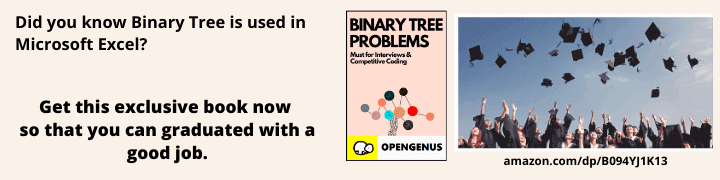
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 25 minutes
In this article we'll go through the Docstrings in Python. Docstrings stand for Documentation Strings.
Docstrings like comments provide us a convenient way of associating documentation to the source code. Unlike comments docstrings are not stripped but are retained through out the runtime of the program. They specify what the function does not how it does. Docstrings are used in functions, classes etc.
Docstrings Declaration
The docstrings are declared using a triple double quotes like """Docstring""". The docstring should should begin with a capital letter and end with a period.
"""Docstring."""
Accessing the Docstrings
The docstrings are accessed using the __ doc __ method or using the help() function.
Using the __ doc __ Method
>>> def my_doc():
"""This is a docstring"""
return None
>>> print(my_doc.__doc__)
This is a docstring
Using help() function
>>> def my_doc():
"""This is a docstring"""
return None
>>> help(my_doc)
Help on function my_doc in module __main__:
my_doc()
This is a docstring
Types of Docstrings
There are two types of docstrings. They are
- One line docstrings
- Multi line docstrings
One Line Docstrings
One line docstring fits in one line. In a one line docstring the opening and closing triple quotes lie on the same line.
>>> def square(a):
"""returns the square of arg"""
return a*a
>>> print(square.__doc__)
returns the square of arg
Multi Line Docstrings
In a multi-line docstring the first line contains the summary of the docstring and the second line should be blank seperating the summary from the rest. The summary can be in the same line of the quotes or in the next line.
>>> def myfunc(arg):
"""
1st line contains the summary.
arg means argument.
This function returns nothing.
"""
return None
>>> print(myfunc.__doc__)
1st line contains the summary.
arg means argument.
This function returns nothing.
Docstrings in classes
Docstrings are also used in classes. In classes the docstrings are placed immediately after the class or class method indented by one level.
To access the Class Docstring
>>> class square:
"""This is a class for finding the perimeter of a square"""
def __init__(self,num):
"""
The constructor for complex number class.
Parameters:
num(int):The side of the square
"""
def perimeter(self):
"""
The function to calculate the perimeter.
Parameters:
self: The side of the square.
Returns the perimeter of te square.
"""
p = 4 * self.num
return square(p)
>>> help(square) #To access the class docstring
Help on class square in module __main__:
class square(builtins.object)
| square(num)
|
| This is a class for finding the perimeter of a square
|
| Methods defined here:
|
| __init__(self, num)
| The constructor for complex number class.
|
| Parameters:
| num(int): The side of the square
|
| perimeter(self)
| The function to calculate the perimeter.
|
| Parameters:
| self: The side of the square.
|
| Returns the perimeter of te square.
|
| ----------------------------------------------------------------------
| Data descriptors defined here:
|
| __dict__
| dictionary for instance variables (if defined)
|
| __weakref__
| list of weak references to the object (if defined)
To access the class docstring we used the help() function and passed the class name square as the argument.
To access the Method's Docstring
>>> class square:
"""This is a class for finding the perimeter of a square"""
def __init__(self,num):
"""
The constructor for complex number class.
Parameters:
num(int):The side of the square
"""
def perimeter(self):
"""
The function to calculate the perimeter.
Parameters:
self: The side of the square.
Returns the perimeter of te square.
"""
p = 4 * self.num
return square(p)
>>> help(square.perimeter) #To access the method's docstring
Help on function perimeter in module __main__:
perimeter(self)
The function to calculate the perimeter.
Parameters:
self: The side of the square.
Returns the perimeter of the square.
Here, to access the method's docstring we used help() function and passed the method square.perimeter as the argument.
Indentation in Docstrings
Any indentation in the first line of the docstring is insignificant and removed. Relative indentation of the later lines of the docstring is retained. Blank lines should be removed from the beginning and from the end of the docstring.