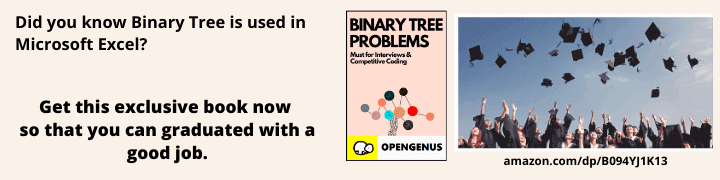
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explained the idea of reading and writing JSON file in Python with a complete Python implementation example.
Table of contents:
- Introduction to JSON
- Example of a JSON file
- How to write to JSON file
- How to read a JSON file
Introduction to JSON
JavaScript Object Notation (JSON) is a data-interchange format. It is lightweight and easy to be interpreted and written by both humans and machines.
Example of a JSON file
Each key is map to a value such as "Name": <John Doe>
. Below is an example of how a JSON file will look like.
{
"user": [
{
"Name": "John Doe",
"Phone": "11123456",
"Email": "john@email.zxy"
},
{
"Name": "Tom Cobley",
"Phone": "22234567",
"Email": "tom@email.zxy"
}
]
}
How to write to JSON file?
Let review the following code on how to use Python to write to JSON file. We first need to import two modules, json
for accessing JSON files and pathlib
which provides an object API for working with files and directorie.
import json
from pathlib import Path
Next we specify the folder path and filename of the JSON file that we wish to work on.
data_folder = Path("data/")
file_to_open = data_folder / "contact.json"
We then create a add_contact
function which take in two inputs, the contact details that we wish to add in the JSON file and the JSON filenames. Note that we have set the indent=2
to prettify the JSON file for better readability by humans.
def add_contact(contact, filename):
with open(filename, "w") as f:
json.dump(contact, f, indent=2)
Next we request the user to input the contact details, such as name, phone and email.
print(f"Adding contacts...")
name = input("Name please ")
phone = input("mobile no. ")
email = input("Email ")
contact = {
"Name": name,
"Phone": phone,
"Email": email
}
We will do a check if there is an existing JSON file, if yes, we will want to append the user input to the JSON file, else a new file will be created instead.
if Path(file_to_open).is_file():
# print("file exist")
with open(file_to_open, 'r+') as f:
contact_record = json.load(f)
contact_record["user"].append(contact)
add_contact(contact_record, file_to_open)
else:
# print("file not found")
contact = {
"user": [
{
"Name": name,
"Phone": phone,
"Email": email
}
]
}
add_contact(contact, file_to_open)
Here is the entire code block for reference
import json
from pathlib import Path
data_folder = Path("data/")
file_to_open = data_folder / "contact.json"
def add_contact(contact, filename):
with open(filename, "w") as f:
json.dump(contact, f, indent=2)
print(f"Adding contacts...")
name = input("Name please ")
phone = input("mobile no. ")
email = input("Email ")
contact = {
"Name": name,
"Phone": phone,
"Email": email
}
if Path(file_to_open).is_file():
# print("file exist")
with open(file_to_open, 'r+') as f:
contact_record = json.load(f)
contact_record["user"].append(contact)
add_contact(contact_record, file_to_open)
else:
# print("file not found")
contact = {
"user": [
{
"Name": name,
"Phone": phone,
"Email": email
}
]
}
add_contact(contact, file_to_open)
How to read a JSON file?
We need to import two modules, json
for accessing JSON files and pathlib
which provides an object API for working with files and directorie.
import json
from pathlib import Path
Next we specify the folder path and filename of the JSON file that we wish to work on.
data_folder = Path("data/")
file_to_open = data_folder / "contact.json"
We then create a `read_contact` function which take in one input which is the JSON filenames.
```python
def read_contact(filename):
with open(file_to_open, 'r') as f:
contact_record = json.load(f)
print (contact_record)
We then call the read_contact()
function to execute our codes
read_contact(file_to_open)
The output will be as follow
{'user': [{'Name': 'John Doe', 'Phone': '11123456', 'Email': 'john@email.zxy'}]}
Here is the entire code block for reference
import json
from pathlib import Path
data_folder = Path("data/")
file_to_open = data_folder / "contact.json"
def read_contact(filename):
with open(file_to_open, 'r') as f:
contact_record = json.load(f)
print (contact_record)
read_contact(file_to_open)
With this article at OpenGenus, you must have the complete idea of Reading and Writing JSON file in Python.