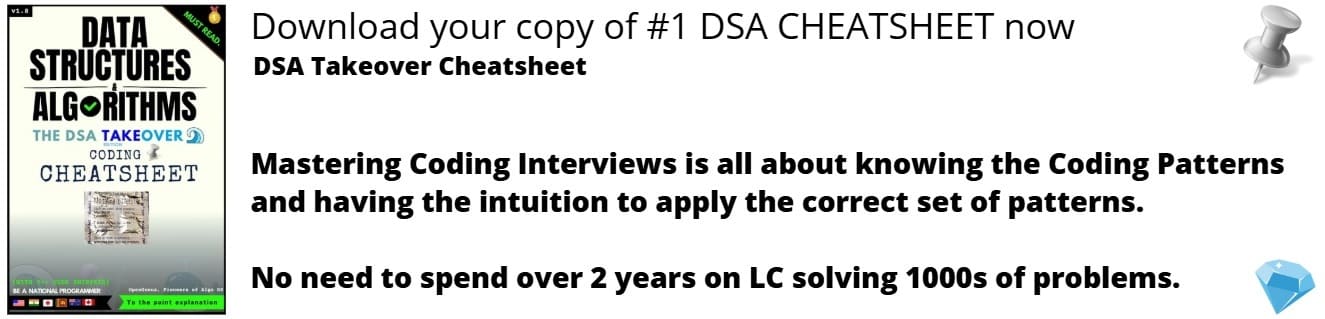
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Reading time: 20 minutes | Coding time: 5 minutes
#if is a preprocessor directive in C to define conditional compilation. It can be used just like an if condition statement which impacts the compilation process and the executable that is created.
Note that everything in this is applicable for C++ as well.
Syntax:
#if <condition>
<code statements>
#endif
if directive is associated with else directive as well like:
#if <condition>
<code statements>
#else
<code statements>
#endif
We have multiple else parts as well like:
#if <condition>
<code statements>
#elif
<code statements>
#else
<code statements>
#endif
Simple example of #if
In this simple C code example, we demonstrate a simple use of if directive. We defined a MACRO named OPENGENUS with value 1 and in the code, we added a if directive to check if the MACRO OPENGENUS is defined and if it is defined, a printf statement is provided which prints the value.
#include <stdio.h>
#define OPENGENUS 1
int main()
{
#if defined OPENGENUS
printf("%d", OPENGENUS);
#endif
return 0;
}
Output:
1
If we do not define the MACRO OPENGENUS, we will get no output. Consider the following C code:
#include <stdio.h>
int main()
{
#if defined OPENGENUS
printf("%d", OPENGENUS);
#endif
return 0;
}
The above code will give no output.
Simple example of #if with else part
In this simple C code, we demonstrate the use of if directive with an else part. We have defined a MACRO named OPENGENUS and check that if it is defined, we will print Yes and if it is not defined, we will print No.
#include <stdio.h>
#define OPENGENUS 1
int main()
{
#if defined OPENGENUS
printf("Yes");
#else
printf("No");
#endif
return 0;
}
Output:
Yes
If we do not define the MACRO OPENGENUS, it will print No. Consider the following C code:
#include <stdio.h>
int main()
{
#if defined OPENGENUS
printf("Yes");
#else
printf("No");
#endif
return 0;
}
Output:
No
Simple example of #if with multiple else part
In the C code example, we demonstrate if directive with multiple if else parts. We define a MACRO OPENGENUS and check if it is even or odd and print the value accordingly.
#include <stdio.h>
#define OPENGENUS 4
int main()
{
#if OPENGENUS%2 == 0
printf("even");
#elif OPENGENUS%2 == 1
printf("odd");
#else
printf("none");
#endif
return 0;
}
Output:
even
Conditions with if directive
Note that only conditions that can be evaluated in compile time must be used. For example, we cannot place variables at compile time as the values within variables are not resolved at compile time.
The main objective of the conditions is to check the defined MACROs and certain conditions on them.
All conditions involving variables or other expressions that cannot be resolved in compile time will evaluate to false. Consider this example:
#include <stdio.h>
#define OPENGENUS 4
int main()
{
int i = 1;
#if i == 1
printf("opengenus");
#endif
return 0;
}
It will give no output as the expression i==1 evaluates to false even though i is 1. If we add an else part, the idea will be clear. Consider this example:
#include <stdio.h>
#define OPENGENUS 4
int main()
{
int i = 1;
#if i == 1
printf("opengenus 1");
#else
printf("opengenus 2");
#endif
return 0;
}
Output:
opengenus 2
Variants of if directive
Two common variants of if directive are:
- ifndef
- ifdef
ifndef means if not defined and is same as:
#if !defined MACRO
Alternative using ifndef is:
#ifndef MACRO
ifdef means if defined and is same as:
#if defined MACRO
Alternative using ifdef is:
#ifdef MACRO
As an advice, when you can use ifdef or ifndef instead of if, use it as it has direct compiler support and allows better performance. Hence, in terms of performance:
ifdef (best) > ifndef > if
Why we need if directive?
We need if directive as this allows us to do compile time conditioning which enables programmers to generate custom code during the compilation process based on various factors like:
- availablility of library files
- platform
- user defined requirements during compile time
and much more. In short, this enables a single code base to be globally acceptable.
With this, you have complete knowledge of working with if directive condition in C.