountering an error, it returns EOF.
Let's look at examples which illustrate the use of puts() function :
#include<stdio.h>
int main(void)
{
char str[15] = "OpenGenus Org";
char str1[20] = "Write and learn!";
puts(str);
puts(str1);
return 0;
}
The output of the above code snippet will be as follows :
OpenGenus Org
Write and learn!
Notice how a new line is appended after each statement in the above output.The output of puts() function is the string passed as an argument appended by a new line.
Well, how about try a question to put this newly acquired knowledge to test?
int main(void)
{
int num1 = 34567;
float num2 = 3456.765;
printf("num1 = %.8d\n",num1);
printf("%num2 = .4f\n",num2);
return 0;
}
Question: What is the output of the above code snippet?
Hope you answered the question correctly, if not, understand the concept of format specifiers again and retry.
There are many more such in-built functions in C for printing data onto files and other output devices.The above mentioned are the most commonly used and very important when it comes to printing operations in C.
Try out all the examples mentioned above on your own and add your own variations to learn more. Keep exploring and learning :)