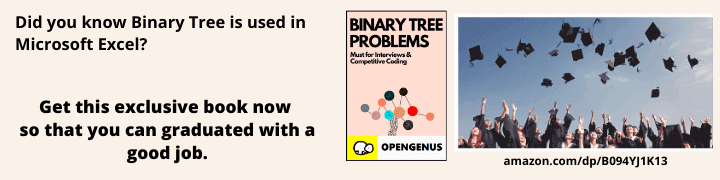
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 20 minutes | Coding time: 10 minutes
The switch statement compares the value of an expression or variable against a list of case values and when a match is found the block of statements associated with that case is executed.
Syntax for switch case:
switch(expression or variable)
{
case 1:<statement block>;
break;
case 2:<statement block>;
break;
case 3:<statement block>;
break;
case n:<statement block>;
break;
default:<statement>;
}
Here switch and case are keywords. break is a keyword which transfers the control at the end of the loop.
Note: the expression or variable in switch condition should evaluate to primitive data types like integer or character. It does not support floating point numbers. Boolean are used as it is converted to integers and strings are not used as it is not natively supported in C.
We can write cases as per our condition and when the case that matches with our condition then the block of statements related with that case is executed and if none of the case matches with our condition then the statements defined in the default case is executed.
Example with Integer case
Let us consider an example where we will print the week day upon the day number (like 2 for Tuesday).
#include <stdio.h>
int main()
{
int weeknumber;
//Reading week no from user
printf("Enter week number(1-7): ");
scanf("%d", &weeknumber);
switch(weeknumber)
{
case 1: printf("Monday");
break;
case 2: printf("Tuesday");
break;
case 3: printf("Wednesday");
break;
case 4: printf("Thursday");
break;
case 5: printf("Friday");
break;
case 6: printf("Saturday");
break;
case 7: printf("Sunday");
break;
default: printf("Invalid input! Please enter week no. between 1-7.");
}
return 0;
}
Output
Enter the week number(1-7):6
Saturday
Output(if you enter anything else than between 1-7)
Enter the week number(1-7):8
Invalid input! Please enter week no. between 1-7.
Example with character case
In this simple example, we will use character as a matching case.
#include <stdio.h>
int main()
{
char character;
printf("Enter a, b or other character\n");
scanf("%c", &character);
switch(character)
{
case 'a': printf("A");
break;
case 'b': printf("B");
break;
default: printf("other character");
}
return 0;
}
Output:
Enter a, b or other character
O
other character
What happens in absence of break statement?
If you don't put a break statement after each case then when a matching case is found, the block of statements associated with it is executed and the block of statements associated with the case below the matching case is also executed, so it is suggested to put break statement after each case.
Let us consider the example below:
#include <stdio.h>
int main()
{
int weeknumber;
//Reading week no from user
printf("Enter week number(1-7): ");
scanf("%d", &weeknumber);
switch(weeknumber)
{
case 1: printf("Monday");
case 2: printf("Tuesday");
case 3: printf("Wednesday");
case 4: printf("Thursday");
case 5: printf("Friday");
case 6: printf("Saturday");
case 7: printf("Sunday");
default: printf("Invalid input! Please enter week no. between 1-7.");
}
return 0;
}
Output
Enter the week number(1-7):3
Wednesday
Thursday
Friday
Saturday
Sunday
Invalid input! Please enter week no. between 1-7.
All the statements associated with the case below the matching case are printed. Hence it is better to use break statement so we can get the desired output.
This is known as switch case flow through.
Points to remember
So, while you use switch case, be careful and remember:
- To use only integers or characters as matching case
- Place break statement after each matching case
- Place a default case
- Missing break case results fall through and is dangerous
Keep using switch case and enjoy