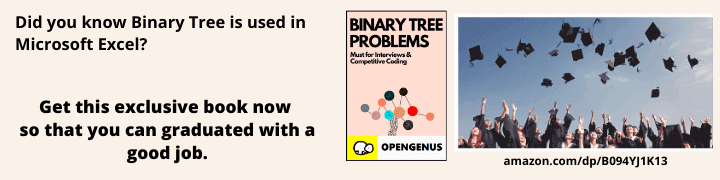
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 40 minutes
The canvas element is part of HTML5 specification and allows for the rendering of 2D shapes and bitmap images. It is a low level, procedural model that updates a bitmap.
Canvas also helps in making 2D games, animations, scenes or any graphics, in general, using JavaScript.
How to use Canvas in the basic way?
In an HTML document, we need to use canvas element as follows:
<!DOCTYPE HTML>
<html>
<head>
<style>
#canvas{ border: 1px solid black; }
</style>
</head>
<body>
<canvas id="canvas" width="300" height="300">
Canvas not supported.
</canvas>
</body>
</html>
A canvas is technically a rectangular area on an HTML page. An id attribute is required to be referred to in a script, and a width and height attribute to define the size of the canvas.
Rendering Context: to draw anything on Canvas
To draw or render anything, access to the rendering context it needed.
The canvas element has a DOM method called getContext
, used to obtain the rendering context. This function takes one parameter, the type of context2d
.
var canvas = document.getElementById("canvas"); // Get element
if (canvas.getContext) { // Check if canvas supported
var ctx = canvas.getContext('2d'); // Get rendering context '2d'
// START Drawing
...
// END Drawing
} else { // Inform, not supported
alert('Canvas not supported.');
}
How to draw a rectangle on a Canvas?
Canvas has three methods to deal with rectangles.
- fillRect() - Draws a rectangle filled with current
fillStyle
- strokeRect() - Draws a rectangle stroked (outline) with current
fillStyle
- clearRect() - Erases the pixels in a rectangular area by setting them to transparent black.
// Draw a red rect
ctx.fillStyle = '#ff0000'; // Red
ctx.fillRect(10, 10, 280, 280); // x, y, width, height
// Stroke a blue rect
ctx.lineWidth = '2';
ctx.strokeStyle = '#0000ff';
ctx.strokeRect(100, 100, 100, 100);
// Erase an area
ctx.clearRect(125, 125, 50, 50);
How to draw Arcs on Canvas?
The arc method is defined as ctx.arc(x, y, radius, startAngle, endAngle [, anticlockwise]);
. It is used to create a circular arc centred at (x, y)
with a radius
.
ctx.beginPath();
ctx.arc(150, 150, 50, 0, 2 * Math.PI);
ctx.stroke();
ctx.beginPath();
ctx.arc(150, 150, 60, 0, 1 * Math.PI);
ctx.stroke();
Now, the vital thing to note here is, the arc method is only available for sub-paths, hence beginPath()
and stroke()
.
How to set Gradients in Canvas?
In the canvas world, a gradient can be seen as a variable colour that can be used as a fillStyle
or strokeStyle
.
There are two types of gradients available
createLinearGradient()
- A gradient along the line connecting two given coordinates.createRadialGradient()
- A radial gradient using the size and coordinates of two circles.
How to set Linear gradient in Canvas?
var gradient = ctx.createLinearGradient(50,50, 50,250); // Start x,y and end x,y
// Add three color stops
gradient.addColorStop(0, 'red');
gradient.addColorStop(.5, 'orange');
gradient.addColorStop(1, 'red');
// Set the fill style and draw a rectangle
ctx.fillStyle = gradient;
ctx.fillRect(50, 50, 200, 200);
How to set Radial Gradient in Canvas?
var gradient = ctx.createRadialGradient(150,150,50, 150,150,100); // Start x,y,r and end x,y,r
// Add three color stops
gradient.addColorStop(0, 'red');
gradient.addColorStop(.9, 'orange');
gradient.addColorStop(1, 'yellow');
// Set the fill style and draw a rectangle
ctx.fillStyle = gradient;
ctx.fillRect(50, 50, 200, 200);
How to write Texts on Canvas?
fillText
or strokeText
are two methods available to render texts.
var gradient = ctx.createRadialGradient(150,150,50, 150,150,100); // Start x,y,r and end x,y,r
// Add three color stops
gradient.addColorStop(0, 'red');
gradient.addColorStop(.9, 'orange');
gradient.addColorStop(1, 'yellow');
// Set the fill style and draw a rectangle
ctx.fillStyle = gradient;
// Text 1
ctx.font = 'italic 32px sans-Serif';
ctx.fillText('Hello world!', 50, 100); // String, x, y
// Text 2
ctx.font = 'bold 30px sans-Serif';
ctx.strokeText('Hello world!', 50, 200);
font
can be used to change the current font settings. The syntax is similar to as in CSS
.
What special effects Canvas support?
How to set Translation in Canvas?
The translate()
method adds a translation transformation to the current matrix. It simply means you can move the current view in (x, y)
.
Note: The coordinate system starts from top-left, rather bottom-left as usual.
// Move
ctx.translate(100, 100);
ctx.fillStyle = 'red';
ctx.fillRect(50, 50, 100, 100);
// Reset current transformation matrix to the identity matrix
ctx.setTransform(1, 0, 0, 1, 0, 0);
// Unmoved
ctx.fillStyle = 'gray';
ctx.fillRect(50, 50, 100, 100);
setTransform
is used to reset the transform matrix, to render normally. 1, 0, 0, 1, 0, 0
is the identity matrix.
How to set Rotation in Canvas?
The rotate(angle)
adds a rotation to the transformation matrix.
// Transformed
ctx.rotate(15 * Math.PI / 180);
ctx.fillStyle = 'red';
ctx.fillRect(100, 100, 100, 100);
// Reset current transformation matrix to the identity matrix
ctx.setTransform(1, 0, 0, 1, 0, 0);
// Untransformed
ctx.fillStyle = 'gray';
ctx.fillRect(100, 100, 100, 100);
How to set Scale in Canvas?
The scale(x, y)
adds a scaling transformation to the canvas horizontally and/or vertically.
// Transformed
ctx.scale(2, 1);
ctx.fillStyle = 'red';
ctx.fillRect(50, 150, 50, 50);
// Reset current transformation matrix to the identity matrix
ctx.setTransform(1, 0, 0, 1, 0, 0);
// Untransformed
ctx.fillStyle = 'gray';
ctx.fillRect(50, 50, 50, 50);
What States does Canvas have?
Canvas provides two methods to save and restore the states.
The canvas state is a snapshot of all the styles and transformations that have been applied. More details.
save()
- saves the entire state of the canvas by pushing the current state onto a stack.
restore()
- restores the most recently saved canvas state by popping the top entry in the drawing state stack.
// Save the default state
ctx.save();
ctx.fillStyle = 'green';
ctx.fillRect(50, 50, 50, 50);
// Restore the default state
ctx.restore();
ctx.fillRect(150, 150, 50, 50);
How to clip rectangles in Canvas?
The clip()
method clips a region of any shape and size from the original canvas.
// Create clipping region
ctx.beginPath();
ctx.arc(150, 150, 100, 0, Math.PI * 2);
ctx.clip();
// Draw
ctx.fillStyle = 'orange';
ctx.fillRect(0, 0, canvas.width, canvas.height);
ctx.fillStyle = 'red';
ctx.fillRect(0, 0, 150, 150);
Once a region is clipped, all future drawing will be limited to the clipped region only. States (save and restore) can be used to work out this limitation.
A clipping region is limited to primitive paths only.
fillRule parameter supports:
- nonzero
- evenodd rules,
which decides if a point is inside or outside the clipping region.