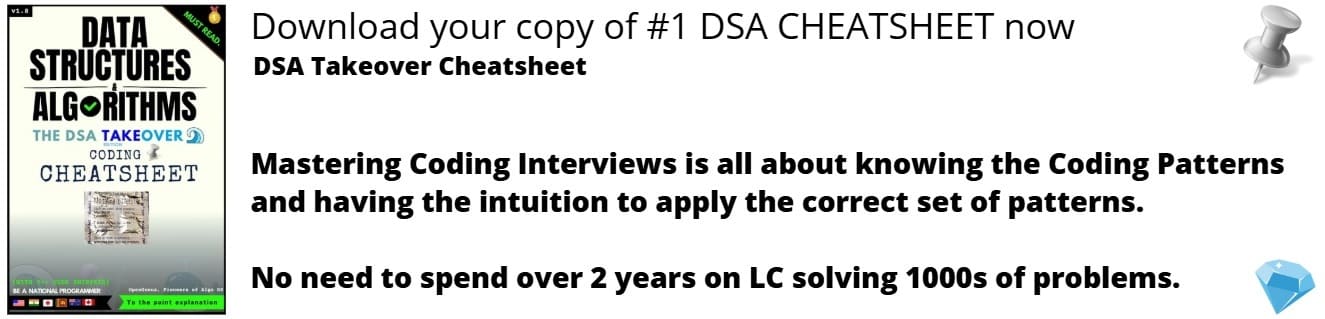
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have covered Different ways to terminate JavaScript code which includes return, break, try catch, throw, debugger and much more.
Table Content
- Introduction
- Inserting JavaScript into HTML file
- Different Ways to Terminate the Code
3.1. Return
3.2. Break
3.3. Try...catch
3.4. Throw
3.5. Debugger - Final Considerations
Prerequisite: JavaScript
1. Introduction
JavaScript is a programming language created by Brendan Eich in 1995 while he worked at Netscape. Initially, the language name was Mocha, later LiveScript, and today it is known as JavaScript.
In 1996 to standardize the newly created language, Netscape decided to join JavaScript to ECMA (European Computer Manufacturers Association). ECMA is an association that performs specifications and/or standardization of information systems.
The ECMAScript (ES) is a specification responsible for publishing JavaScript updates. And from this specification came the acronyms ES6, ES2015, and ES2020.
JavaScript is a language that allows you to implement complex items within web pages, making the content of the pages interact with the user[2].
The language presents some characteristics:
- it is the interpreted type;
- manipulated at runtime;
- the code is executed from top to bottom (order is important);
- the result of code execution is immediately returned.
2. Inserting JavaScript into HTML file
There are two possibilities for inserting JavaScript code inside an HTML file:
- internal form (Figure 1), the code is inserted directly inside the HTML structure, using the tags <script> and </script>;
<!DOCTYPE html>
<html>
<head>
<script type="text/javascript">
//JavaScript Code
</script>
</head>
<body>
</body>
</html>
Figure 1. JavaScript code inserted directly into the HTML file.
- Externally (Figure 2), the JavaScript code is kept inside a file separate from the HTML. For this, it's necessary to reference such file on the page:
<!DOCTYPE html>
<html>
<head>
</head>
<body>
<script type ="text/javascript" src="script.js"></script>
</body>
</html>
Figure 2. Referencing separate JavaScript Code files inside HTML.
Depending on javascript code location (inside the body or header tag). The processing speed also varies. When the code is inside the body tag, the loading speed is faster. The website content loads first and after the JavaScript code.
3. Different ways to terminate the code
There are three main ways to terminate JavaScript code:
3.1. Return
It is possible to exit a function with return. The output uses the return or the return generating a value.
In the function of Figure 3, a verification of the variables a and b is done. If the value of b is equal to zero, the return generates a message. Otherwise, it returns the sum value between a and b.
function sum(a,b){
if(b==0){
return "invalid value";
}
else return a+b;
}
console.log(sum(2,4));
console.log(sum(3,0));
Figure 3. Function using the return command.
The result of the function (Figure 3), we obtained the value 6 related to the sum between a and b and the message invalid value because the value informed of "b" for one of the tests was zero.
3.2. Break
Another way to terminate a Javascript function is to use the break command.
Typically, this command is ideal for exiting loops.
In Figure 4, there is a function that uses the break command. It generates a "Turn on!" message. The message, "Turn off!" will not appear because the code stops with the command break lamp.
const lamp = () => {
lamp : {
console.log("Turn on!");
break lamp ;
// The message below not generated (break command above)
console.log("Turn off!");
}
};
lamp();
Figure 4. JavaScript code using the break command.
3.3. Try...catch
Try and catch are used to exit a function using an exception. In other words, an exception is defined within the code to interrupt the normal flow.
The try block specifies a condition if there is an exception implementation.
The catch block catches this exception and exits the function leaving all other instructions unexecuted.
In the code in Figure 5, the try block specifies that the t variable is not a string, and the catch block generates the message in case of this exception.
function test() {
var t = 34;
try {
if(typeof(t)!= 'string')
throw(t +" is not a string" );
}
catch ( c ) {
console.log("Error: " + c );
}
t++;
return t;
}
test();
Figure 5. Javascript code using the try and catch command.
The result of the code in Figure 5 generates the message Error: 34 is not a string.
3.4. Throw
The throw command implements a user-defined exception, in which execution stops without executing the subsequent instructions.
In the example in Figure 6, control of program execution occurs in the catch block with the throw command. The code returns the message The element is not a number!
function ErrorMsg(i, t) {
if (typeof(i) || typeof(t)) {
throw "The element is not a number!";
}
}
try {
ErrorMsg(32, 'L');
}
catch(e) {
console.error(e);
}
Figure 6. Javascript code using the throw command.
3.5. Debugger
The debugger command stops code execution and implements (if available) the debugging function.
This command acts as a breakpoint in the debugger.
In Figure 6 (the debugger enable), the code will stop execution before executing the third line. The result the code returns is 40.
<!DOCTYPE html>
<html>
<body>
<h1>Debugger</h1>
<p id="test"></p>
<script>
let x = 20 * 2;
debugger;
window.document.getElementById("test").innerHTML = x;
</script>
</body>
</html>
Figure 7. Javascript code inside the HTML file. The use of the debugger command.
4. Final Considerations
The major browsers supported the mentioned ways to terminate the code. There are many other ways to terminate the code, but each termination command depends on the article's purpose. For best use of termination commands, it is necessary to analyze the purpose of the code under development.
With this article at OpenGenus, you must have the complete idea of Different ways to terminate JavaScript code.