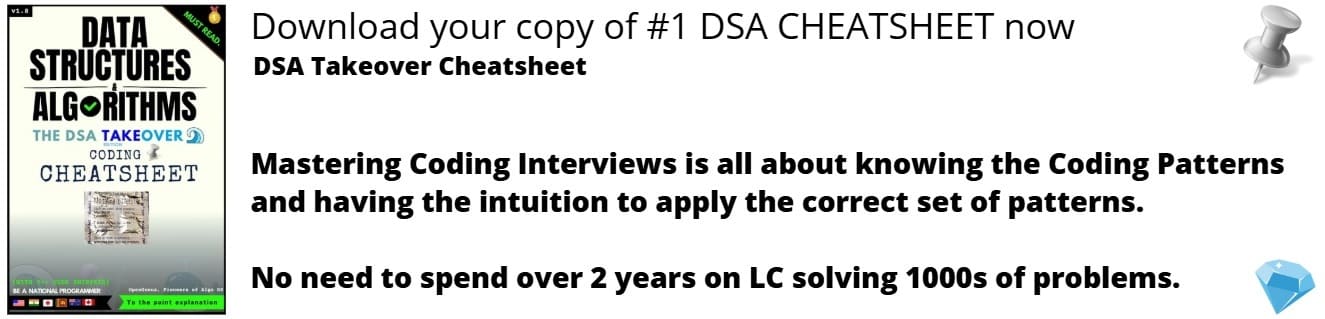
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 30 minutes | Coding time: 5 minutes
In C, we can get the memory address of any variable or member field (of struct). To do so, we use the address of (&) operator, the %p specifier to print it and a casting of (void*) on the address.
If you variable is named opengenus, you can get the address as follows:
// opengenus is a variable
printf("%p\n", (void *)&opengenus); // recommended
// or
printf("%p\n", &opengenus);
Note:
- We have used & before opengenus to get the address in form of a pointer
- We have casted the address to (
void *
) as address/ pointers should be of this type. - We print it using %p specifier of printf.
- Using (
void *
) casting is optional but is strongly recommended as in C, pointers to memory location should be of type "void *
by default.
The output is the memory address of the variable (opengenus in our case) like:
0x7ffffefa8bc4
Complete code example:
#include <stdio.h>
int main()
{
int opengenus = 1;
printf("%p\n", (void *)&opengenus);
return 0;
}
Output:
0x7ffffefa8bc4
We can save the address in a pointer variable as well like:
int opengenus = 1;
void* address = (void *)&opengenus;
You can print it as:
printf("%p\n", address);
Complete example:
#include <stdio.h>
int main()
{
int opengenus = 1;
void* address = (void *)&opengenus;
printf("%p\n", address);
return 0;
}
Output:
0x7ffc486e6a4c
Get address of array elements
In array, we know that elements are placed side by side in memory. By getting the address of the elements we can verify this point in C.
Consider this example:
#include <stdio.h>
int main()
{
int opengenus[] = {1, 3, 2};
int length = 3;
for(int i = 0; i < length; i++)
printf("Address %d: %p\n", i, (void *)&opengenus[i]);
return 0;
}
Output:
Address 0: 0x7ffd74a61300
Address 1: 0x7ffd74a61304
Address 2: 0x7ffd74a61308
Note that addresses are increasing by 4, this is because the size of integer is 4 bytes. If we had taken a different data type, the increment will be different and same as that of the size of the datatype.
If we set length to 5 (that is wrongly), it will overflow. Consider this example:
#include <stdio.h>
int main()
{
int opengenus[] = {1, 3, 2};
int length = 5;
for(int i = 0; i < length; i++)
printf("Address %d: %p\n", i, (void *)&opengenus[i]);
return 0;
}
Output:
Address 0: 0x7ffd1a0e7b00
Address 1: 0x7ffd1a0e7b04
Address 2: 0x7ffd1a0e7b08
Address 3: 0x7ffd1a0e7b0c
Address 4: 0x7ffd1a0e7b10
Note that even on overflowing it is following the step size of 4. Note that the addresses are in hexadecimal format.
Get address of Struct elements
We know that the address of the struct and its elements are not contiguous.
Consider this struct with 2 fields:
struct opengenus
{
int og_data;
float og_data_2;
};
We will print the address of this struct and the 2 fields and observe it deeply.
Consider this code:
#include <stdio.h>
#include <stdlib.h>
struct opengenus
{
int og_data;
float og_data_2;
};
int main()
{
struct opengenus* og = (struct opengenus*)malloc(sizeof(struct opengenus));
og->og_data = 1;
og->og_data_2 = 2.0f;
printf("Address of struct: %p\n", (void *)&og);
printf("Address of struct data 1: %p\n", (void *)&og->og_data);
printf("Address of struct data 2: %p\n", (void *)&og->og_data_2);
return 0;
}
Output:
Address of struct: 0x7fff177b9090
Address of struct data 1: 0x218d260
Address of struct data 2: 0x218d264
Note that the address of the fields are contiguous but the address of the struct is far off. This is because struct is a pointer to a memory location which stores the elements. The pointer of struct is stored separately.
Get address of Linked List elements
We know that
We define the Node as a struct as:
struct Node
{
int data;
struct Node* next;
};
We have used a function to traverse through the elements of linked lists and print the address of each element.
As we allocate the memory for each element in our main function if the system needs to allocate memory to other process in between, memory addresses will not be contiguous. To illustrate this, we have added an extra line of code to allocate some memory to show that linked list elements are not contiguous.
void* a = (void *)malloc(100);
Consider this code:
#include <stdio.h>
#include <stdlib.h>
// Node of Linked List
struct Node
{
int data;
struct Node* next;
};
void printAddress(struct Node* n)
{
int i = 1;
while (n != NULL)
{
printf("Element %d :%p\n", i, (void *)&(n->data));
n = n->next;
++i;
}
}
int main()
{
// 3 elements in linked list
struct Node* opengenus_1 = (struct Node*)malloc(sizeof(struct Node));
void* a = (void *)malloc(100); // random allocation
struct Node* opengenus_2 = (struct Node*)malloc(sizeof(struct Node));
struct Node* opengenus_3 = (struct Node*)malloc(sizeof(struct Node));
opengenus_1->data = 1;
opengenus_1->next = opengenus_2;
opengenus_2->data = 3;
opengenus_2->next = opengenus_3;
opengenus_3->data = 2;
opengenus_3->next = NULL;
printAddress(opengenus_1);
return 0;
}
Output:
Element 1 :0x1dde260
Element 2 :0x1dde2f0
Element 3 :0x1dde310
Note that the difference is address between element 1 and 2 is 120 while for element 2 and 3, it is 20 bytes.
Hence, this shows that the memory address of Linked List elements are not contiguous.
With this, you have the complete knowledge of getting the address of any variable in C. Enjoy!