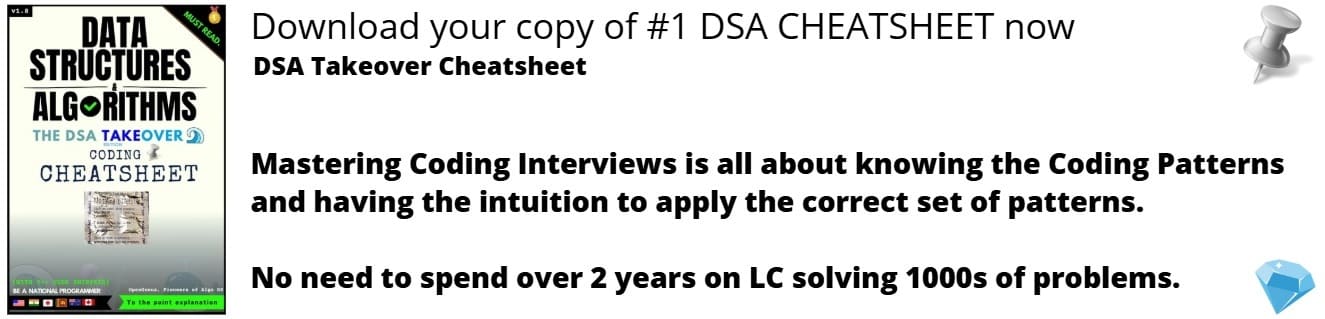
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 30 minutes | Coding time: 5 minutes
#include is a preprocessor directive in C and C++ to include the code from other files (header files + C/ C++ code) in the current file. It is usually used to include header files to impose common API use across different files.
Syntax:
#include <header_file>
// or
#include "header_file>
Example:
#include <stdlib.h>
// or
#include "stdlib.h"
The code statement will arrow brackets #include <stdlib.h>
is used to search the header files (stdlib.h in this case) in the predefined locations of header files for C/ C++.
If we use the second version #include "stdlib.h"
, it will first search the header file in the current working directory and then, if it is not found, it will search in the predefined directories.
With the second version, we can specify the absolute and relative path as well.
To understand where it will search, use the following command:
`gcc -print-prog-name=cc1` -v < /dev/null
Output:
#include "..." search starts here:
#include <...> search starts here:
/usr/lib/gcc/x86_64-redhat-linux/4.8.5/include
/usr/local/include
/usr/include
End of search list.
Analyzing compilation unit
Performing interprocedural optimizations
<*free_lang_data> <visibility> <early_local_cleanups> <*free_inline_summary> <whole-program>Assembling functions:
Execution times (seconds)
phase setup : 0.00 ( 0%) usr 0.00 ( 0%) sys 0.00 ( 0%) wall 2094 kB (90%) ggc
TOTAL : 0.00 0.00 0.00 1210 kB
As given by the above output, by default, it will search in:
- /usr/lib/gcc/x86_64-redhat-linux/4.8.5/include
- /usr/local/include
- /usr/include
Including common header files
In this C code example, we include the stdio.h header file which is needed to use the printf function to give output to stdout stream.
#include <stdio.h>
int main()
{
printf("OPENGENUS");
return 0;
}
Output:
OPENGENUS
We can use the ""
format of include with stdio.h as well. This is because it will find in the current directory filrst and on not finding it, it will eventually, look into the default directories.
#include "stdio.h"
int main()
{
printf("OPENGENUS");
return 0;
}
Output:
OPENGENUS
Using custom header files
In this example, we will create a sample header file in the current directory and include it in our C code.
Header file os.h:
#define __OS__ 1
#define OPENGENUS 1
Code file os.c:
#include <stdio.h>
#include "os.h"
int main()
{
printf("%d\n", OPENGENUS);
return 0;
}
Output:
1
We can define the absolute path as well like:
#include "stdio.h"
#include "/home/opengenus/Desktop/os.h"
int main() {
printf("%d\n", OPENGENUS);
return 0;
}
Output:
1
If the header file is not present, it will give compilation error.
#include <stdio.h>
#include "os_1.h"
int main()
{
printf("%d\n", OPENGENUS);
return 0;
}
Compilation error:
os.c:2:18: fatal error: os_1.h: No such file or directory
#include "os_1.h"
^
compilation terminated.
As os.h is present only in the current directory and not in the default location, it will give error if we use the <>
format for include.
#include <stdio.h>
#include <os.h>
int main()
{
printf("%d\n", OPENGENUS);
return 0;
}
Compilation error:
os.c:2:16: fatal error: os.h: No such file or directory
#include <os.h>
^
compilation terminated.
Including a C code in another C code
In this example, we will create a header file which has actual C code and include it another C code.
Header file os.h:
int get_data()
{
return 1;
}
C code:
#include <stdio.h>
#include "os.h"
int main() {
printf("%d\n", get_data());
return 0;
}
Output:
1
With this, you will have the complete working knowledge of using #include in C and C++ code.