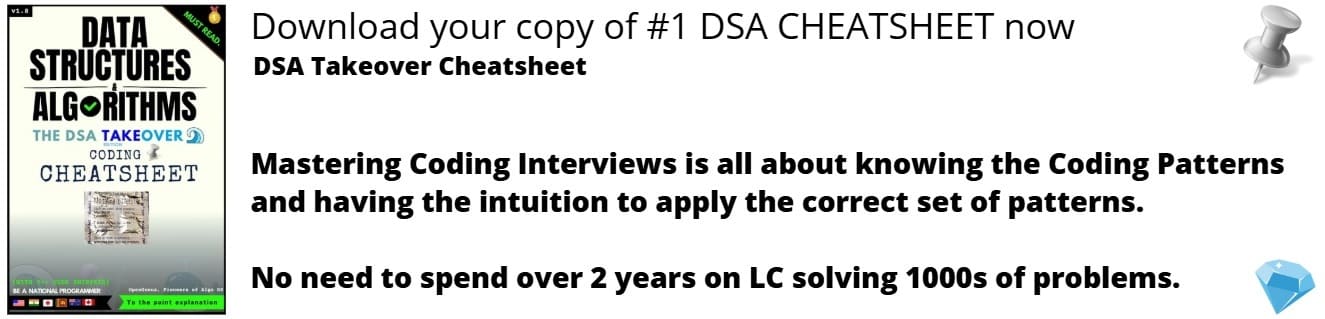
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explored the idea of strict mode in JavaScript that is the statement "use strict" in depth.
Table Contents
1. Introduction
2. How to use
2.1. File level
2.2. Function level
3. Changes
3.1. With
3.2. Global variables
3.3. Restrictions
3.4. This
3.5. Properties and Parameters
3.6. Octal System
4. Final Considerations
1. Introduction
Using strict or strict mode was a directive in ECMAScript version 5. In this directive, JavaScript code is strictly interpreted. In other words, errors previously silenced are pointed out, such as:
- use of an undeclared variable;
- use of reserved words in the code;
- absolute resources;
- parts of the language classified as problematic.
A strict mode is a tool that helps in code development (better quality and detecting bugs early).
2. How to use
It is possible to use strict mode in different scopes:
2.1. File level
In this scope, the string use strict is placed at the beginning of the JavaScript code file. Above this command, it is only allowed to put comments or whitespace.
If a piece of code is placed above the command (use strict), the strict mode will not work.
The example in Figure 1, illustrates where the command is placed in the code. The code result is 10 letters (referring to the number of letters in the JavaScript word).
"use strict";
let str = "JavaScript";
console.log(str.length);
"use strict";
Figure 1. Using strict mode within JavaScript code.
4.2. Function level
Within the Function level, use strict command is placed inside the function.
This way, only the function containing this command is executed in strict mode. And the rest of the code is normally implemented.
Figure 2 exemplifies the three functions, in which the first and second are in strict mode and the third without restrictions.
The message that returns to strict mode functions: I am a strict mode function! And me too!
And for the not strict function: I'm not a strict mode function!
function strict(){
'use strict';
function strict2() {
return " And me too!";
}
return "I'm a strict mode function!" + strict2();
}
function notStrict() {
return "I'm not a strict mode function!";
}
console.log (strict());
console.log(notStrict());
Figure 3. Example of a strict mode within the code.
3. Changes
Strict mode brought several changes to the syntax and behavior of code execution. However, the article will focus on the main parts. If you want a breakdown of all the changes, you can refer to Soshnikov's work.
3.1. With
Removed with command from language. When using it in strict mode, it generates a syntax error (confusing bugs and compatibility issues).
Figure 4 exemplifies the use of "with" without strict mode. The code returns the result 1 2 3.
with ({k: 1}) {
with ({w: 2}) {
with ({y: 3}) {
console.log(k);
console.log(w);
console.log(y);
}
}
}
Figure 4. Using the "with" in JavaScript language.
Figure 5 uses the same code in Figure 4 but in strict mode. There was no result return, generating a syntax error.
"use strict";
with ({k: 1}) {
with ({w: 2}) {
with ({y: 3}) {
console.log(k);
console.log(w);
console.log(y);
}
}
}
Figure 5. Using the "with" in strict mode.
3.2. Global variables
Without strict mode, it is possible to create a global variable whenever we assign a value to an undeclared variable. Within strict mode, this implicit declaration generates an error.
The code in Figure 6, in strict mode, returned no result.
Removing the strict mode from the code will generate the result: JavaScript Python Kotlin.
"use strict";
variableTest = 'JavaScript';
console.log(('value: ' + variableTest));
function1();
console.log(('value: ' + variableTest));
function2();
console.log(('value: ' + variableTest));
function function1() {
variableTest = 'Python';
}
function function2() {
variableTest = 'Kotlin';
}
Figure 6. Implicit declaration of a global variable in strict mode.
3.3. Restrictions
Restricted mode imposes restrictions on various variables, functions, and parameter names.
As an example, identifiers such as eval() and arguments() are no longer used as identifiers or for assigning values. However, this is no loss, as the JavaScript language natively has an eval function (currently JSON is natively implemented in browsers) and an arguments object.
In Figure 7, an error occurs in the strict mode because the variable is not defined. In the absence of strict mode, the code returns the value ECMAScript.
"use strict";
eval('var str="ECMAScript";');
console.log ((str));
Figure 7. Using eval() within the strict mode.
In Figure 8, the code returns an error.
The arguments() cannot assign values. However, in the absence of strict mode, it returns the result 3.14.
"use strict";
let arguments = 3.14;
console.log(arguments)
}
Figure 8. Using arguments() within the strict mode.
Other words should also be avoided as identifiers because they may be future language features: implements, yield, interface, static, let, public, package, protected and private.
3.4. This
This word inside the function points to the object that contains the function. However, when the function does not belong to a specific object, this points to the global object.
In restricted mode, when inside a function defined in the global scope, it returns the value undefined (Figure 9).
"use strict";
function functionTest() {
console.log((this));
}
functionTest();
Figure 9. Strict mode code within a globally scoped function.
However, if the function belongs to an object, it continues to point to the object.
In the example in Figure 10, the code returns the value JavaScript and Java
"use strict";
const language = {
str1: "JavaScript",
str2: "Java",
data : function() {
return this.str1 + " and " + this.str2;
}
};
console.log(language.data());
Figure 10. Code in strict mode with role belonging to the object.
3.5. Properties and Parameters
In strict mode, declaring two properties of an object or parameters with the same name is not allowed. This mode forces the use of unique property and parameter names. Otherwise, it generates a syntax error.
In Figure 11, repeated use of the k property generates an error in strict mode.
If it was not in restricted mode, the code returns {k: 43, y: 90}.
"use strict";
str = {
k: 5,
y: 90,
k: 43
}
console.log(str)
Figure 11.Code in strict mode with repetition of property k.
The syntax error occurs in the example in Figure 12, with the repetition of parameter p1. In the absence of strict mode, the code returns the value of 25.
function functionTest(p1, p1) {
"use strict";
return p1 * p1;
}
console.log(functionTest(2, 5));
Figure 11.Code in strict mode with repetition of parameter p1.
3.6. Octal System
Numbers in the octal system have base 8 and representation with zero in front of the number.
This case created a lot of confusion because many felt that a leading zero would make no difference when representing numbers.
In strict mode, the use of the octal system is not allowed.
If a zero is in front of a valid octal number, a syntax error occurs. Otherwise, the number is a decimal.
In Octal System, the number 19 is equivalent to 023. In the code of Figure 12, in strict mode, f1 generates an error. However, in the absence of strict mode, it returns the decimal value of 19.
"use strict";
var f1 = 023;
console.log(f1);
Figure 12.Code in strict mode in Octal System.
4. Final Considerations
Generally, strict mode enables on all browsers. If any browser does not interpret the "use strict", it will treat the strict mode as a string and not affect the code implementation. Strict mode improves code stability and readability, decreasing bugs and improving security in implementation.
With this article at OpenGenus, you must have the complete idea of Strict mode in JavaScript.