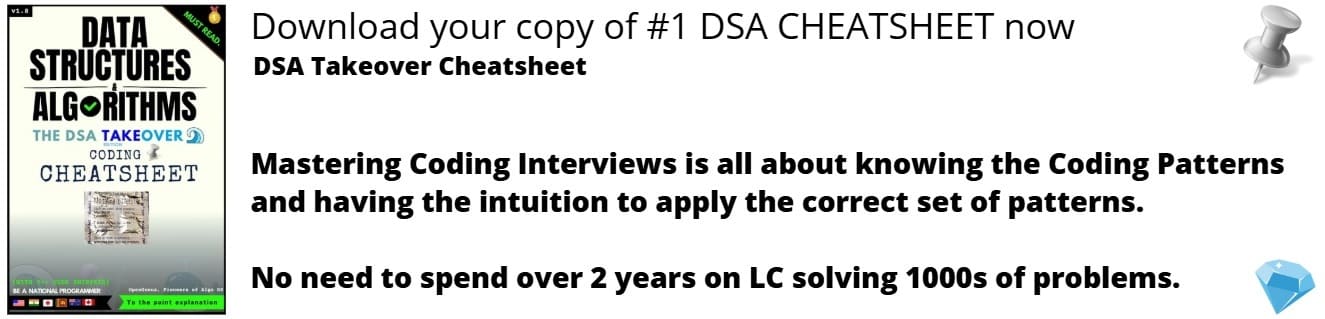
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 30 minutes
Compared to other languages, C is a low-level language. It does not have any specific data-type specific for a String. But as we all know that a String is nothing but a collection of characters. Since there is no built-in data-type in C language for String, it is handled using an array of characters. A String in C can be defined as an array of characters.
We would be working with the strings using a series of question answers.
How do we define a String in C?
We can define a String in C as
char str[arr_size];
This will create an array named str
of characters of size arr_size
.
How do we create a string with value initialized in it?
char str[]="OpenGenus";
This will create a character array named str
of size 9 which is the size of "OpenGenus". The array str
would be initialized with the values in "OpenGenus".
Another way to define string is
char str[100]="OpenGenus"
In this case, an array named arr
of size 100 would be created and only the first 9 characters would be initialized with the value in the string "OpenGenus". All the remaining characters in the array would be having an arbitrary value.
Okay, if a long array of characters is initialized with small string, how do we get to know that the string has terminated?
In a string, represented as an array of characters, the last character is represented as \0
. This represents the termination of a string. Even if an array has more characters after \0
, they are not considered to be in the string. For example:-
char str[]="OpenGenus";
str[4]='\0';
Initially, the string str
had the value as "OpenGenus". But on changing the value of the str[4]
character with \0
, now the string str
represents only "Open".
Why is \0
needed?
Consider this example of creating a string.
char str[50]="OpenGenus";
Now, in this case, it has created an array of size 50, but only the first 10 characters are assigned the characters from "OpenGenus". So, if I need to do a basic operation like printing the string or getting the string size, I need to know where the string is ending. That's when we need \0
. We can traverse through the array until I find \0
and that would be the ending of my string and no more further.
How do we print a string?
Okay, from the basic understanding of a string as an array of characters, we can print it as follows:-
#include<stdio.h>
int main(){
char str[]="Open Genus";
int i=0;
while(str[i]!='\0'){
printf("%c",str[i]);
i++;
}
}
But, there is a better way too.
Although a string does not have any built-in data-type to store sting, it has access specifier to represent a string. You can print it like this as well it as follows:-
char str[]="Open Genus";
printf("%s",str);
Here, the role of \0
becomes even more important, the string will be printed till the point it sees \0
.
For example:-
#include<stdio.h>
int main(){
char str[]="OpenGenus";
printf("%s",str);//output=>"Open Genus"
printf("\n");
str[4]='\0';//the ' ' is converted to '\0'
printf("%s",str);//output=>"Open"
printf("\n");
}
How do I take a string as input from the user?
Similar to the way, we print a string, we can also take a string as input from the user.
#include<stdio.h>
int main(){
char str[100];
scanf("%s",str);
printf("%s",str);
}
But, you might be wondering why there is no &
sign. Because whenever we take input any char
or int
we need the address of that variable. But since the string is basically an array, and an array name is written without any index, it represents the address of the array. So we don't need any &
sign.
How can we take a complete sentence as input from a user?
When you want to take a sentence as input from the user. For this you might try this:-
#include<stdio.h>
int main(){
char str[100];
printf("Enter a sentence:-");
scanf("%s",str);
printf("Sentence:-%s\n",str);
}
And you enter a sentence as input.
Enter a sentence:-open genus
Sentence:-open
We saw this behaviour because the blank space is seen as a delimited and is assumed that the string ends there.
To take a complete sentence as input:-
#include<stdio.h>
int main(){
char str[100];
printf("Enter a sentence:-");
scanf("%[^\n]s",str);
printf("Sentence:-%s\n",str);
}
Issues when we take a number as input followed by a sentence as input
But this again arises a problem when we take an integer as input followed by a sentence. For example:-
#include<stdio.h>
int main(){
int n;
printf("Enter a number:-");
scanf("%d",&n);
char str[100];
printf("Enter a sentence:-");
scanf("%[^\n]s",str);
printf("Number:-%d\n",n);
printf("Sentence:-%s\n",str);
}
But you will see some output like this:-
Enter a number:-13
Enter a sentence:-Number:-13
Sentence:-
The reason for this is, we have defined the delimiter for the sentence as \n
but when the integer is taken as input from the user, and user presses enter key, this makes \n
to be stored in buffer and seen as the first character for string and as we have said to terminated the string as \n
, it is treated as end of sentence.
You can overcome this issue by already defining that we would be getting \n
while taking the sentence as input. For example:-
#include<stdio.h>
int main(){
int n;
printf("Enter a number:-");
scanf("%d",&n);
char str[100];
printf("Enter a sentence:-");
scanf("\n%[^\n]s",str);
printf("Number:-%d\n",n);
printf("Sentence:-%s\n",str);
}
How do we pass a string to a function?
It is passed the same way as an array is passed to a function.
#include<stdio.h>
void printStr(char s[]){
printf("%s",s);
}
int main(){
char str[]="Open Genus";
printStr(str);
return 0;
}