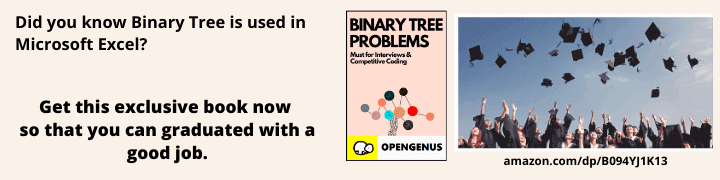
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 20 minutes
memset is a memory utility in C which is used to set a particular value to a range of memory locations in an efficient way. On going through this article, you will understand:
- How to use memset?
- Why performance of memset is better than a simple implementation?
How to use memset?
memset() is built in standard string function that is defined in string header library string.h
. Therefore, to use this function we should include string.h.
#include<string.h>
This function
void *memset(void *str, int c, size_t n)
copies the character c (an unsigned char) to the first n characters of the string pointed to, by the argument str. This function copies ch (represented as unsigned char) into the first n characters of the object or memory block pointed by str.
memset() returns a pointer to the block of memory.
memset takes three parametrs:
- ptr: pointer to the memory location which needs to be set
- value: value that needs to be set
- num: number of bytes to be set
Following are the types of the above three parameters:
- ptr: const void* (as it is a pointer)
- value: int (can be seen as unsigned char)
- num: size_t
Following diagram captures the idea:
Declaration
Example of using memset:
#include<stdio.h>
#include<string.h>
int main()
{
//initializing character array
char str[ 30 ] = "Memset example";
//displaying str
printf("Normal String = %s\n\n", str);
memset(str+14, '!', 5);
printf("String after memset = %s\n",str);
return 0;
}
Output
Normal String = Memset example
String after memset = Memset example!!!!!
Why performance of memset is better than a simple implementation?
We can write a C implementation to loop through all required memory locations and set it one by one to the specific value. This approach will be slower than compared to memset even through the basic procedure followed by memset is same.
There are a couple of reasons for this like:
- memset uses special instructions which set 16 bytes of memory at once
- memset has multiple implementations using are used depending upon the memory layout which is a complicated process and cannot be performed efficiently with a C compiler
- In fact, for a particular situation, it can be performed in O(log N) instead of O(N) by using a particular page structure and references
Question
Consider the following C code:
#include<stdio.h>
#include<string.h>
int main()
{
char str[ 30 ] = "Memset example";
memset(str+14, str[5], 5);
for(int i=1;i<5;i++)
{
if((i%i)*0!=0)
{
memset(str+14, '!', 5);
printf("String after memset = %s\n",str);
return 0;
}
}
printf("String after memset = %s\n",str);
return 0;
}