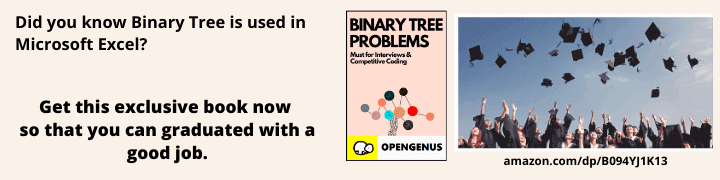
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 20 minutes | Coding time: 5 minutes
A Tuple is an ordered and immutable collection of objects. Once initialized you may not change them.
Read about Tuples in PythonTuples are used to hold together multiple objects. Think of them as similar to lists, but without the extensive functionality that the list class gives you. One major feature of tuples is that they are immutable like strings i.e. you cannot modify tuples.
NamedTuples are Tuples that allow you to:
- Name each Tuple field
- Access fields by name
- Access fields by index.
Regular Tuples only allow you to access fields by index.
NamedTuples also have methods which help you create new Tuples with existing or new data.
Declaration of a NamedTuple
Note: For this examples we'll use the Python 3 interpreter interactively.
In a 2D graph, we can plot a point with its two dimensional x and y coordinates. If we wish to create a point object with Python, we can create a NamedTuple with two fields, one which corresponds to the x coordinate and one that corresponds to the y coordinate.
Example 2D Point:
First we must import NamedTuples from the Collections module.
>>> from collections import namedtuple
Now declare the NamedTuple and its fields names.
>>> MyPoint = namedtuple('MyPoint', ['x', 'y'])
Then we can create a new instance and instantiate its fields with values. In this case two integers, 5 for x and 3 for y.
>>> point = MyPoint(x=5, y=3)
Access the fields
With Named Tuples we can access fields my name.
>>> print(point.x)
5
>>> point.x + point.y
8
Or we can access them by index.
>>> point[0] + point[1]
8
Question
Named Tuples allow you to access fields by:
Basic Methods for NamedTuples
NamedTuple's methods help you create new Tuples from an iterable object or other existing Tuples.
_make()
This method helps the user initialize a NamedTuple from an iterable object like an Array or a Tuple.
In this example we'll:
- Define and initialize a normal Tuple which contains two values as an example.
- Define a NamedTuple with field names x and y.
- Make a new instance from the existing point Tuple.
>>> a = [2, 4] # normal Tuple
>>> MyPoint = namedtuple('MyPoint', ['x', 'y'])
>>> point = MyPoint._make(a)
Now we can access the values with the names x and y correspondingly.
>>> print(point.x)
2
>>> print(point.y)
4
_asdict()
_asdict returns a new OrderedDict object, mapping field names and values from the NamedTuple.
In this example we'll:
- Define a NamedTuple with field names x and y.
- Initialize the NamedTuple with data.
- Use _asdict to returns an OrderedDict.
>>> MyPoint = namedtuple('MyPoint', ['x', 'y'])
>>> point = MyPoint(x=5, y=3)
>>> point._asdict()
OrderedDict([('x', 5), ('y', 3)])
You may use this method to create a new OrderedDict from a NamedTuple.
_replace()
Tuples are immutable. The _replace method allows the user to create a copy of a NamedTuple and replace certain values with new ones.
>>> MyPoint = namedtuple('MyPoint', ['x', 'y'])
>>> point = MyPoint(x=5, y=3)
>>> point._replace(x=7)
MyPoint(x=7, y=3)
As you see, the last instruction returns a NamedTuple in which x is no longer 5, but 7.
_fields
The _fields method helps to create a normal Tuple which contains a Named Tuple's field names. Only it field names, not its corresponding values.
>>> MyPoint = namedtuple('MyPoint', ['x', 'y'])
>>> point = MyPoint(x=5, y=3) # new instance
>>> point._fields
('x', 'y') # tuple
We can use this method to create a Tuple like this:
>>> b = point._fields
>>> b[0]
x
Conclusion
With this article at OpenGenus, you must have the complete idea of NamedTuples in Python. Using NamedTuples helps you self-document your code conveying meaning to each field. Its methods allow you to work more easily with data.