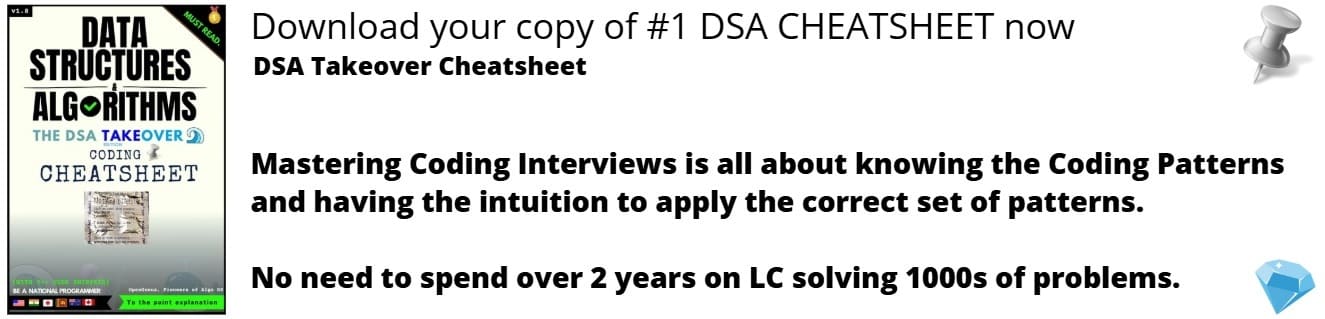
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Gatsby.js is a full-stack front-end framework that is based on React and GraphQL. Gatsby.js comes natively packaged with GraphQL, alongside some additions to it. GraphQL might be suitable for your workflow.
GraphQL is a specification for querying data. Gatsby always uses GraphQL when querying data. On top of that, Gatsby can directly call GraphQL APIs.
Table of contents
- Why Gatsby.js uses GraphQL
- GraphiQL
- How GraphQL queries work the overview
- Ways to use the queries with your Gatsby site
- The gatsby-node.js file
- Page templates
- Demo Example
- Setup
- The data layer
- Pages without a data layer
- Pages with a data layer
- Pages with GraphQL as the data layer
Why Gatsby.js uses GraphQL
Gatsby uses GraphQL to simplify the process of manipulating data and using data. GraphQL removes a lot of the hassle with data manipulation, and so overall can improve one's workflow. With this data-first approach, Gatsby can also be optimal for SEO and create lightning-fast data queries.
If Gatsby decided that they did not want to include GraphQL together in their framework, then it would be left up to the user to find a data management tool if they need it. Thus, if you don't think you will need GraphQL as your project doesn't require much data manipulation, then Gatsby.js may not be the best fit. Often, even in a small setup, GraphQL can help make your work more consistent as you are calling for data from a central location rather than having to hard-code it each time. In the case where you do find yourself needing to use a lot of data and have the need to manipulate and search that data, then Gatsby.js would be an even better fit as it comes built with it and also has some optimizations and quality of life improvements that will give you a better workflow.
GraphiQL
One of the significant quality of life improvements that comes with Gatsby.js is the GraghiQL editor. When you start the developer server, it will also host a GraqhiQL instance. GraqhiQL is a browser-based GraphQL IDE that contains the data for your currently hosted website. It also helps you learn the basics and understand how to form, create, and structure your queries so that you get precisely what you want, down to the order and the amount.
Example query
Through the GraqhiQL IDE, you can learn how to the perfect queries and see what the response JSON will be.
An example from the later demo
How GraphQL queries work the overview
Lets do an analysis of the query
First, we start with the query name, which here is MyQuery
Then we list the next field, which here is edges
Then we repeat this using the following fields edges, then node, and finally, within the node field, we want to see the id variable.
Then, we add a filter and order to the query on top of the fields. As shown in the below example
In this query, we first added a limit for three results. Then we sorted it by id in descending order. The filters and orders are placed in the topmost field.
Ways to use the queries with your Gatsby site
When accessing an external or internal data source, you can use GraphQL queries to query them.
For example, when creating a layout component, you could make a query to your site metadata to get the title, as shown below.
This would also require the following to be in your gatsby-config.js file, which is where you configure your gatsby project.
Specificly the highlighted part as that is what is being referred to in the query
What the compoent results in:
A simple line of text with the title. This is just an example, and your project can be vastly more complicated, which is where the advantages would become more apparent.
The gatsby-node.js file
In Gatsby, the gatsby-node.js is a way to use Gatsby's Node API. The code in this file is run at the start of creating the website when first hosting it. These APIs allow you to create pages dynamically using data, adding data, responding to events, and more. One prime example of when you may want to use this is with a blog. You could use the createPage API, create a template, then simply input some data, and your website will automatically create the corresponding page that will go along with it.
Below is an examaple of a gatsby-node.js file that will be used in the later example
Breaking down what is shown in the image:
First we define a function createPages
The parameters are what API we want to use. In our case we can to use the action: createPage.
Then inside the function we have two actions of createPage each filled with different parameters.
This is the start of what is needed to automate the production of pages.
Page templates
The page templates are simply another React component and work the same way. Meaning if you wanted, you could do a GraphQL query in them.
In the previous example we made the createPage action require a template as highlighted in the below picture
There also is another example in the other createPage action
This is the highlighted template that was required from the previous example
Demo Example
Setup
First we have to create the project which we can do with the gatsby-cli with the following command:
gatsby new graph-ql-demo https://github.com/gatsbyjs/gatsby-starter-hello-world
If you don't have the gatsby-cli you can install it with
npm install gatsby-cli
Now for some setup we are going to create a templates folder inside the src with the files inside: no-data.js, and with-context.js
Next we are going to create a gatsby-node.js file on the same level as the src(not in the src)
Then we will also make a data folder on the same level as the src. Then within the data folder create a file called cats.json
With the following in cats.json
[
{
"breed": "Russian-Blue",
"color": "Gray",
"description": "Average sized gray cat."
},
{
"breed": "American-Shorthair",
"color": "Varies",
"description": "Nice and cute cat."
},
{
"breed": "Bombay-Cat",
"color": "Black",
"description": "Nice cat with yellow eyes and black fur."
}
]
You will also need to add some plugins to allow GraghQL to parse JSON, gatsby-source-filesystem, and gatsby-transformer-json. You can add them by adding the following to your gatsby-config.js file.
Then running the following command
npm install gatsby-source-filesystem gatsby-transformer-json
The data layer
- Pages without a data layer
Within the no-data.js file let us define a simple component with hardcoded data so that there is no passing of data.
Then within the gatsby-node.js file let us use the createPage action to generate a page from the template.
We create a function called createPages and we have it take the parameters to create a page.
Then inside the createPage we define a path, and a required component
When the program is running, it will generate the pages with the provided paths and the component. Any pages created this way will all look the same. This example likely wouldn't be used as there is no data passing.
- Pages with a data layer
Next the setup for the data layer is very similar just we pass in pageContext and use it within our component
Here we have one extra attribute with the context where you put the content you want to be shown.
- Pages with GraphQL as the data layer
First we have to create a query to query the cats.json so that we can get the data that we want to use to generate our pages with.
query catQuery {
allCatsJson {
edges {
node {
breed
color
description
}
}
}
}
But since we only want the breed, and description right now we will change it to just
query catQuery {
allCatsJson {
edges {
node {
breed
description
}
}
}
}
Now incorparating it into the gatsby-node.js file we have
exports.createPages = async ({ actions: { createPage } }) => {
const results = await graphql(`
{
allCatsJson {
edges {
node {
breed
description
}
}
}
}
`)
results.data.allCatsJson.edges.forEach(edge => {
const cats = edge.node
createPage({
path: `/gql/${cats.breed}/`,
component: require.resolve("./src/templates/with-context.js"),
context: {
h1: cats.breed,
p: cats.description,
},
})
})
Notice how the function is now async. This is because we have to wait as this is run during the startup, so GraphQL may not yet be ready when the gatsby-node.js is initially ready.
We have now also created pages using data from GraphQL queries.