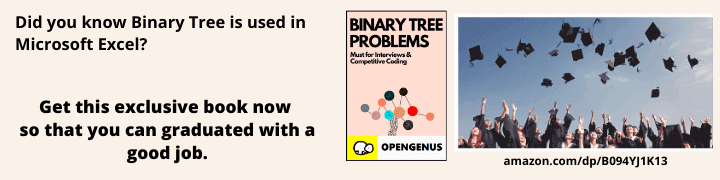
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Variable in C Programming langauge is a name of a placeholder (or memory location) that is used to store values or it is a location in memory of a computer where a program can manipulate the data.
Some points about a variable in C:
- Variable can take different type of values but only one at a time.
- The values of the variable can be changed during the execution of the program.
- With each variable there is a datatype associated with it which decides the type of values a variable will hold like int, char, float, double etc.
Rules for naming variables in C
For naming variables in C language we have a set of rules that needs to be followed:
- Variable name must begin with letter or underscore.
example:
int opengenus; //variable name starting with a letter
int _opengenus; //variable name starting with an underscore
- Variables are case sensitive. It means the name of the variable can be written in lower case letter or in upper case letter.
example:
int opengenus; //variable name in lower case
int OPENGENUS; //variable name in upper case
- No special characters or symbol can be used for defining the variable.
example:
int $opengenus; //variable starting with a special character is not valid
int opengenus; //is valid
- No spaces are allowed.
example:
int open genus; //variable name with blank spaces are not valid
int open_genus; //is valid
- A variable name must not be any reserved words or keywords.
example:
int break; // break is a keyword in C language, can't use it as a variable name
int OpenGenus; // this is a valid variable name
Declaration of variables in C language.
- Before using the variables in c, there is a need to declare them.
- While declaring the variables we can define the datatype of the variables.
data-type variable_name;
example:
int a;
char b;
float c;
long d; etc.
Here a is a variable of type int, b is a variable of type char, c is a variable of type float, d is a variable of type float and d is variable of type long.
We can also declare more than one variable in a single declaration.
example:
int a, b, c;
Here a,b and c are the variables of datatype int.
- During the declaration no memory space is allocated to the variable.The space is allocated during variable definition.
Initialization of variables in C
- When a variable is declared it contains undefined value known as garbage value. So we need to assign some values to the variable.
- Assigning values to the variables is known as intialization of variables.
example:
int a=5;
float b=2.3, c=5.4;
char d[]="Opengenus";
double e=0.15197e-7;
int x,y,z=1; //only variable z is initialized
let's take an example:
#include<stdio.h>
int main()
{
int a,c; //variables are declared but not initialized
int b=3; //variable is declared as well as defined
a=2; //value assigned to varable a
c=a+b;
printf("%d",c);
return 0;
}
Types of variables in C language
- Local variable
- Global variable
- Static variable
- Automatic variable
- External variable
1) Local variable
Local variables are the variables which are defined or declared within a function or within the blocks.Local variables are accessed only by those statements which are written in the same block.They can't be used outside the function or block.
example:
#include<stdio.h>
int main()
{
int a=5,b=6,c; //variables are declared inside the function
c=a+b;
printf("%d",c);
return 0;
}
Output:
11
2) Global variable
Global variables are the variables which are declared outside the function and can be accessed easily by any of the functions defined in the program.Once it is declared, it can be used throughout the program.
example:
#include<stdio.h>
int c; //global declaration
int main()
{
int a=5,b=6; //local declaration
c=a+b; //variable c is used here which is globally declared
printf("%d",c);
return 0;
}
Output:
11
There is another case of global declaration of variables.We can globally declare and initialize a variable and when we access it locally inside a function or block, we can re-initialize it.
example:
#include<stdio.h>
int b=20; //globally variable b is initialized as 20
int main()
{
int a=10,b=10,c; //variable b is re-initialized as 10
c=a+b;
printf("%d",c);
return 0;
}
Output:
20
3) Static variable
Static avriables in c are the variables which have a property of retaining their value between multiple function calls.A variable can be declared static using the static keyword.
static int a;
static float b;
#include<stdio.h>
int main()
{
fun();
fun();
fun();
return 0;
}
void fun()
{
int a=5; //local variable
static int b=5; //static variable
a=a+1;
b=b+1;
printf("a=%d\tb=%d\n",a,b);
}
output:
a=6 b=6
a=6 b=7
a=6 b=8
Here the local variable a is initialized as 5 and the static variable b is also initialized as 5 and the function "fun()" is called three times in the main program.
In the output it is clear that in every function call, local variable a holds same value but the static variable b is retaining the previous value and displaying the incremented value.
4) Automatic variable
The variables which are declared inside the block are automatic variables by default.The keyword auto is used to declare autoamtic variables but it is rarely used while writing programs in C language.The automatic variables can only be accessed within the block or function they have been declared and not outside them.
#include<stdio.h>
int main()
{
fun();
fun();
fun();
return 0;
}
void fun()
{
auto int a=5; //auto int or simply int
a=a+1;
printf("a=%d\n",a);
}
Output:
a=6
a=6
a=6
5) External variable
In this type of variable we have a keyword called extern to share the variable between two source files.
extern keyword is used for declaring a global variable in another file to provide the reference of variable which have been already defined in the original file.
Let's take an example:
We have two files f1.c and f2.c.
f1.h contains:
#include<stdio.h>
a=50;
f2.h contains:
#include"f1.h" //file containing external variable is included here
#include<stdio.h>
extern int a;
int main()
{
printf("value of external interger is:%d",a);
return 0;
}
Learn more:
- Get address of a variable in C
- Ternary Operator in C by Shobhit Sinha
- Understand Pointers in C in depth by Shobhit Sinha
- List of C topics at OpenGenus
With this, you have the complete knowledge of variables in C. Enjoy.