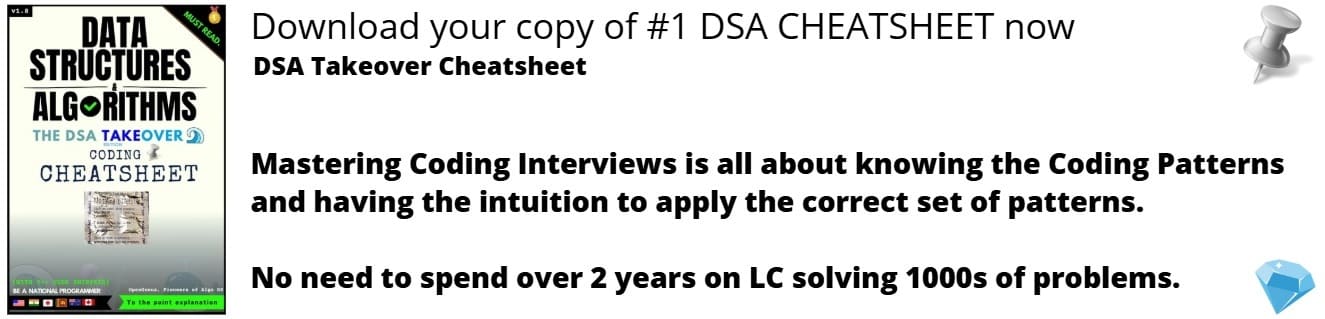
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
React.js was developed in 2011 by the Facebook team for their internal use. Later, it became open source. Due to its attractive features, React.js welcomed by the entire developer community. This article will attempt to explain the basic concepts of react.js.
What is React?
React is a javascript library used for building the user interfaces or single page apps. Complex UI components can be composed into a smaller and isolated piece of code. React is declarative, efficient, and flexible, it updates and renders the only that component where data have been changed.
All the react components are being rendered by the single root level HTML element.
React maintains the virtual DOM. Instead of, directly updating the real DOM and refreshing again and again which is time-consuming and affects the web performance.
React uses the diffing algorithm, React virtual DOM compares the previous changes with the new changes and updates only the necessary ones. After that real DOM copies the necessary update from the virtual DOM.
Basic Example In React look like
ReactDOM.render(
<h1>Learning React</h1>,
document.getElementById('root')
)
In the above snippet ReactDOM.render renders the h1 element and add it inside the 'root' id of div element which you can find in the index.html file of the react app.
Basic Concepts of React: -
- JSX
- Component
- Props
- State
- Handling Events
- List and Keys
We will start looking into each of the concepts one by one : -
JSX in React
JSX is an extension of javascript used for defining how the UI should look like. It's XML based syntax. It's not javascript code, if you run the same code in javascript your browser engine will crash. React doesn't understand the jsx instead, it is just syntactical sugar, which makes the developer's life easier to write the UI code. The code is transpile into something which the React can understand.
take below the example
const element=<div>Learning react</div>
//transpile into
const element=React.createElement('div',null,'Learning React')
We can embed the javascript variables in the JSX using the curly brackets
const a=5;
const element=<div>He will go home at {a} pm </div>
Component
A component in react is an independent and reusable piece of code. Each piece of code works in isolation and returns the react element.
Components in react are two types :
- Class Components
- Functional Components
Let see first class components :-
Class component in react in javascript class that extends the React.Component which has the render method.
Class component
import React from "react";
class ClassComponent extends React.Component {
render() {
return <h1>Learning react</h1>;
}
}
Functional Component is simple javascript function which returns JSX.
function Functional(){
return <h1>Learning react</h1>;
}
Props
At the above, we have seen simple DOM tag is used for react element.Element can also be represented as user-defined components
const element=<Student name="Devansh">
React element is represented in the JSX with attribute name. Here Student is a component. Then, we will pass the JSX attributes as the argument in the functional component
All the JSX attributes and children will be passed as arguments in the form of objects which we called as props mean properties.
Let see how this works : -
function Student(props){
return <h1>Hello , {props.name} </h1>;
In case of Class components
class Student extends React.Component{
render(){
const {name}=this.props;
return <h1>Hello ,{name}</h1>;
}
}
In case of class component ,using the object destructure we can acess the name from the props.
State
State is an object in react that exist inside the component. Whenever any state change happens the entire app will re-render.State is useful for getting or rendering the different values like for example clock timer, stopwatch, or on button click we want something to happen with an app that all can be managed using the state.
We will see the usage of state with the below examples: -
In the case of functional components, we have state hook.
Hook is a special type of function that lets you 'hook into' the react features. useState is used for adding the react state to the functional components.
import React,{useState} from 'react';
function Example() {
// Declare a new state variable, which we'll call "count"
const [count, setCount] = useState(0);
setTimeout(
() => setCount(count + 1),
1000
)
return (
<div>
<p>{count} times</p>
</div>
);
}
Let see what above code trying to do line by line : -
First of all ,importing the 'useState' hook feature.
Here the square bracket mean ,we are using the array destructring.We declare the two variables count and setCount ,count is intialized as 0 ,setCount is assigned to the function that we can use to modify the state variable 'count' values.
Using the class component
class Clock extends React.Component {
constructor(props) {
super(props);
this.state = {count:0};
}
setTimeout(
() => this.setState({
count:this.state.count+1
}),
1000
)
render() {
return (
<div>
<p>{count} times</p>
</div>
);
}
}
Handling Events
Often times we want to associate the event with our buttons or links.Handling the event in react works different than javascript .In react ,events are named as camelCase and using JSX,we pass a function as the event handler.
Let's take an above example of 'state' code snippet.
We will make a button .On clicking the button the count value increment will be displayed .
import React,{useState} from 'react';
function Example() {
// Declare a new state variable, which we'll call "count"
const [count, setCount] = useState(0);
const handleClick=()=>{
console.log('clicked')
}
return (
<div>
<button onClick={handleClick}>
Click me
</button>
</div>
);
}
Above , we have set above the button attributes onClick(mouse event
)reference to the handleClick
function.Every time , button is clicked handleClick
function will be called and log the message clicked
.We will use this function calling and click event for incrementing the state of count. Let see how ..
import React,{useState} from 'react';
function Example(){
const [count,setCount]=useState(0);
const handleClick=()=>{
setCount(count+1)
console.log(count)
}
return (
<div>
<p>{count} times</p>
<button onClick={handleClick}>
Click me
</button>
</div>
);
}
If we now see the browser console , we will see the increment in the value of count ,whenever the button is clicked.
List and Keys
For rendering the list of items ,we use the map
function from the javascript.Let's take an example
const students=['john','david','alice','bob','smith']
we can loop through the 'students' array using the map function.
const studentlist=students.map((student)=>
<li>{student}</li>
);
render this into the DOM
ReactDOM.render(
<ul>{studentlist}</ul>,
document.getElementById('root')
)
Let see the same example with the StudentList Component
function StudentList(props) {
const students = props.students;
const studentlist = students.map((student) =>
<li>{student}</li>
);
return (
<ul>{studentlist}</ul>
);
}
const students=['john','david','alice','bob','smith']
ReactDOM.render(
<StudentList students={students} />,
document.getElementById('root')
);
It displays the list of student on browser screen
- john
- david
- alice
- bob
- smith
But, it also throws a warning if you see in the browser console -
“Warning: Each child in an array or iterator should have a unique ‘key’ prop.%s%s See https://fb.me/react-warning-keys for more information.%s”.Key helps react uniquely identify which items have changed ,are added or removed.Key gives the unique identity to each element of arrays.
We will take same example : -
function StudentList(props) {
const students=props.students;
const studentlist = students.map((student) =>
<li key={student.id}>
{student.value}
</li>
);
return (
<ul>{studentlist}</ul>
);
}
const student = [{id: 'a', value: 'john'},
{id: 'b', value: 'david'},
{id: 'c', value: 'alice'},
{id: 'd', value: 'bob'},
{id: 'e', value: 'smith'}];
ReactDOM.render(
<ListComponent students={students} />,
document.getElementById('root')
);
The above code produce the same result but we will not get any warning in the browser console.
So far we have read about components, state, props, event handling, and key, props in react. These are basics or fundamentals of react but this is not the end, there are a whole lot more things in react.