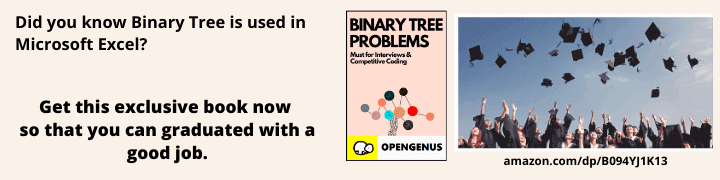
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
Next.js has become a popular choice among web developers for building production-ready applications. This versatile framework combines the power of React with server-side rendering (SSR) capabilities, making it an excellent option for modern web development. In this article at OpenGenus, we will explore the various aspects of Next.js as a production-ready framework.
Next.js provides a well-documented and easy-to-follow guide for developers. The official documentation covers all the essential topics, from getting started with a basic setup to advanced features such as data fetching, routing, and deployment. The text is clear and concise, making it easier for both beginners and experienced developers to understand and utilize the framework effectively.
Popularity of Next.js
- Next.js is used by the biggest companies like Netflix, Starbucks, Nike, Hulu, TikTok, and more.
- It is considered one of the fastest-growing React frameworks.
- It also witnessed a 50% usage growth from October 202 to June 2021
What are the advantages?
Performance: With server-side rendering, Next.js delivers fast initial page loads by generating the HTML content on the server and sending it to the client. This approach significantly reduces the time users spend waiting for content to load.
SEO-friendly: Search engines prefer websites with server-rendered content. Next.js's SSR and SSG capabilities make it a perfect choice for applications that require optimal search engine visibility.
Automatic Code Splitting: Next.js automatically splits your code into smaller, more manageable chunks. This ensures that users only download the code required for the specific page they are visiting, leading to faster loading times and improved performance.
Easy Routing: Next.js simplifies client-side navigation with its built-in routing system. Developers can use simple folder structures to define routes, making it intuitive and straightforward to create complex applications.
Vibrant Community: Next.js has a thriving community of developers who actively contribute to its growth. This means continuous updates, improvements, and a vast repository of packages and resources.
Code: Next.js Simplified
Next.js is built upon React, and the code structure is quite similar. Developers familiar with React can quickly grasp the fundamentals of Next.js. The framework offers a wide range of features and modules to enhance development productivity. Let's look at an example of how easy it is to create a simple Next.js component:
import React from 'react';
const HomePage = () => {
return (
<div>
<h1>Welcome to My Next.js App!</h1>
<p>Next.js makes server-side rendering a breeze.</p>
</div>
);
};
export default HomePage;
Backend Integration
Next.js not only excels on the frontend but also seamlessly integrates with backend functionality. We'll explore how you can implement backend code in Next.js, allowing you to build full-stack applications with ease.
Incorporating backend features enhances the capabilities of your Next.js applications, opening up a whole new world of possibilities for dynamic data handling, user authentication, and more.
Let's dive into some examples of integrating backend code in Next.js:
import { MongoClient } from "mongodb";
export default async function handler(req, res) {
const uri = `mongodb+srv://${process.env.MON_DB_USER}:${process.env.MON_DB_PASS}@${process.env.MON_DB_CLUSTER}.uo9kuvq.mongodb.net/?retryWrites=true&w=majority`;
let client;
try {
client = new MongoClient(uri);
} catch (error) {
res.status(500).json({ message: "connect to database is failed!" });
}
if (req.method === "POST") {
const { email, name, message } = req.body;
// Do validation
// store in database
const newMessage = {
email,
name,
message,
};
const db = client.db(dbName);
const collection = db.collection(collName);
try {
const results = await collection.insertOne(newMessage);
newMessage.id = results._id;
} catch (error) {
client.close();
res.status(500).json({ message: "Faild to send your message!" });
return;
}
res
.status(201)
.json({ message: "Successfuly submited!", newMessage: newMessage });
client.close();
}
}
In this Example, the provided code is an async function that handles POST requests by connecting to a MongoDB database and inserting submitted messages. It requires validation implementation for input data and improved error handling for database connections to enhance its reliability.
With backend integration in Next.js, you can build more complex and feature-rich applications without the need for a separate backend framework.
Quick Intro
Applications: Unlocking Next.js Potential
- E-commerce Platforms: Next.js enables fast-loading product pages and enhances SEO, providing a solid foundation for e-commerce websites.
- Blogs and Content Websites: With SSG capabilities, Next.js can generate static pages, reducing the server load and improving overall user experience.
- Enterprise Applications: Next.js can be used to build complex and scalable enterprise-level applications that demand high performance and maintainability.
Next.js 13: What's New?
Next.js 13 is a major release of the most popular React framework: in face, it ships with a new routing system, aslo called App Router. In many ways, this new routing system is complete rewrite of the previous one, and fundamentally changes the way we write Next.js applications.
Server Components (RSC)
The biggest change in the new App Router is the introduction of Server Components, a new type of React components that run on the server and return compiled JSX that is sent to the client.
Advantage:
- Load faster - don't have to wait for the javascript to load
- smaller client bundle size
- SEO friendly
- Access to resource the client can't access
- More secure against XSS attacks
Disadvantages
- Not as interactive
- No component state(we can not use the useState hook)
- No component lifecycle methods(we can not use the useEffect hook)
Layouts
Powered by Server Components, layouts are foundational components that wrap pages. Next.js Layouts are not only useful for displaying a common UI across pages, but also to reuse logic and data fetching across pages.
Enhanced Router
Using conventional filenames, we can add various types of components in the app directory. This is a big improvement over the current routing system, which requires us to use a specific directory structure to define pages, API handlers, and so on.
What does this mean? From now on, we can create various types of components in the app directory using a specific filename convention specific to Next.js:
- Pages are defined as page.tsx
- layouts are defined as layout.tsx
- errors are defined as error.tsx
- loading states are defined as loading.tsx
File structure
What does the new Next.js 13 file structure look like? Below is an example of a Next.js 13 app with the new app directory:
Wrapping Up
Next.js has emerged as a game-changing framework for building production-ready web applications. Its blend of server-side rendering, static site generation, and other features like built-in CSS support and TypeScript integration simplifies web development while ensuring exceptional performance and scalability.
Whether you are a seasoned developer or just starting your journey, Next.js offers an excellent platform for crafting modern web experiences that will delight your users. Embrace the power of Next.js to take your web development projects to the next level, and stay ahead in this ever-evolving digital landscape. Happy coding!