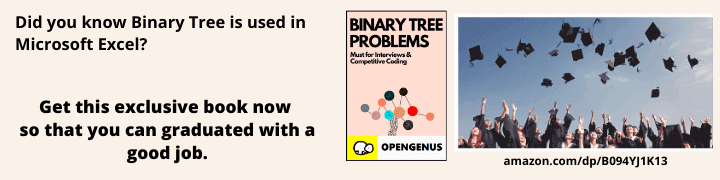
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Table of content:
- Introduction
- Understanding Prime Numbers
- What is Flask
- What is The Sieve of Eratosthenes algorithm
- Creating the Application
- Creating the HTML Template
- Run Application
Introduction:
Prime numbers have fascinated mathematicians and computer scientists for centuries due to their unique properties and applications in various fields. In this article at OpenGenus, we will explore how to build a Flask web application that generates a list of all prime numbers using server-side calculations. Flask is a lightweight and versatile web framework in Python, making it an excellent choice for developing this type of application.
Understanding Prime Numbers:
Before diving into the code, let's briefly review prime numbers. A prime number is a natural number greater than 1 that has no positive divisors other than 1 and itself. For example, 2, 3, 5, 7, and 11 are prime numbers. Prime numbers play a vital role in many cryptographic algorithms, number theory, and mathematical research.
What is Flask?
Flask is a popular web framework written in Python. It allows developers to build web applications quickly and efficiently. Flask provides a simple and intuitive interface, making it accessible for both beginners and experienced developers. Its flexibility and scalability make it an ideal choice for various types of projects, from simple prototypes to complex web applications.
What is The Sieve of Eratosthenes algorithm:
The Sieve of Eratosthenes algorithm is a simple and efficient method for finding all prime numbers up to a given limit. It works by iteratively marking the multiples of each prime found, starting from 2. By eliminating the multiples, it identifies the prime numbers among the remaining unmarked numbers. The algorithm is based on the principle that any number greater than 1 that is not marked as composite must be a prime number. The Sieve of Eratosthenes algorithm reduces the time complexity of finding prime numbers by avoiding the need to check divisibility by all smaller numbers. It has a time complexity of O(n log log n), making it one of the fastest algorithms for generating prime numbers.
Creating the Application:
from flask import Flask, render_template, request
app = Flask(__name__)
def sieve_of_eratosthenes(n):
primes = [True] * (n + 1)
primes[0] = primes[1] = False
for i in range(2, int(n ** 0.5) + 1):
if primes[i]:
for j in range(i * i, n + 1, i):
primes[j] = False
return [num for num, is_prime in enumerate(primes) if is_prime]
@app.route('/', methods=['GET', 'POST'])
def index():
if request.method == 'POST':
n = int(request.form['n'])
prime_numbers = sieve_of_eratosthenes(n)
else:
prime_numbers = []
return render_template('index.html', primes=prime_numbers)
if __name__ == '__main__':
app.run()
This code demonstrates a basic web application built using the Flask framework. In order to install Flask you need to open up the terminal and type pip install flask
. The application generates prime numbers using the Sieve of Eratosthenes algorithm and displays them on a webpage.
In our code above we create an instance of the Flask application by the line app = Flask(__name__)
which creates an instance of the Flask application. __name__
is a special Python variable that represents the name of the current module. Then we implement the Sieve of Eratosthenes algorithm. The sieve_of_eratosthenes(n)
function takes an integer n as input and generates a list of prime numbers up to n using the Sieve of Eratosthenes algorithm. This algorithm efficiently determines prime numbers by iteratively marking the multiples of each prime found, starting from 2. Then I have defined the route and view function. The @app.route('/', methods=['GET', 'POST'])
decorator specifies the URL route for the home page of the web application. The associated view function is defined immediately after the decorator.
Handle HTTP requests in the view function is the index()
function for the home page. It handles both GET and POST requests. When a POST request is received the function extracts the value of the form field 'n', converts it to an integer, and calls the sieve_of_eratosthenes()
function to generate the prime numbers. If it's a GET request, an empty list is assigned to prime_numbers.
After that I render the The render_template()
function to render the HTML template named 'index.html'. The template is passed the list of prime numbers as a parameter named 'primes'. Also the line if __name__ == '__main__':
checks if the current module is the main module. If it is i.e. if the script is directly run and not imported as a module, then the app.run() function starts the Flask development server to run the application.
Creating the HTML Template:
Next, let's create an HTML template called index.html, which will display the list of prime numbers generated by the Flask app.
<!DOCTYPE html>
<html>
<head>
<title>List of Prime Numbers</title>
</head>
<body>
<h1>List of Prime Numbers</h1>
<ul>
{% for prime in primes %}
<li>{{ prime }}</li>
{% endfor %}
</ul>
</body>
</html>
In the HTML template, we use Flask's templating engine to loop through the list of prime numbers and display each number as a list item.
Run Application
In order to run the Flask app in your terminal or command prompt, navigate to the directory where you saved the app.py file and run the following command:
python app.py
To view the output open your web browser and go to http://localhost:5000 or http://127.0.0.1:5000 and you should see a webpage titled "List of Prime Numbers" with a list of prime numbers from 2 to 97 because we set the limit to 100 in the code.
You have successfully run the Flask app and seen the output, which is a webpage displaying a list of prime numbers generated by the server-side calculations. Here is how the application should look like when its running:
In this image I have entered the number 100 and once I press generate it shows me all of the prime numbers up to that value.