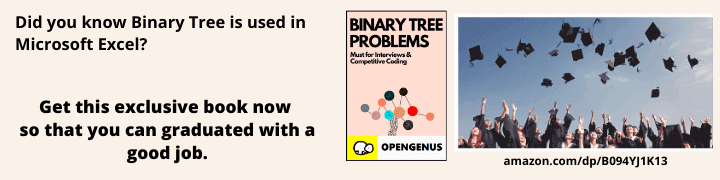
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explained the idea of using Python to read an email from Gmail with a complete Python implementation example.
Table Of Contents:
- Introduction to the email, and impalib modules, IMAP4 SSL protocol, RFC822 standard
- Generate gmail app code
- Config file setup
- Example in Python
Introduction to the email, and impalib modules, IMAP4 SSL protocol, RFC822 standard, email headers
Python email module
The email package is a library for managing email messages. It is specifically not designed to send email messages which is where impalib comes in and allows us to send and read and do much more with the emails
Email Headers and how to apply it with the email module
Email headers are the metadata for the email. It contains information such as the sender email, who it is for, the subject, body, date, content-type/content-disposition, message-id, and MIME version. The metadata can also store many other things.
The information we will be using in this demonstration is the subject, body, content-disposition, and sender. When creating an email, the subject is the topic/title that the sender assigns in a subject line. The body is the message of the email. The sender is who sent the email. Finally, the content-disposition is the disposition of the email's content, which can be separated as content to a web page, part of a web page, or an attachment.
Python impalib module
IMAP stands for Internet Mail Access Protocol, and it is an email retrieval protocol that we will use to access data in our email using a Python application. IMAP typically does not download the emails. It just reads them and displays them.
IMAP4 SSL protocol
IMAP, Internet Mail Access Protocol version 4, is a protocol for storing and retrieving messages from Simple Mail Transfer Protocol (SMTP) servers.
SSL (Secure Sockets Layer) is an encryption-based Internet security protocol. It was first developed to ensure privacy, authentication, and data integrity in Internet communications. SSL is the predecessor to the modern TLS (Transport Layer Security), a newer protocol that fixes some of the issues SSL had. If a website has SSL/TLS, the URL will show as HTTPS rather than HTTP to show that it is secure.
RFC822 standard
RFC822 standard is the format of ARPA Internet text messages. This standard defines a syntax for text messages sent via emails. This format consists of header fields, the subject and sender, and an optional message body.
Example of the format:
From: example@example.com
To: someone_else@example.com
Subject: An RFC 822 formatted message
This is the plain text body of the message. Note the blank line
between the header information and the body of the message.
Generate gmail app code
Go to myaccount.google.com/security, and make sure you have enabled 2-Step Verification.
Next, select the following fields, you may change the second field if your platform is different.
Store the password that is provided in the following part
Config file setup
Create an email_config.py file with the following
gmail_pass = "[this will be the App password you generated in the previous step]"
user = "[replace it with your gmail address, for example hello-world@gmail.com]"
host = "imap.gmail.com"
Example in Python
Imports
import email
import imaplib
from email_config import gmail_pass, user, host
Define the function with the parameters to decide how many emails to show, and if to contain the body of the email or not, both with defaults.
def read_email_from_gmail(count=3, contain_body=False):
Create server and login
mail = imaplib.IMAP4_SSL(host)
mail.login(user, gmail_pass)
Using select to chose the e-mails from the inbox section, the res is to catch string literal the mail.select also returns.
res, messages = mail.select('INBOX')
Total number of emails. Used for the range when iterating over the messages
messages = int(messages[0])
Iterating over the emails and printing the messages
for i in range(messages, messages - count, -1):
# RFC822 protocol
res, msg = mail.fetch(str(i), "(RFC822)")
for response in msg:
if isinstance(response, tuple):
msg = email.message_from_bytes(response[1])
# Store the senders email
sender = msg["From"]
# Store subject of the email
subject = msg["Subject"]
print("-"*50) # To divide the messages
print("From : ", sender)
print("Subject : ", subject)
Store Body. This is a more complicated then storing the subject or the senders email as it can come in different content types.
body = ""
temp = msg
if temp.is_multipart():
for part in temp.walk():
ctype = part.get_content_type()
cdispo = str(part.get('Content-Disposition'))
if ctype == 'text/plain' and 'attachment' not in cdispo:
body = part.get_payload(decode=True) # decode
break
else:
body = temp.get_payload(decode=True)
# Add to the print section
if(contain_body):
print("Body : ", body.decode())
Final Code:
import email
import imaplib
from email_config import gmail_pass, user, host
def read_email_from_gmail(count=3, contain_body=False):
# Create server and login
mail = imaplib.IMAP4_SSL(host)
mail.login(user, gmail_pass)
# Using SELECT to chose the e-mails.
res, messages = mail.select('INBOX')
# Caluclating the total number of sent Emails
messages = int(messages[0])
# Iterating over the sent emails
for i in range(messages, messages - count, -1):
# RFC822 protocol
res, msg = mail.fetch(str(i), "(RFC822)")
for response in msg:
if isinstance(response, tuple):
msg = email.message_from_bytes(response[1])
# Store the senders email
sender = msg["From"]
# Store subject of the email
subject = msg["Subject"]
# Store Body
body = ""
temp = msg
if temp.is_multipart():
for part in temp.walk():
ctype = part.get_content_type()
cdispo = str(part.get('Content-Disposition'))
# skip text/plain type
if ctype == 'text/plain' and 'attachment' not in cdispo:
body = part.get_payload(decode=True) # decode
break
else:
body = temp.get_payload(decode=True)
# Print Sender, Subject, Body
print("-"*50) # To divide the messages
print("From : ", sender)
print("Subject : ", subject)
if(contain_body):
print("Body : ", body.decode())
mail.close()
mail.logout()
read_email_from_gmail(3, True)
Sample output
One thing to note is that many modern day emails contain images and other content that may not problerly be shown.
--------------------------------------------------
From : Demo <demo_email@gmail.com>
Subject : Demo Subject
Body : This is a demo body
This is the next line
and the line after that
and the line after that
and the line after that
--------------------------------------------------
From : Demo <demo_email@gmail.com>
Subject : Second Demo Subject
Body : This is a demo body
This is the next line
and the line after that
This is for a demo
--------------------------------------------------
From : Demo2 <demo_email2@gmail.com>
Subject : Third Demo Subject
Body : This is a demo body
This is the next line
and the line after that
This is for a demo
With this article at OpenGenus, you must have the complete idea of how to read email from Gmail.