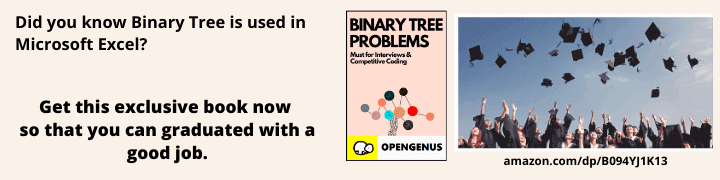
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explained the approach to get IP addresses using JavaScript.
Table of Contents
- Getting IP addresses using an external API
- Setting up your own IP address service
- Why is there no client side method
Getting IP addresses using an external API
When you make a request to a website, the request includes your IP address. Such requests are made whenever you visit a website, or use APIs such as fetch, or XMLHttpRequest. There are services out there made to send this IP address back to you after reading it. Thus, these websites allow you to find your IP address. You send an HTTP request to one of these services, and just read your IP address from the response. ipify.org, ipinfo.io are some such services. A more comprehensive list can be found on Stack Overflow incase the services listed above do not work when you are reading this article at OpenGenus.
Let's use the fetch API to make a request to one of the above services, and log the IP address to the console.
fetch('https://api.ipify.org/').then(
r => r.text()
).then(console.log);
// -> 144.22.31.81
// (The output will vary for everyone)
Ipify returns the response in the form of text. On receiving the response, we extract the text from the contents of the response, following which we log it to the console.
Setting up your own IP address service
Instead of relying on a third party service such as ipify to read your IP and send it back to you, you can create your own. Creating your own IP address service isn't hard and can be done in just a few lines of code. We are going to set up a server using node.js. Type the code written below into a file.
const http = require('http')
http.createServer((req, res)=>{
res.writeHead(200, {'Content-Type' : 'text/plain'});
res.end(req.socket.remoteAddress);
}).listen(3000);
Now, run the code by opening a terminal in the same folder and issuing the command node <filename>
. This will start a server on localhost:3000 which we can make requests to.
In the code above, we have imported the http module, and used its createServer
method to create a server which is listening on port 3000. Inside createServer
, we pass a method which is executed for every incoming request. The method simply writes a 200 status response, which includes the plaintext remote IP address. Let's now make a request to localhost:3000
, similar to how you made a request to ipify.org
. Run this javascript on the client-side in the usual way :
fetch('http://localhost:3000').then(
r => r.text()
).then(console.log);
You will probably see the address ::1
on the console, which is the IPv6 loopback address, or 127.0.0.1
- the IPv4 loopback address. It means that the machine is making a request to itself. If this service was on a different server, then the IP address visible to that server would have been returned. This IP address varies based on the details of your connection, whether you are using a VPN or a proxy etc.
Why is there no client side method ?
You might be wondering why there is no straightforward way of getting the IP on the client itself. A simple getIP()
method would make the process so simple, trivial even. One reason is that IP addresses only make sense when computers are connected in a network. When you run a local server on your computer, you'll simply get the loopback address ::1
or 127.0.0.1
. It is when you are connected to the internet, and ask a computer what your IP is, that you are able to learn of your IP address (or at least of what IP the other computer is able to see).
Still, you might complain that you have an IP address assigned to you when you are connected to the internet, and perhaps there could be a variable which shows the value of this assigned IP address. But such variable is not provided in Javascript either. Distinction between public and private IP addresses might be the reason for this. Some computers have both public and private addresses assigned to them, and private addresses are meant to be just that - private. Imagine you are using a proxy. Your computer has a private IP address, which no one knows of, and it has a public IP address visible to everyone else - the address of the proxy. If there was a method such as getIP()
that would execute on the client, the proxy is not able to hide the address of the computer anymore - because the getIP()
function is executing directly on the computer itself revealing it's private IP. Can you think of other reasons there isn't a client-side method to get the IP of a computer ? Or can you think of secure ways to make this possible on a computer ?
Wrapping up
The standard way to find the IP address of a computer is to make requests to a server. This server could be your own, or it could be someone else's server like in case of third party services. Regardless, all methods to find your IP require you to make remote requests and a client side method is not available at the time of writing.