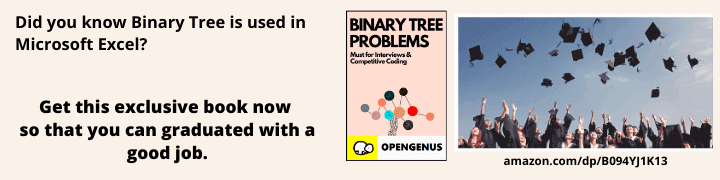
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 30 minutes | Coding time: 10 minutes
The only difference between strncmp and strcmp in C is that strncmp compares only the first N characters while strcmp continues until the termination conditions are met. The termination conditions for both strncmp and strcmp are:
- if two characters are different
- if null character (\0) is reached for any of the strings
Both strncmp and strcmp can be used to compare strings in C but it is strongly advised to use strncmp as on setting N correctly, it prevents buffer overflow which is a real problem in production systems and cause several security concerns.
strncmp is more customizable
Consider two null terminated strings S1 and S2.
When we use strcmp, we have to compare the entire string till the end (null character). In case of strncmp, we can customize this is compare only the first few characters. This is useful in reality as in production system, comparisons are limited to only a section of the data. Even in most platforms/ softwares, only the first character is used for comparison.
Comparing only limited number of characters defines an upper limit of comparison and makes the program behaviour deterministic and more efficient.
Let us take an example:
In this, we are comparing "OPENGENUS" with "OPEN0ENU8". Carefully, observe the difference row.
Points to note:
- If we use strcmp, it will return -8 as it is the first mismatched difference.
- If we use strncmp over the entire string, it will return -8 as well.
- If our system matches only the first 4 characters, we can use strncmp with N=4 and it will return equal string. This is not directly possible with strcmp.
Implementation:
#include <stdio.h>
#include <string.h>
int main()
{
char* s1 = "OPENGENUS\0";
char* s2 = "OPENOENU8\0";
printf("Using strcmp: %d\n", strcmp(s1, s2));
printf("Using strncmp with N=9: %d\n", strncmp(s1, s2, 9));
printf("Using strncmp with N=4: %d\n", strncmp(s1, s2, 4));
return 0;
}
Output:
Using strcmp: -8
Using strncmp with N=9: -8
Using strncmp with N=4: 0
Non null terminated strings
Now, consider the case with non null terminated strings.
Both strings are same but does not have null character at the end. The last character is a garbage value that the value that was stored in the memory location in previous execution of any program.
It is likely to be different and will give different results for different system states. If it is same, the comparison will move to the next character/ memory location which will have garbage as well.
Thus, theoretically, all garbage value can be same and strcmp can end up comparing memory of the entire system. The major concern is that it is accessing memory outside of what it is required to which can lead to security issues.
Thus, in both ways, performance and security, strncmp should be preferred over strcmp.
Implementation:
We illustrated the above situation in this C++ code example:
#include <stdio.h>
#include <string.h>
int main()
{
char s1[] = {'O', 'P', 'E', 'N', 'G', 'E', 'N', 'U', 'S'};
char s2[] = {'O', 'P', 'E', 'N', 'G', 'E', 'N', 'U', 'S'};
printf("Using strcmp: %d\n", strcmp(s1, s2));
printf("Using strncmp with N=9: %d\n", strncmp(s1, s2, 9));
printf("Using strncmp with N=4: %d\n", strncmp(s1, s2, 4));
// wrong value of N
printf("Using strncmp with N=11: %d\n", strncmp(s1, s2, 11));
return 0;
}
Output:
Using strcmp: -5
Using strncmp with N=9: 0
Using strncmp with N=4: 0
Using strncmp with N=11: -5
With this, you have the complete knowledge of working with strncmp and strcmp and the difference behaviour each can produce.