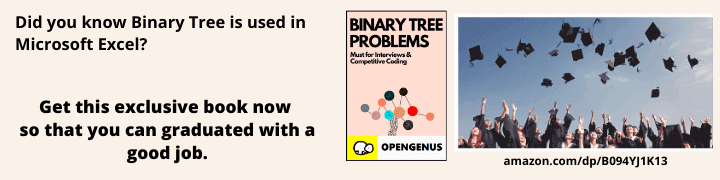
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explored how to delete or deallocate an array in C. By deleting an array, we mean to deallocate the memory that was assigned to the array so that it can be reused. In short, following are the main points regarding it:
- If the array is declared statically, then we do not need to delete an array since it gets deleted by the end of the program/ block in which it was declared.
- If the array is declared dynamically, we need to free the memory allocated to it using the free() function.
Before going to this, we will revisit some basic information regarding arrays, statically allocated array and dynamically allocated array. Following this, we have explored the deallocation and deletion of an array in C.
WHAT IS AN ARRAY?
An array is a named memory location. It is used to store similar types of data (like integer, double, string, boolean, etc.) of fixed size in a contiguous fashion. This means that given the address of a particular value/location we can find out the address of other values using some mathematical calculations since the data is stored back to back.
We use arrays when we need to store a large amount of data. So instead of creating different variables, we make an array of one variable to avoid clutter and confusion. For example if we want to store say 5 integer values, then instead of writing:
int a=1;
int b=2;
int c=3;
int d=4;
int e=5;
we can declare an array like this:
int a[]={1,2,3,4,5};
The lowest address of the array contains the first element which starts at index 0 and the highest address of the array contains the last element ending at an index of length-1.
DECLARATION OF AN ARRAY
There are two ways of declaring an array:
- Static : Statically allocated arrays are the ones declared at compile time. They have a fixed length and execute only in a specific block of code where they are declared.
Note:They are slightly different from arrays declared using static storage class specifier.
The syntax for declaring a static array of type integer and of length 10 is as follows:
- In the code below 10 blocks of memory have been allocated to an integer array and each value of the array is initialized to zero by default.
int n=10;
int arr[n];
OR
int arr[10];
- In the code below 10 blocks of memory have been allocated to an integer array and each value of the array is initialized to the value provided.
int arr[10]={1,4,5,2,3,6,8,7,10,9};
- In the code below 10 blocks of memory have been allocated to an integer array and the first three values (0 to 2) of the array is initialized to the value provided and the rest of the 7 values(3-9) are initialized to 0.
int arr[10]={1,4,5};
- Dynamic : Dynamic arrays are those which are declared at run-time. They are declared initially using
malloc
orcalloc
for a fixed size. Later, they can be resized usingrealloc
which is not possible in static declaration.
Note: Please be careful and remember to free the memory allocated dynamically, else memory leak may occur and the program may crash at some point of time.
The syntax for declaring a dynamic array followed by resizing is as follows:
#define SIZE 6
int *data=malloc(SIZE * sizeof(int));
data=(int*)realloc(data, 10*sizeof(int));
OR
#define SIZE 6
int *data=calloc(SIZE * sizeof(int));
data=(int*)realloc(data, 10*sizeof(int));
In the code above, initially 6 blocks of memory have been allocated to an integer array and each value of the array is initialized to zero. Later with the help of realloc, the size of the array is changed and 4 more blocks of memory have been allocated to the integer array with 0 being initialized to each value.
Difference between static and dynamic arrays:
-
When memory is allocated to an array by specifying the size during compile time, the size of the array gets fixed and cannot be changed at run-time. Such arrays are known as static arrays.
On the other hand, when memory is allocated to an array by specifying the size during run time, the size of the array is not permanently fixed and can be changed at run-time. Such arrays are known as dynamic arrays and are created using pointer variables and allocated memory using memory management functions calloc/malloc and resized using realloc function. -
Static arrays are only used when we know the amount of data beforehand. In cases, where we are not sure of our data requirements, dynamic arrays are used.
-
In static memory allocation, the unused allocated memory is wasted, while in dynamic memory allocation, memory is allocated as and when required and hence minimizes wastage of memory.
-
In static arrays memory is allocated sequentially one after the other i.e. they use stack memory whereas in dynamic arrays memory is not allocated sequentially i.e. they use heap memory. But dynamic arrays can still be tracked since the previous element points to the next element and so on. This dynamic allocation is useful in cases where the computer does not have a specific amount of memory available in a continuous fashion but has it available in a random fashion.
DEALLOCATION/ DELETION OF ARRAYS
Deletion of an array means that we need to deallocate the memory that was allocated to the array so that it can be used for other purposes.
Arrays occupy a lot of our memory space. Hence, to free up the memory at the end of the program, we must remove/deallocate the memory bytes used by the array to prevent wastage of memory.
If the array is declared statically, then we do not need to delete an array since it gets deleted by the end of the program/block in which it was declared.
But if the array is declared dynamically, i.e. using malloc/calloc, then we need to free up the memory space used up by them by the end of the program for the program to not crash down. It can be done by calling the free method and passing the array.
When we allocate memory dynamically, some part of information is stored before or after the allocated block. free
uses this information to know how memory was allocated and then frees up the whole block of memory.
The syntax for doing the same is as follows:
int *a=malloc(10*sizeof(int));
free(a);
In the above example, the whole array a is passed as an argument to the in-built function free which deallocates the memory that was assigned to the array a.
However, if we allocate memory for each array element, then we need to free each element before deleting the array.
char ** a = malloc(10*sizeof(char*));
for(int i=0; i < 10; i++)
{
a[i] = malloc(i+1);
}
for (int i=0; i < 10; i++)
{
free(a[i]);
}
free(a);
In the above code, memory allotted to each element is deleted before actually freeing up the array pointer.
With this article at OpenGenus, you must have a complete idea of deleting or deallocate an array in C. Enjoy.
Learn more:
- 2D array in C by Subhash Bhandari at OpenGenus
- 3D array in C by Subhash Bhandari at OpenGenus
- Variable in C by Subhash Bhandari at OpenGenus
- List of C topics at OpenGenus