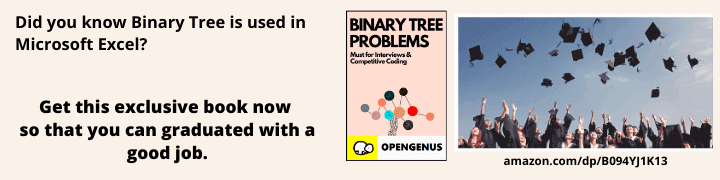
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this post, we will go through how to ZIP a file in Python, how to extract files from a ZIP file or how to print contents of a ZIP file using Python. Before that let's talk about a ZIP file.
What is a ZIP file?
ZIP file is an archive file which supports lossless data compression. It can contain several files and folders or other compressed ZIP files in it. This format permits a number of compression algorithms, though DEFLATE is the most common. So, basically ZIP file is used to compress several large files and folders into a single smaller archive file. Thus, there are several softwares available to create a ZIP file or to extract content from a ZIP file.
Similarly, Python too have several modules to work with ZIP files or to create them or to extract data from them such as zipfile, zipimport, shutil, etc.
Here in this post we will be using module zipfile, shutil. As zipfile, shutil are inbuilt Python modules. Thus, you won't need to install them externally.
Creating a ZIP file using shutil
# importing shutil module
import shutil
#using make_archive method to zip a whole directory
shutil.make_archive(output_filename, 'zip', dir_name)
make_archive() method
It requires three arguments to create a ZIP file:
- base_name - name of the output file, you want to create.
- format - format of the archive file. Such as zip, tar, gztar, bztar, xztar, etc.
- root_dir or base_dir - root_dir is the directory that will be the root directory of the archive or base_dir is the directory where we start archiving from.
Let's talk about the zipfile module in Python
zipfile module provides tools to create, read, write, append, and list a ZIP file. Any advanced use of this module will require an understanding of the format. This module does not currently handle multi-disk ZIP files. It can handle ZIP files that use the ZIP64 extensions (that is ZIP files that are more than 4 GiB in size). It supports decryption of encrypted files in ZIP archives, but it currently cannot create an encrypted file. Decryption is extremely slow as it is implemented in native Python rather than C.
Creating a ZIP file using zipfile
# importing ZipFile class from zipfile module
from zipfile import ZipFile
# more fine-grained control over ZIP files
with ZipFile("file_name.zip", "w") as newzip:
newzip.write("file.txt")
newzip.write("file2.jpg")
write() method
It requires a argument to create a ZIP file.
- filename - type the name of the file you want to add to the ZIP file.
Beside creating ZIP files, we can also extract or display contents from a ZIP file.
How to extract contents from a ZIP file?
# importing ZipFile class from zipfile module
from zipfile import ZipFile
# specifying the zip file_name
file = "file_name.zip"
# opening the zip file in READ mode
with ZipFile(file, 'r') as zip:
# extracting all contents
print('Extracting all the files now...')
zip.extractall()
print('Done!')
extractall() method
extractall() method will extract all the contents of the zip file to the current working directory.
How to print contents from a ZIP file?
# importing ZipFile class from zipfile module
from zipfile import ZipFile
# specifying the zip file_name
file = "file_name.zip"
# opening the zip file in READ mode
with ZipFile(file, 'r') as zip:
# printing all the contents of a zip file
zip.printdir()
printdir() method
printdir() method will print all the contents of a zip file.
There are several other essential methods used from zipfile module. Let's learn about them:
- zipfile.is_zipfile(FILE_NAME) - is_zipfile() method is used to check whether a file is valid ZIP file based on its magic number, otherwise returns False. filename may be a file or file-like object too.
Example
# importing zipfile module
import zipfile
# specifying the zip file_name
file = "file_name.zip"
# using the is_zipfile method
print(zipfile.is_zipfile(file))
- ZipFile.close() close() method of ZipFile class is used to close the archive file. So, that essential records will not be written. As we used with statement in previous examples, which automatically closes the archive file after the end of with statement.
Example
# importing ZipFile class from zipfile module
from zipfile import ZipFile
# closing the zip file
ZipFile.close()
- ZipFile.namelist() namelist() method of ZipFile class returns a list of archive members by name.
Example -
# importing ZipFile class from zipfile module
from zipfile import ZipFile
# specifying the zip file_name
file = "file_name.zip"
# opening the zip file in READ mode
with ZipFile(file, 'r') as zip:
# to get archive members
print(zip.namelist())
- ZipFile.setpassword(PWD) setpassword() method is used to set the password which is used to encrypt the zip file, in order to extract it.
Example
# importing ZipFile class from zipfile module
from zipfile import ZipFile
# specifying the zip file_name
file = "file_name.zip"
# opening the zip file in READ mode
with ZipFile(file, 'r') as zip:
# setting the pwd to extract files
zip.setpassword("ganofins")
# extracting all contents
zip.extractall()
print('Done!')
- ZipFile.infolist() infolist() method is used to return a list containing a ZipInfo object for each member of the archive. The objects are in the same order as their entries in the actual ZIP file on disk if an existing archive was opened.
Example
# importing ZipFile class from zipfile module
from zipfile import ZipFile
# specifying the zip file_name
file = "file_name.zip"
# opening the zip file in READ mode
with ZipFile(file, 'r') as zip:
# to get a list containing a ZipInfo object
print(zip.infolist())
- ZipFile.getinfo(NAME) getinfo() method is used to return a ZipInfo object with information about the archive member name.
Example
# importing ZipFile class from zipfile module
from zipfile import ZipFile
# specifying the zip file_name
file = "file_name.zip"
# opening the zip file in READ mode
with ZipFile(file, 'r') as zip:
# to get a list containing a ZipInfo object
print(zip.getinfo("test"))
Well I hope you understood you to extract files using Python. If you have any query regarding this, feel free to ask us in the discussion section below.
With this article at OpenGenus, you must have the complete idea of creating ZIP files in Python and handle it. Enjoy.