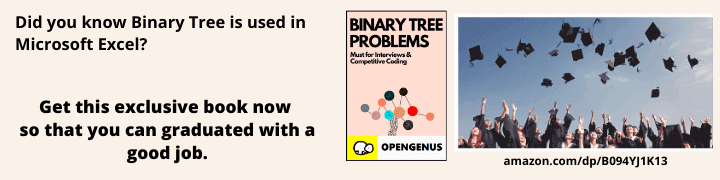
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article we are going to talk about the static variables in C.
Here we will be talking about:
- Introduction to static variables
- Difference between static and automatic variables
- Important points related to static variables
Before we get ahead with our topic first lets take a quick view on -
Some important terms related to C programming language
- Variable -A variable is used to store some value and points to a specific memory location.
- Scope -The region of program where you can access a particular variable is called the scope of that variable.
Introduction to Static variables
Before diving into the topic, lets begin by answering a simple question-
What are static variables?
Static variables are the variables that persist their values within their scope.
You need to initialize the static variables only once. You can access and use the static variables within the block in which it is declared.
The word "static" is itself very self explanatory due to the fact that once initialized, the variable continues to be in memory throughout the execution of the program. It remains "static" in the sense that it isn't deleted after you exit a particular block, loop or function.
General syntax of static variables in C language
static datatype varaiable_name = value;
In C programming language we have:
- static variable.
- automatic variable.
- local variable.
- global variable.
- external variable.
In order to understand how static variable differs from the rest, we need keep in mind some important points.
Memory Allocation for static variables in C
Static allocation happens when we declare a static variable. Each static variable defines one block, or we can say a fixed size.
When the program is started, the static memory allocation takes place in the case of static variables. This memory space is not freed even after the execution of the block in which the static variable is declared.
That is why the static variable can easily retain its value throughout the execution of the whole program and you don't have to worry about it getting erased from the memory after the function or block in which it is declared gets executed.
Difference between automatic variable and static variable
Static variable remains in memory while the program is running even if it is declared within a block.
On the other hand automatic variables get destroyed after the completion of the function in which they were declared.
Hence, it is possible to retain the value of static variables but in the case of automatic variables, you can not access them after the execution of the block or the function in which it is declared ends.
Important points
- Just like global variables, default value of static variables is zero.
That means if we don't assign any value to the static variable, it will automatically be assigned to zero as the default value.
When the static variables are initialized, they are stored in the data segment of the program's address space. And when they are uninitialized, they are stored in the BSS segment.
In the BSS segment the uninitialized variables are given zero as the default value and hence the default value of static variables is zero.
Here is an example to illustrate this fact -
#include <stdio.h>
int main(){
static int a;
printf("The value of a is %d",a);
return 0;
}
Output-
The value of a is 0
In the above example, static variable a is declared but is uninitialized. When we try to print the value of a, zero is printed on the output screen, which is the default value for any uninitialized static variable in C.
As the static variable is uninitialized, it will be stored in the BSS segment therefore this output is expected.
-
But it is important to note that the static variable cannot be accessed outside the program. Unlike the global variables, static variables are available only in the file in which they are declared.
-
Static variable if declared outside the scope of any function will act like a global variable but only within the file in which it is declared. Even if you try to access some static variable in a different program file using the extern keyword, it will produce an error during the compilation.
This is one of the main differences between static and global variables. -
You can only assign a constant literal to a static variable.
If you try to assign anything else than a constant literal than it will produce an error. Here is an example to illustrate this fact-
static int a;
a= 5;
Here in the above code you cannot assign another variable to the static variable.
It has to be some constant literal value.
Conclusion
- Static variables are available within the scope in which they are declared.
- Static allocation of memory takes place in case of static variables in C.
- When initialized, static variables are stored in data segment else in the BSS segment in case they are uninitialized.
- The default value for static variables is zero.
- You can assign only constant literals to a static variable.
- Static variables cannot be accessed outside the program file.
- Unlike automatic variables, static variables persist their values even after the execution of the block or the function in which they are declared.