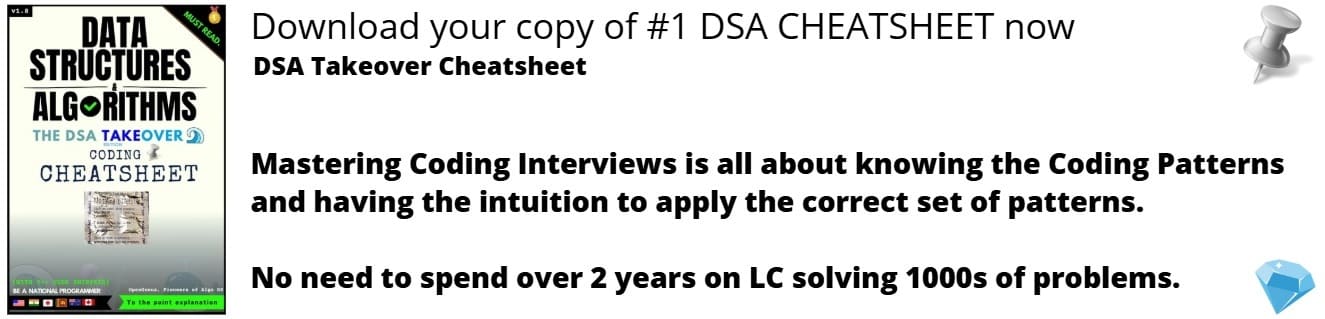
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we'll be discussing on the steps to write a simple Python script that generates a Wiki summary. Firstly we'll discuss on the Introduction to the article. Secondly, This section we'll discussing a bit more on the wikipedia module. After which, we'll be starting the implementation part of the article. At the end we'll be running the article and look into the output of the same.
Following are the sections of this article:-
1. Introduction
We all know that Wikipedia is a free, online Encyclopedia created and maintained by a community of volunteer editors using a wiki-based editing system. It was launched on January 15 2001. So in this article we'll be writing a python script using a python module called wikipedia. This script will be displaying the summary of a topic with decent number of the description of the same. It'll get the information for the searching topic from the Wikipedia
website and it'll display the content at terminal. As a bonus
part in this article we'll also be exploring a bit on the module wikipedia. So lets get started!!
2. Prerequisites
Following are the Prerequisites that an user need to have to note are-
I. The module wikipedia is compatible with Python versions 2.6+ & 3.3+. (You can click on the link to view its documentation at PyPi for other information- wikipedia.
II. In this article the implementation is done with Python version 3.5.2.
3. wikipedia module
There are many modules as such that can be used to perform this operation. We have taken up this module - wikipedia from PyPi, which got a decent documentation to follow. Also you can refer this,
import wikipedia
dir(wikipedia)
['API_URL', 'BeautifulSoup', 'Decimal', 'DisambiguationError', 'HTTPTimeoutError', 'ODD_ERROR_MESSAGE', 'PageError', 'RATE_LIMIT', 'RATE_LIMIT_LAST_CALL', 'RATE_LIMIT_MIN_WAIT', 'RedirectError', 'USER_AGENT', 'WikipediaException', 'WikipediaPage', '__builtins__', '__cached__', '__doc__', '__file__', '__loader__', '__name__', '__package__', '__path__', '__spec__', '__version__', 'cache', 'datetime', 'debug', 'donate', 'exceptions', 'geosearch', 'languages', 'page', 'random', 're', 'requests', 'search', 'set_lang', 'set_rate_limiting', 'set_user_agent', 'stdout_encode', 'suggest', 'summary', 'sys', 'time', 'timedelta', 'unicode_literals', 'util', 'wikipedia']
Following are the couple of sample implementation that this article will be presenting in the up coming section,
A. Getting the summary of any title or topic
B. Change the website language of Wiki (bonus content)
First steps is to create a Virtual environment for Python 3.5.2,
# Creating Virtual environment
~/home/Desktop/wiki_summary$ python3 -m venv wiki_summary
# Now we have to activate this Virtual environment
~/home/Desktop/wiki_summary/$ source wiki_summary/bin/activate
# Post activation of Virtual environment
(wiki_summary)~/home/Desktop/wiki_summary$
Now we have to install the module by running the command at terminal,
# installing wikipedia module
(wiki_summary)~/home/Desktop/wiki_summary$ pip3 install wikipedia
# Create a file called "myscript.py"
(wiki_summary)~/home/Desktop/wiki_summary$ touch myscript.py
We're ready with our environment to start writing the code.
4. Implementation
Below is the complete script which we are exploring in the article, file name- myscript.py
.
import wikipedia
class Mywiki:
def get_summary(self, title, tot_sentence):
return wikipedia.summary(title, sentences=tot_sentence)
def change_language(self, tolanguage, title):
wikipedia.set_lang(tolanguage)
return wikipedia.summary(title)
if __name__ == '__main__':
wiki = Mywiki()
print('-----------------------------------')
print('|Welcome to OpenGenus Wiki Summary|')
print('-----------------------------------')
print('''Available option:
1] Get Summary
2] Change Web-Page Lanuage''')
option = str(input('Please select any one option by typing respective number: '))
if option == '1':
title = input("Enter the topic/title to get the summary:\n")
tot_sentence = int(input("Enter the total number of sentence to get the summary:\n"))
print(wiki.get_summary(title=title, tot_sentence=tot_sentence))
elif option == '2':
tolang = input("Enter the language's first two letters to convert in lower case:\n")
title = input("Enter the topic/title to get the summary:\n")
print(wiki.change_language(tolanguage=tolang, title=title))
else:
print('Please select proper option from the menu')
exit()
Following are the step-wise break-down of the above code snippet-
The above snippet has aclass
calledMywiki
inside which it has two different methods defined.
The method get_summary will internally call the wikipedia's method summary and the method change_language will internally call wikipedia's method called set_lang to set the current language as per user.
Program execution starts from the if statement, and it'll call the respective Mywiki class method as stated below,
A. To Get the Summary from Wikipedia
(This is achieved by calling the method summary from wikipedia module.)
- First step is to run the file at the terminal
(wiki_summary)~/home/Desktop/wiki_summary$ python3 myscript.py
- We get the below output at the terminal,
-----------------------------------
|Welcome to OpenGenus Wiki Summary|
-----------------------------------
Available option:
1] Get Summary
2] Change Web-Page Lanuage
Please select any one option by typing respective number:
- If we select option 1 from the menu,
which is our articles topic to get the wiki summary
and Here in the module of wikipedia it has the method called summary which accepts two arguments -wikipedia.summary(title, sentence)
a.title
- this argument is topic/title (we choose cricket)
b.sentences
- this argument accepts the total number of lines of summary we need, So we choose atotal sentence
as 2.- We get the following output,
-----------------------------------
|Welcome to OpenGenus Wiki Summary|
-----------------------------------
Available option:
1] Get Summary
2] Change Web-Page Lanuage
Please select any one option by typing respective number: 1
Enter the topic/title to get the summary:
cricket
Enter the total number of sentence to get the summary:
2
Cricket is a bat-and-ball game played between two teams of eleven players on a field at the centre of which is a 22-yard (20-metre) pitch with a wicket at each end, each comprising two bails balanced on three stumps. The batting side scores runs by striking the ball bowled at the wicket with the bat (and running between the wickets), while the bowling and fielding side tries to prevent this (by preventing the ball from leaving the field, and getting the ball to either wicket) and dismiss each batter (so they are "out").
B. Change the website language of Wiki (bonus content)
(This is achieved by calling the method set_lang from wikipedia module.)
- First step is to run the file at the terminal
(wiki_summary)~/home/Desktop/wiki_summary$ python3 myscript.py
- We get the below output at the terminal,
-----------------------------------
|Welcome to OpenGenus Wiki Summary|
-----------------------------------
Available option:
1] Get Summary
2] Change Web-Page Lanuage
Please select any one option by typing respective number: 2
Enter the language's first two letters to convert in lower case:
hi
Enter the topic/title to get the summary:
cricket
- Now lets choose option 2, which is to
Change the language of the Wikipedia webpage
and Here in the module of wikipedia it has the method called set_lang, wikipedia.set_lang(one_argument) it accepts an argument, which is a 2 letter language code. We choose as "hi" for HINDI.- Then we have to choose the , we get the summary for any topic. At the end we get the content in HINDI language as output.
- Following is the output,
5. Conclusion
At last we are at the end of the article, In this article we have explored on the new python module called wikipedia, through which we learned on its two methods. We have just discovered it as part of a basic of the module wikipedia.
Hope this was an informative article, Thanks!!