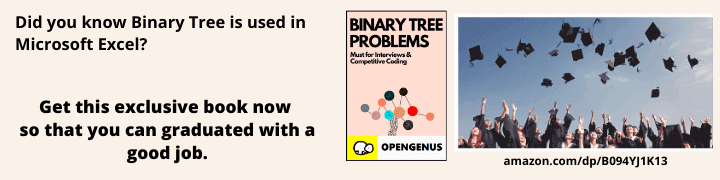
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explored everything about strings in JavaScript including core methods to get length of string, remove character and much more.
Table Contents
1. Introduction
2. String Properties
2.1. Length
2.2. Replacement
2.3. Extraction
2.4. Concatenation
2.5. Conversion
2.6. Removal
2.7. Filling
2.8. Extraction
2.9. Verification
2.10. Converting String to Matrix
3. Final Considerations
Reference
1. Introduction
JavaScript, like any other language, has different types of pre-built data primitive data). These data represent something that is part of our reality as numbers and words within a code.
In the article, we will work the String variable within the JavaScript language. However, viz., there are seven primitive data types:
- Boolean, the variable can assume the values true and false;
- Null, represents nothingness or emptiness, being able to empty an object;
- Undefined, similar to null. However, used when:
a) creating an empty variable;
b) to empty an object;
c) a function returns no value.
The null and undefined are equal in value but different in type. Undefined is undefined itself, and the null is an object;
- Number, its use allows the use of numerical values to build algorithms;
- BigInt, represents non-exact precision integers. Remembering that it is not possible to use numbers of the type number and bigInt in the same expression, as they are of different types;
- String, represents the characters inside the code;
- Symbol, represents immutable unique primitive values and can be used as a key to an Object (Data and Access Properties).
2. String Properties
In the JavaScript language, you can get properties, characteristics, and information from variables of type String within code:
2.1. Length
Using the length property allows us to obtain information about the length of the string. In the example in Figure 1, for the OpenGenus keyword, the length result is nine letters.
let text = "OpenGenus";
console.log(text.length);
Figure 1. Length property in JavaScript language.
2.2. Replacement
The replace() method replaces one value with another without changing the string but returns a new string. This method is case sensitive.
For the example in Figure 2, we replaced C++ with JavaScript using the replace() command. The result is JavaScript, Kotlin.
let str = "C++, Kotlin";
let res = str.replace("C++", "JavaScript");
console.log (res);
Figure 2. Replace property in JavaScript language.
2.3. Extraction
There are three ways to extract parts of a string into code:
a) Substring ()
This method is similar to the slice(). The difference is that the substring() method does not allow negative numbers.
To extract the JavaScript keyword from the code list in Figure 3 was used positions 7 and 18.
let str = "Python, JavaScript, Java";
console.log (str.substring(7,18));
Figure 3. Substring() method in JavaScript language.
b) Slice ()
In this method, there are two important parameters (start position and end position).
Part of the string is extracted and returned in a new.
To extract the portion alle (position 3 and 7) from the word Parallelepiped (Figure 4), the following code was developed:
let str = "Parallelepiped";
console.log(str.slice(3,7));
Figure 4. Slice() method with positive values in JavaScript language.
If the position informed is negative, the string count starts from the end of the string. In the example in Figure 5, to return the same portion alle was adopted the values -11 and -7.
let str = "Parallelepiped";
console.log(str.slice(-11, -7));
Figure 5. Slice() method with negative values in JavaScript language.
c) Substr ()
The subtr() method is also similar to the slice(), but the second parameter specifies the length of the extracted part.
To extract the Serp portion of the code list in Figure 6 positions 13 and length 4 were adopted.
let str = "Labradorite, Serpentine, Natrolite";
console.log(str.substr(13, 4));
Figure 6. Subtr() method with positive values to extract the Serp part of the list.
And if the position informed is negative, the counting starts at the end of the string. For example, to extract the word Natrolite from the list, position -9 was adopted within the code (Figure 7).
let str = "Labradorite, Serpentine, Natrolite";
console.log(str.substr(-9));
Figure 7. subtr() method with a negative value to extract the word Natrolite.
2.4. Concatenation
In concatenation, it is possible to join two or more strings. In Figure 8 was concatenate str1 with str2, resulting in the phrase *Thanks!*.
let str1 = "Thank";
let str2 = "you!";
let res = str1.concat(" ", str2);
console.log(res);
Figure 8. Concatenation without using the operator.
Another way to concatenate strings is using the operator (+). In Figure 9, the same sentence Thank you! was created but with the operator.
let str = "Thank" + " " + "you!";
console.log(str);
Figure 9. Concatenation with operator (+).
2.5. Conversion
A string can be converted to lowercase with the command toLowerCase().
In the example in Figure 10, the word "SOFTWARE" has been converted to software.
let str = "SOFTWARE";
let res = str.toLowerCase(str);
console.log (res);
Figure 10. toLowerCase() command, converting strings from uppercase to lowercase.
Lowercase letters are converted to uppercase with the toUpperCase() command.
In Figure 11, "computer" was transformed into "COMPUTER".
let str = "computer";
let res = str.toUpperCase(str);
console.log (res);
Figure 11. toLowerCase() command, turning strings from uppercase to lowercase.
2.6. Removal
The trim() method allows the removal of unnecessary white space around the string. In the code, in Figure 12, we remove the blanks around the words, resulting in LiveScript.
let str = " LiveScript ";
console.log(str.trim());
Figure 12. Removing blanks around the word with the trim() method.
2.7. Filling
The padStart command fills the beginning of the string.
In Figure 13, we add the prefix "un" to the word "forgettable" which results in the string unforgettable.
To the process, informed total length and the prefix (13, "un").
let str = "forgettable";
console.log(str.padStart(13, "un"))
Figure 13.Using the padStart command to fill in the beginning of the string.
Another way to fill the string is at the end, with the padEnd command.
In the word "server" the suffix "less" was added, originating the string serverless (Figure 14).
For this process, the total string length was 10, and the suffix "less".
let str = "server";
console.log(str.padEnd(10, "less"))
Figure 14. Using padEnd command to fill end of string.
2.8. Extraction
Extracting a string can be done in three ways:
a) Property Access
This command enables access to the property in strings. If the value exists, it returns undefined. There are two ways to access the property:
- bracket notation, where the indicated position returns the value contained in it. In the example in Figure 15, the value of position 4 was requested, which returned the value S.
var str = "JAVASCRIPT";
console.log(str[4]);
Figure 15. Extracting information with square bracket notation.
- dot notation accesses the property as long as it is composed of Unicode letters, $, underline, and does not start with numbers.
In Figure 16, accessing the variables π, name and $, the following values were obtained, respectively: 3.1141592653589793, JavaScript and Code.
const str = {
Ï€: Math.PI,
name: 'JavaScript',
$: 'Code'
};
console.log(str.Ï€);
console.log(str.name);
console.log(str.$);
Figure 16. Extraction information with dot notation.
b) charCodeAt() method
This method returns the Unicode of the character at a specified index in a string.
For the word JavaScript, the letter J returned the Unicode 74 (Figure 17).
let srt = "JavaScript";
console.log(srt.charCodeAt(0));
Figure 17. charCodeAt method that returns character Unicode.
c) charAt() method
This method returns the character at a position (index) specified in the string. For the word feldspar, index 3 returned the value d.
var str = "feldspar";
console.log(str.charAt(3));
Figure 17. charAt method returns the value contained in the specified position.
2.9. Verification
In JavaScript, it is possible to check if a string is part of another string. For this check, there are several ways, but in this article, we will work with includes() command.
In the example sentence in Figure 18 was verified the string "Java".
If found, it generates the message String Found otherwise Not Found.
var str = "I'm studying JavaScript and Java";
if(str.includes("Java")){
console.log("String Found");
} else {
console.log("Not found");
};
Figure 18. String verification within another string.
2.10. Converting String to Matrix
The split() command converts string to array.
In the example (Figure 19), position zero returns the k value, and division is by the comma.
let str = "k, w, y, t";
const res = str.split(",");
console.log(str[2]);
Figure 19. Converting string to matrix.
3. Final Considerations
Primitive data (type String) are widely used in the JavaScript language. In this article, we present some ways to work with string within codes. However, there are still other ways to consult on the w3school website.
With this article at OpenGenus, you must have the complete idea of Strings in JavaScript.